svgui
1.9
|
A simple widget for coarse level and pan control. More...
#include <LevelPanWidget.h>
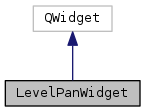
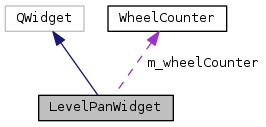
Public Slots | |
void | setLevel (float) |
Set level. More... | |
void | setPan (float) |
Set pan in the range [-1,1]. The value will be rounded. More... | |
void | setMonitoringLevels (float, float) |
Set left and right peak monitoring levels in the range [0,1]. More... | |
void | setEditable (bool) |
Specify whether the widget is editable or read-only (default editable) More... | |
void | setIncludeMute (bool) |
Specify whether the level range should include muting or not. More... | |
void | setToDefault () |
Reset to default values. More... | |
void | wheelEvent (QWheelEvent *ev) override |
Signals | |
void | levelChanged (float) |
void | panChanged (float) |
void | mouseEntered () |
void | mouseLeft () |
Public Member Functions | |
LevelPanWidget (QWidget *parent=0) | |
~LevelPanWidget () | |
float | getLevel () const |
Return level as a gain value. More... | |
float | getPan () const |
Return pan as a value in the range [-1,1]. More... | |
bool | isEditable () const |
Find out whether the widget is editable. More... | |
bool | includesMute () const |
Discover whether the level range includes muting or not. More... | |
void | renderTo (QPaintDevice *, QRectF, bool asIfEditable) const |
Draw a suitably sized copy of the widget's contents to the given device. More... | |
QSize | sizeHint () const override |
Protected Member Functions | |
void | mousePressEvent (QMouseEvent *ev) override |
void | mouseMoveEvent (QMouseEvent *ev) override |
void | mouseReleaseEvent (QMouseEvent *ev) override |
void | paintEvent (QPaintEvent *ev) override |
void | enterEvent (QEvent *) override |
void | leaveEvent (QEvent *) override |
void | emitLevelChanged () |
void | emitPanChanged () |
int | clampNotch (int notch) const |
int | clampPan (int pan) const |
int | audioLevelToNotch (float audioLevel) const |
float | notchToAudioLevel (int notch) const |
int | audioPanToPan (float audioPan) const |
float | panToAudioPan (int pan) const |
int | coordsToNotch (QRectF rect, QPointF pos) const |
int | coordsToPan (QRectF rect, QPointF pos) const |
QColor | cellToColour (int cell) const |
QSizeF | cellSize (QRectF) const |
QPointF | cellCentre (QRectF, int row, int col) const |
QSizeF | cellLightSize (QRectF) const |
QRectF | cellLightRect (QRectF, int row, int col) const |
QRectF | cellOutlineRect (QRectF, int row, int col) const |
double | thinLineWidth (QRectF) const |
double | cornerRadius (QRectF) const |
Protected Attributes | |
int | m_minNotch |
int | m_maxNotch |
int | m_notch |
int | m_pan |
float | m_monitorLeft |
float | m_monitorRight |
bool | m_editable |
bool | m_editing |
bool | m_includeMute |
bool | m_includeHalfSteps |
WheelCounter | m_wheelCounter |
Detailed Description
A simple widget for coarse level and pan control.
Definition at line 26 of file LevelPanWidget.h.
Constructor & Destructor Documentation
LevelPanWidget::LevelPanWidget | ( | QWidget * | parent = 0 | ) |
Definition at line 66 of file LevelPanWidget.cpp.
References setLevel(), and setPan().
LevelPanWidget::~LevelPanWidget | ( | ) |
Definition at line 84 of file LevelPanWidget.cpp.
Member Function Documentation
float LevelPanWidget::getLevel | ( | ) | const |
Return level as a gain value.
The basic level range is [0,1] but the gain scale may go up to 4.0
Definition at line 152 of file LevelPanWidget.cpp.
References m_notch, and notchToAudioLevel().
Referenced by LevelPanToolButton::contextMenuRequested(), emitLevelChanged(), LevelPanToolButton::getLevel(), LevelPanToolButton::selfClicked(), and setLevel().
float LevelPanWidget::getPan | ( | ) | const |
Return pan as a value in the range [-1,1].
Definition at line 182 of file LevelPanWidget.cpp.
