svcore
1.9
|
#include <OSCQueue.h>
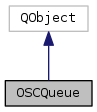
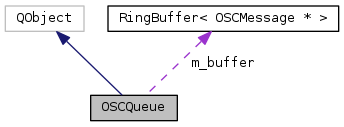
Signals | |
void | messagesAvailable () |
Public Member Functions | |
OSCQueue (bool withNetworkPort) | |
virtual | ~OSCQueue () |
bool | isOK () const |
bool | isEmpty () const |
int | getMessagesAvailable () const |
void | postMessage (OSCMessage) |
OSCMessage | readMessage () |
QString | getOSCURL () const |
bool | hasPort () const |
Protected Member Functions | |
bool | parseOSCPath (QString path, int &target, int &targetData, QString &method) |
Static Protected Member Functions | |
static void | oscError (int, const char *, const char *) |
static int | oscMessageHandler (const char *, const char *, lo_arg **, int, lo_message, void *) |
Protected Attributes | |
lo_server_thread | m_thread |
bool | m_withPort |
RingBuffer< OSCMessage * > | m_buffer |
Detailed Description
Definition at line 34 of file OSCQueue.h.
Constructor & Destructor Documentation
OSCQueue::OSCQueue | ( | bool | withNetworkPort | ) |
Definition at line 93 of file OSCQueue.cpp.
References m_thread, m_withPort, oscError(), oscMessageHandler(), and SVDEBUG.
|
virtual |
Definition at line 127 of file OSCQueue.cpp.
References RingBuffer< T, N >::getReadSpace(), m_buffer, m_thread, and RingBuffer< T, N >::readOne().
Member Function Documentation
bool OSCQueue::isOK | ( | ) | const |
Definition at line 141 of file OSCQueue.cpp.
References m_thread, and m_withPort.
|
inline |
Definition at line 44 of file OSCQueue.h.
References getMessagesAvailable(), getOSCURL(), postMessage(), and readMessage().
int OSCQueue::getMessagesAvailable | ( | ) | const |
Definition at line 167 of file OSCQueue.cpp.
References RingBuffer< T, N >::getReadSpace(), and m_buffer.
Referenced by isEmpty().
void OSCQueue::postMessage | ( | OSCMessage | message | ) |
Definition at line 184 of file OSCQueue.cpp.
References OSCMessage::getMethod(), RingBuffer< T, N >::getSize(), OSCMessage::getTarget(), OSCMessage::getTargetData(), RingBuffer< T, N >::getWriteSpace(), m_buffer, messagesAvailable(), SVDEBUG, and RingBuffer< T, N >::write().
Referenced by isEmpty(), and oscMessageHandler().
OSCMessage OSCQueue::readMessage | ( | ) |
Definition at line 173 of file OSCQueue.cpp.
References m_buffer, RingBuffer< T, N >::readOne(), SVDEBUG, and OSCMessage::toString().
Referenced by isEmpty().
QString OSCQueue::getOSCURL | ( | ) | const |
|
inline |
Definition at line 51 of file OSCQueue.h.
References m_withPort, and messagesAvailable().
|
signal |
Referenced by hasPort(), and postMessage().
|
staticprotected |
|
staticprotected |
Definition at line 42 of file OSCQueue.cpp.
References OSCMessage::addArg(), parseOSCPath(), postMessage(), OSCMessage::setMethod(), OSCMessage::setTarget(), and OSCMessage::setTargetData().
Referenced by OSCQueue().
|
protected |
Member Data Documentation
|
protected |
Definition at line 58 of file OSCQueue.h.
Referenced by getOSCURL(), isOK(), OSCQueue(), and ~OSCQueue().
|
protected |
Definition at line 67 of file OSCQueue.h.
Referenced by hasPort(), isOK(), and OSCQueue().
|
protected |
Definition at line 68 of file OSCQueue.h.
Referenced by getMessagesAvailable(), postMessage(), readMessage(), and ~OSCQueue().
The documentation for this class was generated from the following files:
Generated by
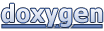