Bela
|
digital_gpio_mapping.h
8 //which might lead to unexpected results in case someone uses those in place of these or viceversa
14 //if you want to use different pins/ordering, define here new pins. The ordering here is NOT binding
33 //used in the declaration of short int digitalPins[NUM_DIGITALS] below, which is used in PRU::prepareGPIO to export the pins
35 //The ordering here is NOT binding, but if you want to use a different ordering, please change it here as well as below and in the PRU, for consistency
53 //mapping bits in the digital word to pin headers, so that pin header name can be used instead of but number
Generated on Fri Jun 6 2025 06:28:01 for Bela by
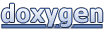