svcore
1.9
|
#include <OSCMessage.h>
Public Member Functions | |
OSCMessage () | |
~OSCMessage () | |
void | setTarget (const int &target) |
int | getTarget () const |
void | setTargetData (const int &targetData) |
int | getTargetData () const |
void | setMethod (QString method) |
QString | getMethod () const |
void | clearArgs () |
void | addArg (QVariant arg) |
int | getArgCount () const |
const QVariant & | getArg (int i) const |
QString | toString () const |
Private Attributes | |
int | m_target |
int | m_targetData |
QString | m_method |
std::vector< QVariant > | m_args |
Detailed Description
Definition at line 32 of file OSCMessage.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 35 of file OSCMessage.h.
References ~OSCMessage().
OSCMessage::~OSCMessage | ( | ) |
Member Function Documentation
|
inline |
Definition at line 38 of file OSCMessage.h.
References m_target.
Referenced by OSCQueue::oscMessageHandler().
|
inline |
Definition at line 39 of file OSCMessage.h.
References m_target.
Referenced by OSCQueue::postMessage().
|
inline |
Definition at line 41 of file OSCMessage.h.
References m_targetData.
Referenced by OSCQueue::oscMessageHandler().
|
inline |
Definition at line 42 of file OSCMessage.h.
References m_targetData.
Referenced by OSCQueue::postMessage().
|
inline |
Definition at line 44 of file OSCMessage.h.
References m_method.
Referenced by OSCQueue::oscMessageHandler().
|
inline |
Definition at line 45 of file OSCMessage.h.
References addArg(), clearArgs(), getArg(), getArgCount(), and m_method.
Referenced by OSCQueue::postMessage().
void OSCMessage::clearArgs | ( | ) |
Definition at line 30 of file OSCMessage.cpp.
References m_args.
Referenced by getMethod(), and ~OSCMessage().
void OSCMessage::addArg | ( | QVariant | arg | ) |
Definition at line 36 of file OSCMessage.cpp.
References m_args.
Referenced by getMethod(), and OSCQueue::oscMessageHandler().
int OSCMessage::getArgCount | ( | ) | const |
const QVariant & OSCMessage::getArg | ( | int | i | ) | const |
|
inline |
Definition at line 54 of file OSCMessage.h.
References m_args, m_method, m_target, and m_targetData.
Referenced by OSCQueue::readMessage().
Member Data Documentation
|
private |
Definition at line 66 of file OSCMessage.h.
Referenced by getTarget(), setTarget(), and toString().
|
private |
Definition at line 67 of file OSCMessage.h.
Referenced by getTargetData(), setTargetData(), and toString().
|
private |
Definition at line 68 of file OSCMessage.h.
Referenced by getMethod(), setMethod(), and toString().
|
private |
Definition at line 69 of file OSCMessage.h.
Referenced by addArg(), clearArgs(), getArg(), getArgCount(), and toString().
The documentation for this class was generated from the following files:
Generated by
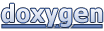