Bela
|
Macros | |
#define | INPUT 0x0 |
#define | OUTPUT 0x1 |
static float | audioRead (BelaContext *context, int frame, int channel) |
Read an audio input, specifying the frame number (when to read) and the channel. More... | |
static void | audioWrite (BelaContext *context, int frame, int channel, float value) |
Write an audio output, specifying the frame number (when to write) and the channel. More... | |
static float | analogRead (BelaContext *context, int frame, int channel) |
Read an analog input, specifying the frame number (when to read) and the channel. More... | |
static void | analogWrite (BelaContext *context, int frame, int channel, float value) |
Write an analog output, specifying the frame number (when to write) and the channel. More... | |
static void | analogWriteOnce (BelaContext *context, int frame, int channel, float value) |
Write an analog output, specifying the frame number (when to write) and the channel. More... | |
static int | digitalRead (BelaContext *context, int frame, int channel) |
Read a digital input, specifying the frame number (when to read) and the pin. More... | |
static void | digitalWrite (BelaContext *context, int frame, int channel, int value) |
Write a digital output, specifying the frame number (when to write) and the pin. More... | |
static void | digitalWriteOnce (BelaContext *context, int frame, int channel, int value) |
Write a digital output, specifying the frame number (when to write) and the pin. More... | |
static void | pinMode (BelaContext *context, int frame, int channel, int mode) |
Set the direction of a digital pin to input or output. More... | |
static void | pinModeOnce (BelaContext *context, int frame, int channel, int mode) |
Set the direction of a digital pin to input or output. More... | |
Detailed Description
These functions and macros are used for audio, analog and digital I/O. All the I/O functions require the BelaContext data structure from render() to be passed in. This means that these functions are, by design, only usable from within the rendering thread.
The naming conventions are loosely derived from the Arduino environment, and the syntax is similar. Unlike Arduino, the I/O functions require the frame number at which the read or write should take place, since all I/O happens synchronously with the audio clock.
Function Documentation
|
inlinestatic |
Read an audio input, specifying the frame number (when to read) and the channel.
This function returns the value of an audio input, at the time indicated by frame
. The returned value ranges from -1 to 1.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to read the audio input. Valid values range from 0 to (context->audioFrames - 1). channel Which audio input to read. Valid values are between 0 and (context->audioChannels - 1), typically 0 to 1 by default.
- Returns
- Value of the analog input, range to 1.
- Examples:
- FFT-phase-vocoder/render.cpp, passthrough/render.cpp, and tremolo/render.cpp.
|
inlinestatic |
Write an audio output, specifying the frame number (when to write) and the channel.
This function sets the value of an audio output, at the time indicated by frame
. Valid values are between -1 and 1.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to write the audio output. Valid values range from 0 to (context->audioFrames - 1). channel Which analog output to write. Valid values are between 0 and (context->audioChannels - 1), typically 0 to 1 by default. value Value to write to the output, range -1 to 1.
|
inlinestatic |
Read an analog input, specifying the frame number (when to read) and the channel.
This function returns the value of an analog input, at the time indicated by frame
. The returned value ranges from 0 to 1, corresponding to a voltage range of 0 to 4.096V.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to read the analog input. Valid values range from 0 to (context->analogFrames - 1). channel Which analog input to read. Valid values are between 0 and (context->analogChannels - 1), typically 0 to 7 by default.
- Returns
- Value of the analog input, range 0 to 1.
|
inlinestatic |
Write an analog output, specifying the frame number (when to write) and the channel.
This function sets the value of an analog output, at the time indicated by frame
. Valid values are between 0 and 1, corresponding to the range 0 to 5V.
The value written will persist for all future frames if BELA_FLAG_ANALOG_OUTPUTS_PERSIST is set in context->flags. This is the default behaviour.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to write the analog output. Valid values range from 0 to (context->analogFrames - 1). channel Which analog output to write. Valid values are between 0 and (context->analogChannels - 1), typically 0 to 7 by default. value Value to write to the output, range 0 to 1.
