Bela
|
Utilities.h
Go to the documentation of this file.
337 // Sets a given analog output channel to a value for the current frame and, if persistent outputs are
364 // Sets a given digital output channel to a value for the current frame and all subsequent frames
const float *const analogIn
Buffer holding analog input samples.
Definition: Bela.h:210
const uint32_t audioInChannels
Number of input audio channels.
Definition: Bela.h:227
const uint32_t analogInChannels
Number of input analog channels.
Definition: Bela.h:241
static float analogRead(BelaContext *context, int frame, int channel)
Read an analog input, specifying the frame number (when to read) and the channel. ...
Definition: Utilities.h:331
static void audioWrite(BelaContext *context, int frame, int channel, float value)
Write an audio output, specifying the frame number (when to write) and the channel.
Definition: Utilities.h:324
static float constrain(float x, float min_val, float max_val)
Constrain a number to stay within a given range.
Definition: Utilities.h:424
const uint32_t analogOutChannels
Number of output analog channels.
Definition: Bela.h:246
const uint32_t digitalFrames
Number of digital frames per period.
Definition: Bela.h:257
static float map(float x, float in_min, float in_max, float out_min, float out_max)
Linearly rescale a number from one range of values to another.
Definition: Utilities.h:414
static int digitalRead(BelaContext *context, int frame, int channel)
Read a digital input, specifying the frame number (when to read) and the pin.
Definition: Utilities.h:358
float *const audioOut
Buffer holding audio output samples.
Definition: Bela.h:203
static void digitalWriteOnce(BelaContext *context, int frame, int channel, int value)
Write a digital output, specifying the frame number (when to write) and the pin.
Definition: Utilities.h:377
Structure holding current audio and sensor settings and pointers to data buffers. ...
Definition: Bela.h:190
static float min(float x, float y)
Returns the maximum of two numbers.
Definition: Utilities.h:435
float *const analogOut
Buffer holding analog output samples.
Definition: Bela.h:217
const uint32_t audioOutChannels
Number of output audio channels.
Definition: Bela.h:229
static void analogWriteOnce(BelaContext *context, int frame, int channel, float value)
Write an analog output, specifying the frame number (when to write) and the channel.
Definition: Utilities.h:351
uint32_t *const digital
Buffer holding digital input/output samples.
Definition: Bela.h:222
static void analogWrite(BelaContext *context, int frame, int channel, float value)
Write an analog output, specifying the frame number (when to write) and the channel.
Definition: Utilities.h:339
static float max(float x, float y)
Returns the minimum of two numbers.
Definition: Utilities.h:431
Main Bela public API.
static float audioRead(BelaContext *context, int frame, int channel)
Read an audio input, specifying the frame number (when to read) and the channel.
Definition: Utilities.h:317
const uint32_t analogFrames
Number of analog frames per period.
Definition: Bela.h:236
static void pinMode(BelaContext *context, int frame, int channel, int mode)
Set the direction of a digital pin to input or output.
Definition: Utilities.h:387
static void pinModeOnce(BelaContext *context, int frame, int channel, int mode)
Set the direction of a digital pin to input or output.
Definition: Utilities.h:399
static void digitalWrite(BelaContext *context, int frame, int channel, int value)
Write a digital output, specifying the frame number (when to write) and the pin.
Definition: Utilities.h:365
const float *const audioIn
Buffer holding audio input samples.
Definition: Bela.h:196
Generated on Fri Aug 1 2025 06:28:13 for Bela by
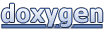