svgui
1.9
|
#include <LevelPanToolButton.h>
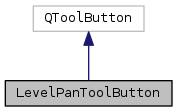
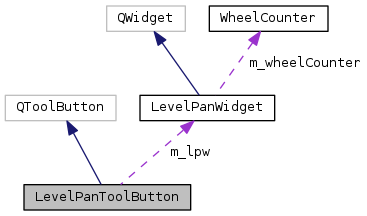
Public Slots | |
void | setLevel (float) |
Set level in the range [0,1] – will be rounded. More... | |
void | setPan (float) |
Set pan in the range [-1,1] – will be rounded. More... | |
void | setMonitoringLevels (float, float) |
Set left and right peak monitoring levels in the range [0,1]. More... | |
void | setIncludeMute (bool) |
Specify whether the level range should include muting or not. More... | |
void | setEnabled (bool enabled) |
Signals | |
void | levelChanged (float) |
void | panChanged (float) |
void | mouseEntered () |
void | mouseLeft () |
Public Member Functions | |
LevelPanToolButton (QWidget *parent=0) | |
~LevelPanToolButton () | |
float | getLevel () const |
Return level as a gain value in the range [0,1]. More... | |
float | getPan () const |
Return pan as a value in the range [-1,1]. More... | |
bool | includesMute () const |
Discover whether the level range includes muting or not. More... | |
void | setImageSize (int pixels) |
void | setProvideContextMenu (bool) |
Specify whether a right-click context menu is provided. More... | |
void | setBigImageSize (int pixels) |
Protected Slots | |
void | contextMenuRequested (const QPoint &) |
Protected Member Functions | |
void | paintEvent (QPaintEvent *) override |
void | enterEvent (QEvent *) override |
void | leaveEvent (QEvent *) override |
void | mousePressEvent (QMouseEvent *) override |
void | wheelEvent (QWheelEvent *e) override |
Protected Attributes | |
LevelPanWidget * | m_lpw |
int | m_pixels |
int | m_pixelsBig |
bool | m_muted |
float | m_savedLevel |
bool | m_provideContextMenu |
QMenu * | m_lastContextMenu |
Private Slots | |
void | selfLevelChanged (float) |
void | selfClicked () |
Detailed Description
Definition at line 23 of file LevelPanToolButton.h.
Constructor & Destructor Documentation
LevelPanToolButton::LevelPanToolButton | ( | QWidget * | parent = 0 | ) |
Definition at line 34 of file LevelPanToolButton.cpp.
References contextMenuRequested(), levelChanged(), m_lpw, m_pixels, m_pixelsBig, panChanged(), selfClicked(), selfLevelChanged(), setBigImageSize(), and setImageSize().
LevelPanToolButton::~LevelPanToolButton | ( | ) |
Definition at line 70 of file LevelPanToolButton.cpp.
References m_lastContextMenu.
Member Function Documentation
float LevelPanToolButton::getLevel | ( | ) | const |
Return level as a gain value in the range [0,1].
Definition at line 95 of file LevelPanToolButton.cpp.
References LevelPanWidget::getLevel(), and m_lpw.
float LevelPanToolButton::getPan | ( | ) | const |
Return pan as a value in the range [-1,1].
Definition at line 101 of file LevelPanToolButton.cpp.
References LevelPanWidget::getPan(), and m_lpw.
bool LevelPanToolButton::includesMute | ( | ) | const |
Discover whether the level range includes muting or not.
Definition at line 107 of file LevelPanToolButton.cpp.
References LevelPanWidget::includesMute(), and m_lpw.
void LevelPanToolButton::setImageSize | ( | int | pixels | ) |
Definition at line 113 of file LevelPanToolButton.cpp.
References m_pixels.
Referenced by LevelPanToolButton(), paintEvent(), and PropertyBox::populateViewPlayFrame().
void LevelPanToolButton::setProvideContextMenu | ( | bool | provide | ) |
Specify whether a right-click context menu is provided.
Definition at line 123 of file LevelPanToolButton.cpp.
