svcore
1.9
|
OSCQueue.h
Go to the documentation of this file.
void messagesAvailable()
Definition: OSCMessage.h:32
static int oscMessageHandler(const char *, const char *, lo_arg **, int, lo_message, void *)
Definition: OSCQueue.cpp:42
Definition: OSCQueue.h:34
bool parseOSCPath(QString path, int &target, int &targetData, QString &method)
Definition: OSCQueue.cpp:212
Generated by
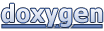