svgui
1.9
|
LevelPanToolButton.cpp
Go to the documentation of this file.
void setLevel(float)
Set level in the range [0,1] – will be rounded.
Definition: LevelPanToolButton.cpp:138
float getLevel() const
Return level as a gain value in the range [0,1].
Definition: LevelPanToolButton.cpp:95
void setPan(float)
Set pan in the range [-1,1]. The value will be rounded.
Definition: LevelPanWidget.cpp:172
void mouseEntered()
void wheelEvent(QWheelEvent *e) override
Definition: LevelPanToolButton.cpp:89
LevelPanToolButton(QWidget *parent=0)
Definition: LevelPanToolButton.cpp:34
void setIncludeMute(bool)
Specify whether the level range should include muting or not.
Definition: LevelPanWidget.cpp:215
bool includesMute() const
Discover whether the level range includes muting or not.
Definition: LevelPanToolButton.cpp:107
float getPan() const
Return pan as a value in the range [-1,1].
Definition: LevelPanToolButton.cpp:101
void setPan(float)
Set pan in the range [-1,1] – will be rounded.
Definition: LevelPanToolButton.cpp:145
void selfLevelChanged(float)
Definition: LevelPanToolButton.cpp:173
void renderTo(QPaintDevice *, QRectF, bool asIfEditable) const
Draw a suitably sized copy of the widget's contents to the given device.
Definition: LevelPanWidget.cpp:405
void setMonitoringLevels(float, float)
Set left and right peak monitoring levels in the range [0,1].
Definition: LevelPanToolButton.cpp:152
void paintEvent(QPaintEvent *) override
Definition: LevelPanToolButton.cpp:236
void panChanged(float)
void wheelEvent(QWheelEvent *ev) override
Definition: LevelPanWidget.cpp:283
void setIncludeMute(bool)
Specify whether the level range should include muting or not.
Definition: LevelPanToolButton.cpp:159
void setMonitoringLevels(float, float)
Set left and right peak monitoring levels in the range [0,1].
Definition: LevelPanWidget.cpp:188
bool m_provideContextMenu
Definition: LevelPanToolButton.h:88
void mouseLeft()
void contextMenuRequested(const QPoint &)
Definition: LevelPanToolButton.cpp:201
void enterEvent(QEvent *) override
Definition: LevelPanToolButton.cpp:253
void setBigImageSize(int pixels)
Definition: LevelPanToolButton.cpp:129
void leaveEvent(QEvent *) override
Definition: LevelPanToolButton.cpp:260
void levelChanged(float)
bool includesMute() const
Discover whether the level range includes muting or not.
Definition: LevelPanWidget.cpp:202
void mousePressEvent(QMouseEvent *) override
Definition: LevelPanToolButton.cpp:76
void setProvideContextMenu(bool)
Specify whether a right-click context menu is provided.
Definition: LevelPanToolButton.cpp:123
Generated by
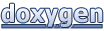