Bela
|
Scoping sensor input
This example reads from analogue inputs 0 and 1 via analogRead()
and generates a sine wave with amplitude and frequency determined by their values. It's best to connect a 10K potentiometer to each of these analog inputs. Far left and far right pins of the pot go to 3.3V and GND, the middle should be connected to the analog in pins.
The sine wave is then plotted on the oscilloscope. Click the Open Scope button to view the results. As you turn the potentiometers you will see the amplitude and frequency of the sine wave change. You can also see the two sensor readings plotted on the oscilloscope.
The scope is initialised in setup()
where the number of channels and sampling rate are set.
We can then pass signals to the scope in render()
using:
This project also shows as example of map()
which allows you to re-scale a number from one range to another. Note that map()
does not constrain your variable within the upper and lower limits. If you want to do this use the constrain()
function.
Generated on Sat Jul 12 2025 06:27:58 for Bela by
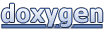