Bela
|
Bela.h
Go to the documentation of this file.
103 #define BELA_FLAG_ANALOG_OUTPUTS_PERSIST (1 << 1) // Set if analog/digital outputs persist for future buffers
605 AuxiliaryTask Bela_createAuxiliaryTask(void (*functionToCall)(void*), int priority, const char *name, void* args, bool autoSchedule = false);
606 AuxiliaryTask Bela_createAuxiliaryTask(void (*functionToCall)(void), int priority, const char *name, bool autoSchedule = false);
int Bela_getopt_long(int argc, char *argv[], const char *customShortOptions, const struct option *customLongOptions, BelaInitSettings *settings)
Get long options from command line argument list, including Bela standard options.
const float *const analogIn
Buffer holding analog input samples.
Definition: Bela.h:210
int ampMutePin
Pin where amplifier mute can be found.
Definition: Bela.h:173
const uint32_t audioInChannels
Number of input audio channels.
Definition: Bela.h:227
const float digitalSampleRate
Digital sample rate in Hz (currently always 44100.0)
Definition: Bela.h:263
const uint32_t analogInChannels
Number of input analog channels.
Definition: Bela.h:241
int receivePort
Port where the UDP server will listen.
Definition: Bela.h:175
float headphoneLevel
Level for the headphone output.
Definition: Bela.h:146
const uint32_t analogOutChannels
Number of output analog channels.
Definition: Bela.h:246
int Bela_startAuxiliaryTask(AuxiliaryTask it)
Stop processing audio and sensor data.
int transmitPort
Port where the UDP client will transmit.
Definition: Bela.h:177
AuxiliaryTask Bela_createAuxiliaryTask(void(*functionToCall)(void *), int priority, const char *name, void *args, bool autoSchedule=false)
Create a new auxiliary task.
const uint32_t digitalChannels
Number of digital channels.
Definition: Bela.h:261
const uint32_t digitalFrames
Number of digital frames per period.
Definition: Bela.h:257
int numAudioInChannels
How many audio input channels.
Definition: Bela.h:127
int Bela_setHeadphoneLevel(float decibels)
Set the level of the onboard headphone amplifier.
void Bela_scheduleAuxiliaryTask(AuxiliaryTask task)
Run an auxiliary task which has previously been created.
int pruNumber
Which PRU (0 or 1) the code should run on.
Definition: Bela.h:151
void cleanup(BelaContext *context, void *userData)
User-defined cleanup function which runs when the program finishes.
Definition: 01-Basics/minimal/render.cpp:51
Structure containing initialisation parameters for the real-time audio control system.
Definition: Bela.h:114
float *const audioOut
Buffer holding audio output samples.
Definition: Bela.h:203
int numAudioOutChannels
How many audio out channels.
Definition: Bela.h:129
int interleave
Whether audio/analog data should be interleaved.
Definition: Bela.h:161
const uint32_t audioFrames
Number of audio frames per period.
Definition: Bela.h:225
const float analogSampleRate
Analog sample rate in Hz.
Definition: Bela.h:254
Structure holding current audio and sensor settings and pointers to data buffers. ...
Definition: Bela.h:190
bool setup(BelaContext *context, void *userData)
User-defined initialisation function which runs before audio rendering begins.
Definition: 01-Basics/minimal/render.cpp:35
int numMuxChannels
How many channels to use on the multiplexer capelet, if enabled.
Definition: Bela.h:148
int Bela_initAudio(BelaInitSettings *settings, void *userData)
Initialise audio and sensor rendering environment.
int numAnalogOutChannels
How many analog output channels.
Definition: Bela.h:133
float *const analogOut
Buffer holding analog output samples.
Definition: Bela.h:217
const uint32_t audioOutChannels
Number of output audio channels.
Definition: Bela.h:229
int Bela_setPgaGain(float decibels, int channel)
Set the gain of the audio preamplifier.
int beginMuted
Whether to begin with the speakers muted.
Definition: Bela.h:138
int numAnalogInChannels
How many analog input channels.
Definition: Bela.h:131
int gShouldStop
Wiring-inspired utility functions and macros.
void render(BelaContext *context, void *userData)
User-defined callback function to process audio and sensor data.
Definition: 01-Basics/minimal/render.cpp:44
uint32_t *const digital
Buffer holding digital input/output samples.
Definition: Bela.h:222
const uint64_t audioFramesElapsed
Number of elapsed audio frames since the start of rendering.
Definition: Bela.h:271
int codecI2CAddress
Where the codec can be found on the I2C bus.
Definition: Bela.h:171
const uint32_t analogFrames
Number of analog frames per period.
Definition: Bela.h:236
int numDigitalChannels
How many channels for the GPIOs.
Definition: Bela.h:135
int useAnalog
Whether to use the analog input and output.
Definition: Bela.h:123
const float audioSampleRate
Audio sample rate in Hz (currently always 44100.0)
Definition: Bela.h:231
int periodSize
Number of (analog) frames per period.
Definition: Bela.h:121
int analogOutputsPersist
Whether analog outputs should persist to future frames.
Definition: Bela.h:165
const float *const audioIn
Buffer holding audio input samples.
Definition: Bela.h:196
int useDigital
Whether to use the 16 programmable GPIOs.
Definition: Bela.h:125
void Bela_defaultSettings(BelaInitSettings *settings)
Initialise the data structure containing settings for Bela.
Generated on Thu May 8 2025 06:28:04 for Bela by
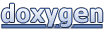