- Examples:
- analog-output/render.cpp, and passthrough/render.cpp.
|
inlinestatic |
Write an analog output, specifying the frame number (when to write) and the channel.
This function sets the value of an analog output, at the time indicated by frame
. Valid values are between 0 and 1, corresponding to the range 0 to 5V.
Unlike analogWrite(), the value written will affect only the frame specified, with future values unchanged. This is faster than analogWrite() so is better suited to applications where every frame will be written to a different value. If BELA_FLAG_ANALOG_OUTPUTS_PERSIST is not set within context->flags, then analogWriteOnce() and analogWrite() are equivalent.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to write the analog output. Valid values range from 0 to (context->analogFrames - 1). channel Which analog output to write. Valid values are between 0 and (context->analogChannels - 1), typically 0 to 7 by default. value Value to write to the output, range 0 to 1.
|
inlinestatic |
Read a digital input, specifying the frame number (when to read) and the pin.
This function returns the value of a digital input, at the time indicated by frame
. The value is 0 if the pin is low, and nonzero if the pin is high (3.3V).
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to read the digital input. Valid values range from 0 to (context->digitalFrames - 1). channel Which digital pin to read. 16 pins across the P8 and P9 headers of the BeagleBone Black are available. See the constants P8_xx and P9_xx defined in digital_gpio_mapping.h.
- Returns
- Value of the digital input.
- Examples:
- digital-input/render.cpp.
|
inlinestatic |
Write a digital output, specifying the frame number (when to write) and the pin.
This function sets the value of a digital output, at the time indicated by frame
. A value of 0 sets the pin low; any other value sets the pin high (3.3V).
The value written will persist for all future frames.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to write the digital output. Valid values range from 0 to (context->digitalFrames - 1). channel Which digital output to write. 16 pins across the P8 and P9 headers of the BeagleBone Black are available. See the constants P8_xx and P9_xx defined in digital_gpio_mapping.h. value Value to write to the output.
- Examples:
- digital-output/render.cpp.
|
inlinestatic |
Write a digital output, specifying the frame number (when to write) and the pin.
This function sets the value of a digital output, at the time indicated by frame
. A value of 0 sets the pin low; any other value sets the pin high (3.3V).
Unlike digitalWrite(), the value written will affect only the frame specified, with future values unchanged. This is faster than digitalWrite() so is better suited to applications where every frame will be written to a different value.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to write the digital output. Valid values range from 0 to (context->digitalFrames - 1). channel Which digital output to write. 16 pins across the P8 and P9 headers of the BeagleBone Black are available. See the constants P8_xx and P9_xx defined in digital_gpio_mapping.h. value Value to write to the output.
- Examples:
- digital-input/render.cpp.
|
inlinestatic |
Set the direction of a digital pin to input or output.
This function sets the direction of a digital pin, at the time indicated by frame
. Valid values are INPUT
and OUTPUT
. All pins begin as inputs by default.
The value written will persist for all future frames.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to set the pin direction. Valid values range from 0 to (context->digitalFrames - 1). channel Which digital output to write. 16 pins across the P8 and P9 headers of the BeagleBone Black are available. See the constants P8_xx and P9_xx defined in digital_gpio_mapping.h. value Direction of the pin ( INPUT
orOUTPUT
).
- Examples:
- digital-input/render.cpp, and digital-output/render.cpp.
|
inlinestatic |
Set the direction of a digital pin to input or output.
This function sets the direction of a digital pin, at the time indicated by frame
. Valid values are INPUT
and OUTPUT
. All pins begin as inputs by default.
The value written will affect only the specified frame.
- Parameters
-
context The I/O data structure which is passed by Bela to render(). frame Which frame (i.e. what time) to set the pin direction. Valid values range from 0 to (context->digitalFrames - 1). channel Which digital output to write. 16 pins across the P8 and P9 headers of the BeagleBone Black are available. See the constants P8_xx and P9_xx defined in digital_gpio_mapping.h. value Direction of the pin ( INPUT
orOUTPUT
).
Generated on Fri Nov 22 2024 06:27:57 for Bela by
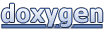