svgui
1.9
|
LevelPanWidget.cpp
Go to the documentation of this file.
QRectF cellOutlineRect(QRectF, int row, int col) const
Definition: LevelPanWidget.cpp:387
void setPan(float)
Set pan in the range [-1,1]. The value will be rounded.
Definition: LevelPanWidget.cpp:172
QPointF cellCentre(QRectF, int row, int col) const
Definition: LevelPanWidget.cpp:341
int coordsToNotch(QRectF rect, QPointF pos) const
Definition: LevelPanWidget.cpp:303
QRectF cellLightRect(QRectF, int row, int col) const
Definition: LevelPanWidget.cpp:360
void mouseMoveEvent(QMouseEvent *ev) override
Definition: LevelPanWidget.cpp:260
void mouseLeft()
void levelChanged(float)
void setIncludeMute(bool)
Specify whether the level range should include muting or not.
Definition: LevelPanWidget.cpp:215
void mousePressEvent(QMouseEvent *ev) override
Definition: LevelPanWidget.cpp:240
int audioLevelToNotch(float audioLevel) const
Definition: LevelPanWidget.cpp:123
void setEditable(bool)
Specify whether the widget is editable or read-only (default editable)
Definition: LevelPanWidget.cpp:208
void renderTo(QPaintDevice *, QRectF, bool asIfEditable) const
Draw a suitably sized copy of the widget's contents to the given device.
Definition: LevelPanWidget.cpp:405
void mouseReleaseEvent(QMouseEvent *ev) override
Definition: LevelPanWidget.cpp:253
void wheelEvent(QWheelEvent *ev) override
Definition: LevelPanWidget.cpp:283
void setMonitoringLevels(float, float)
Set left and right peak monitoring levels in the range [0,1].
Definition: LevelPanWidget.cpp:188
void panChanged(float)
float notchToAudioLevel(int notch) const
Definition: LevelPanWidget.cpp:131
void mouseEntered()
int coordsToPan(QRectF rect, QPointF pos) const
Definition: LevelPanWidget.cpp:317
void paintEvent(QPaintEvent *ev) override
Definition: LevelPanWidget.cpp:575
bool includesMute() const
Discover whether the level range includes muting or not.
Definition: LevelPanWidget.cpp:202
int audioPanToPan(float audioPan) const
Definition: LevelPanWidget.cpp:158
Generated by
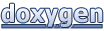