References m_pan, and panToAudioPan().
Referenced by LevelPanToolButton::contextMenuRequested(), emitPanChanged(), and LevelPanToolButton::getPan().
bool LevelPanWidget::isEditable | ( | ) | const |
Find out whether the widget is editable.
Definition at line 196 of file LevelPanWidget.cpp.
References m_editable.
bool LevelPanWidget::includesMute | ( | ) | const |
Discover whether the level range includes muting or not.
Definition at line 202 of file LevelPanWidget.cpp.
References m_includeMute.
Referenced by LevelPanToolButton::includesMute().
void LevelPanWidget::renderTo | ( | QPaintDevice * | dev, |
QRectF | rect, | ||
bool | asIfEditable | ||
) | const |
Draw a suitably sized copy of the widget's contents to the given device.
Definition at line 405 of file LevelPanWidget.cpp.
References audioLevelToNotch(), cellCentre(), cellLightRect(), cellOutlineRect(), cellToColour(), cornerRadius(), m_includeMute, m_maxNotch, m_monitorLeft, m_monitorRight, m_notch, m_pan, maxPan, and thinLineWidth().
Referenced by LevelPanToolButton::paintEvent(), and paintEvent().
|
override |
Definition at line 98 of file LevelPanWidget.cpp.
References WidgetScale::scaleQSize().
|
slot |
Set level.
The basic level range is [0,1] but the scale may go higher. The value will be rounded.
Definition at line 138 of file LevelPanWidget.cpp.
References audioLevelToNotch(), emitLevelChanged(), getLevel(), and m_notch.
Referenced by LevelPanWidget(), LevelPanToolButton::selfClicked(), LevelPanToolButton::setLevel(), and setToDefault().
|
slot |
Set pan in the range [-1,1]. The value will be rounded.
Definition at line 172 of file LevelPanWidget.cpp.
References audioPanToPan(), and m_pan.
Referenced by LevelPanWidget(), LevelPanToolButton::setPan(), and setToDefault().
|
slot |
Set left and right peak monitoring levels in the range [0,1].
Definition at line 188 of file LevelPanWidget.cpp.
References m_monitorLeft, and m_monitorRight.
Referenced by LevelPanToolButton::setMonitoringLevels().
|
slot |
Specify whether the widget is editable or read-only (default editable)
Definition at line 208 of file LevelPanWidget.cpp.
References m_editable.
|
slot |
Specify whether the level range should include muting or not.
Definition at line 215 of file LevelPanWidget.cpp.
References emitLevelChanged(), m_includeMute, and m_minNotch.
Referenced by LevelPanToolButton::setIncludeMute().
|
slot |
Reset to default values.
Definition at line 89 of file LevelPanWidget.cpp.
References emitLevelChanged(), emitPanChanged(), setLevel(), and setPan().
Referenced by LevelPanToolButton::mousePressEvent(), and mousePressEvent().
|
overrideslot |
Definition at line 283 of file LevelPanWidget.cpp.
References clampNotch(), clampPan(), WheelCounter::count(), emitLevelChanged(), emitPanChanged(), m_notch, m_pan, and m_wheelCounter.
Referenced by LevelPanToolButton::wheelEvent().
|
signal |
Referenced by emitLevelChanged().
|
signal |
Referenced by emitPanChanged().
|
signal |
Referenced by enterEvent().
|
signal |
Referenced by leaveEvent().
|
overrideprotected |
Definition at line 240 of file LevelPanWidget.cpp.
References m_editing, mouseMoveEvent(), and setToDefault().
|
overrideprotected |
Definition at line 260 of file LevelPanWidget.cpp.
References coordsToNotch(), coordsToPan(), emitLevelChanged(), emitPanChanged(), m_editable, m_editing, m_notch, and m_pan.
Referenced by mousePressEvent(), and mouseReleaseEvent().
|
overrideprotected |
Definition at line 253 of file LevelPanWidget.cpp.
References m_editing, and mouseMoveEvent().
|
overrideprotected |
Definition at line 575 of file LevelPanWidget.cpp.
References m_editable, and renderTo().
|
overrideprotected |
Definition at line 581 of file LevelPanWidget.cpp.
References mouseEntered().
|
overrideprotected |
Definition at line 588 of file LevelPanWidget.cpp.