References m_provideContextMenu.
void LevelPanToolButton::setBigImageSize | ( | int | pixels | ) |
Definition at line 129 of file LevelPanToolButton.cpp.
References m_lpw, and m_pixelsBig.
Referenced by LevelPanToolButton().
|
slot |
Set level in the range [0,1] – will be rounded.
Definition at line 138 of file LevelPanToolButton.cpp.
References m_lpw, and LevelPanWidget::setLevel().
|
slot |
Set pan in the range [-1,1] – will be rounded.
Definition at line 145 of file LevelPanToolButton.cpp.
References m_lpw, and LevelPanWidget::setPan().
|
slot |
Set left and right peak monitoring levels in the range [0,1].
Definition at line 152 of file LevelPanToolButton.cpp.
References m_lpw, and LevelPanWidget::setMonitoringLevels().
|
slot |
Specify whether the level range should include muting or not.
Definition at line 159 of file LevelPanToolButton.cpp.
References m_lpw, and LevelPanWidget::setIncludeMute().
|
slot |
Definition at line 166 of file LevelPanToolButton.cpp.
References m_lpw.
|
protectedslot |
Definition at line 201 of file LevelPanToolButton.cpp.
References MenuTitle::addTitle(), LevelPanWidget::getLevel(), LevelPanWidget::getPan(), m_lastContextMenu, m_lpw, m_muted, and m_provideContextMenu.
Referenced by LevelPanToolButton().
|
signal |
Referenced by LevelPanToolButton(), and selfClicked().
|
signal |
Referenced by LevelPanToolButton().
|
signal |
Referenced by enterEvent().
|
signal |
Referenced by leaveEvent().
|
privateslot |
Definition at line 173 of file LevelPanToolButton.cpp.
References m_muted, and m_savedLevel.
Referenced by LevelPanToolButton().
|
privateslot |
Definition at line 185 of file LevelPanToolButton.cpp.
References LevelPanWidget::getLevel(), levelChanged(), m_lpw, m_muted, m_savedLevel, and LevelPanWidget::setLevel().
Referenced by LevelPanToolButton().
|
overrideprotected |
Definition at line 236 of file LevelPanToolButton.cpp.
References m_lpw, m_pixels, LevelPanWidget::renderTo(), and setImageSize().
|
overrideprotected |
Definition at line 253 of file LevelPanToolButton.cpp.
References mouseEntered().
|
overrideprotected |
Definition at line 260 of file LevelPanToolButton.cpp.
References mouseLeft().
|
overrideprotected |
Definition at line 76 of file LevelPanToolButton.cpp.
References m_lpw, and LevelPanWidget::setToDefault().
|
overrideprotected |
Definition at line 89 of file LevelPanToolButton.cpp.
References m_lpw, and LevelPanWidget::wheelEvent().
Member Data Documentation
|
protected |
Definition at line 83 of file LevelPanToolButton.h.
Referenced by contextMenuRequested(), getLevel(), getPan(), includesMute(), LevelPanToolButton(), mousePressEvent(), paintEvent(), selfClicked(), setBigImageSize(), setEnabled(), setIncludeMute(), setLevel(), setMonitoringLevels(), setPan(), and wheelEvent().
|
protected |
Definition at line 84 of file LevelPanToolButton.h.
Referenced by LevelPanToolButton(), paintEvent(), and setImageSize().
|
protected |
Definition at line 85 of file LevelPanToolButton.h.
Referenced by LevelPanToolButton(), and setBigImageSize().
|
protected |
Definition at line 86 of file LevelPanToolButton.h.
Referenced by contextMenuRequested(), selfClicked(), and selfLevelChanged().
|
protected |
Definition at line 87 of file LevelPanToolButton.h.
Referenced by selfClicked(), and selfLevelChanged().
|
protected |
Definition at line 88 of file LevelPanToolButton.h.
Referenced by contextMenuRequested(), and setProvideContextMenu().
|
protected |
Definition at line 89 of file LevelPanToolButton.h.
Referenced by contextMenuRequested(), and ~LevelPanToolButton().
The documentation for this class was generated from the following files:
Generated by
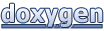