References mouseLeft().
|
protected |
Definition at line 228 of file LevelPanWidget.cpp.
References getLevel(), and levelChanged().
Referenced by mouseMoveEvent(), setIncludeMute(), setLevel(), setToDefault(), and wheelEvent().
|
protected |
Definition at line 234 of file LevelPanWidget.cpp.
References getPan(), and panChanged().
Referenced by mouseMoveEvent(), setToDefault(), and wheelEvent().
|
protected |
Definition at line 104 of file LevelPanWidget.cpp.
References m_includeHalfSteps, m_maxNotch, and m_minNotch.
Referenced by audioLevelToNotch(), coordsToNotch(), and wheelEvent().
|
protected |
Definition at line 115 of file LevelPanWidget.cpp.
References maxPan.
Referenced by audioPanToPan(), coordsToPan(), and wheelEvent().
|
protected |
Definition at line 123 of file LevelPanWidget.cpp.
References clampNotch(), and m_maxNotch.
Referenced by renderTo(), and setLevel().
|
protected |
|
protected |
Definition at line 158 of file LevelPanWidget.cpp.
References clampPan(), and maxPan.
Referenced by setPan().
|
protected |
|
protected |
Definition at line 303 of file LevelPanWidget.cpp.
References clampNotch(), and m_maxNotch.
Referenced by mouseMoveEvent().
|
protected |
Definition at line 317 of file LevelPanWidget.cpp.
References clampPan(), and maxPan.
Referenced by mouseMoveEvent().
|
protected |
Definition at line 395 of file LevelPanWidget.cpp.
Referenced by renderTo().
|
protected |
Definition at line 331 of file LevelPanWidget.cpp.
References m_maxNotch, and maxPan.
Referenced by cellCentre(), cellLightSize(), and cornerRadius().
|
protected |
Definition at line 341 of file LevelPanWidget.cpp.
References cellSize(), and maxPan.
Referenced by cellLightRect(), and renderTo().
|
protected |
Definition at line 351 of file LevelPanWidget.cpp.
References cellSize().
Referenced by cellLightRect().
|
protected |
Definition at line 360 of file LevelPanWidget.cpp.
References cellCentre(), and cellLightSize().
Referenced by cellOutlineRect(), and renderTo().
|
protected |
Definition at line 387 of file LevelPanWidget.cpp.
References cellLightRect(), and thinLineWidth().
Referenced by renderTo().
|
protected |
Definition at line 371 of file LevelPanWidget.cpp.
References m_maxNotch, and maxPan.
Referenced by cellOutlineRect(), and renderTo().
|
protected |
Member Data Documentation
|
protected |
Definition at line 93 of file LevelPanWidget.h.
Referenced by clampNotch(), and setIncludeMute().
|
protected |
Definition at line 94 of file LevelPanWidget.h.
Referenced by audioLevelToNotch(), cellSize(), clampNotch(), coordsToNotch(), notchToAudioLevel(), renderTo(), and thinLineWidth().
|
protected |
Definition at line 95 of file LevelPanWidget.h.
Referenced by getLevel(), mouseMoveEvent(), renderTo(), setLevel(), and wheelEvent().
|
protected |
Definition at line 96 of file LevelPanWidget.h.
Referenced by getPan(), mouseMoveEvent(), renderTo(), setPan(), and wheelEvent().
|
protected |
Definition at line 97 of file LevelPanWidget.h.
Referenced by renderTo(), and setMonitoringLevels().
|
protected |
Definition at line 98 of file LevelPanWidget.h.
Referenced by renderTo(), and setMonitoringLevels().
|
protected |
Definition at line 99 of file LevelPanWidget.h.
Referenced by isEditable(), mouseMoveEvent(), paintEvent(), and setEditable().
|
protected |
Definition at line 100 of file LevelPanWidget.h.
Referenced by mouseMoveEvent(), mousePressEvent(), and mouseReleaseEvent().
|
protected |
Definition at line 101 of file LevelPanWidget.h.
Referenced by includesMute(), renderTo(), and setIncludeMute().
|
protected |
Definition at line 102 of file LevelPanWidget.h.
Referenced by clampNotch().
|
protected |
Definition at line 104 of file LevelPanWidget.h.
Referenced by wheelEvent().
The documentation for this class was generated from the following files:
Generated by
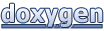