svcore
1.9
|
Write a MIDI file. More...
#include <MIDIFileWriter.h>
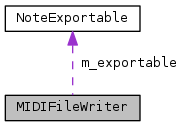
Public Member Functions | |
MIDIFileWriter (QString path, const NoteExportable *exportable, sv_samplerate_t sampleRate, float tempo=120.f) | |
~MIDIFileWriter () | |
bool | isOK () const |
QString | getError () const |
void | write () |
Protected Types | |
enum | MIDIFileFormatType { MIDI_SINGLE_TRACK_FILE = 0x00, MIDI_SIMULTANEOUS_TRACK_FILE = 0x01, MIDI_SEQUENTIAL_TRACK_FILE = 0x02, MIDI_FILE_BAD_FORMAT = 0xFF } |
typedef std::vector< MIDIEvent * > | MIDITrack |
typedef std::map< unsigned int, MIDITrack > | MIDIComposition |
Protected Member Functions | |
std::string | intToMIDIBytes (int number) const |
std::string | longToMIDIBytes (unsigned long number) const |
std::string | longToVarBuffer (unsigned long number) const |
unsigned long | getMIDITimeForTime (RealTime t) const |
bool | writeHeader () |
bool | writeTrack (int track) |
bool | writeComposition () |
bool | convert () |
Protected Attributes | |
QString | m_path |
const NoteExportable * | m_exportable |
sv_samplerate_t | m_sampleRate |
float | m_tempo |
int | m_timingDivision |
MIDIFileFormatType | m_format |
unsigned int | m_numberOfTracks |
MIDIComposition | m_midiComposition |
std::ofstream * | m_midiFile |
QString | m_error |
Detailed Description
Write a MIDI file.
This includes file write code for generic simultaneous-track MIDI files, but the conversion stage only supports a single-track MIDI file with fixed tempo, time signature and timing division.
Definition at line 44 of file MIDIFileWriter.h.
Member Typedef Documentation
|
protected |
Definition at line 59 of file MIDIFileWriter.h.
|
protected |
Definition at line 60 of file MIDIFileWriter.h.
Member Enumeration Documentation
|
protected |
Enumerator | |
---|---|
MIDI_SINGLE_TRACK_FILE | |
MIDI_SIMULTANEOUS_TRACK_FILE | |
MIDI_SEQUENTIAL_TRACK_FILE | |
MIDI_FILE_BAD_FORMAT |
Definition at line 62 of file MIDIFileWriter.h.
Constructor & Destructor Documentation
MIDIFileWriter::MIDIFileWriter | ( | QString | path, |
const NoteExportable * | exportable, | ||
sv_samplerate_t | sampleRate, | ||
float | tempo = 120.f |
||
) |
Definition at line 44 of file MIDIFileWriter.cpp.
MIDIFileWriter::~MIDIFileWriter | ( | ) |
Definition at line 57 of file MIDIFileWriter.cpp.
References m_midiComposition.
Member Function Documentation
bool MIDIFileWriter::isOK | ( | ) | const |
Definition at line 74 of file MIDIFileWriter.cpp.
References m_error.
QString MIDIFileWriter::getError | ( | ) | const |
Definition at line 80 of file MIDIFileWriter.cpp.
References m_error.
void MIDIFileWriter::write | ( | ) |
Definition at line 86 of file MIDIFileWriter.cpp.
References writeComposition().
|
protected |
Definition at line 92 of file MIDIFileWriter.cpp.
Referenced by writeHeader().
|
protected |
Definition at line 107 of file MIDIFileWriter.cpp.
Referenced by writeTrack().
|
protected |
Definition at line 132 of file MIDIFileWriter.cpp.
Referenced by writeTrack().
|
protected |
|
protected |
Definition at line 166 of file MIDIFileWriter.cpp.
References intToMIDIBytes(), m_format, m_midiFile, m_numberOfTracks, m_timingDivision, and MIDIConstants::MIDI_FILE_HEADER.
Referenced by writeComposition().
|
protected |
Definition at line 188 of file MIDIFileWriter.cpp.
References longToMIDIBytes(), longToVarBuffer(), m_midiComposition, m_midiFile, MIDIConstants::MIDI_CHNL_AFTERTOUCH, MIDIConstants::MIDI_CTRL_CHANGE, MIDIConstants::MIDI_FILE_META_EVENT, MIDIConstants::MIDI_NOTE_OFF, MIDIConstants::MIDI_NOTE_ON, MIDIConstants::MIDI_PITCH_BEND, MIDIConstants::MIDI_POLY_AFTERTOUCH, MIDIConstants::MIDI_PROG_CHANGE, MIDIConstants::MIDI_SYSTEM_EXCLUSIVE, and MIDIConstants::MIDI_TRACK_HEADER.
Referenced by writeComposition().
|
protected |
Definition at line 282 of file MIDIFileWriter.cpp.
References m_error, m_midiFile, m_numberOfTracks, m_path, writeHeader(), and writeTrack().
Referenced by write().
|
protected |
Definition at line 318 of file MIDIFileWriter.cpp.
References NoteExportable::getNotes(), m_exportable, m_format, m_midiComposition, m_numberOfTracks, m_sampleRate, m_tempo, m_timingDivision, MIDIConstants::MIDI_CUE_POINT, MIDIConstants::MIDI_END_OF_TRACK, MIDIConstants::MIDI_FILE_META_EVENT, MIDIConstants::MIDI_NOTE_OFF, MIDIConstants::MIDI_NOTE_ON, MIDIConstants::MIDI_SET_TEMPO, MIDI_SINGLE_TRACK_FILE, and notes.
Referenced by MIDIFileWriter().
Member Data Documentation
|
protected |
Definition at line 81 of file MIDIFileWriter.h.
Referenced by writeComposition().
|
protected |
Definition at line 82 of file MIDIFileWriter.h.
Referenced by convert().
|
protected |
Definition at line 83 of file MIDIFileWriter.h.
Referenced by convert().
|
protected |
Definition at line 84 of file MIDIFileWriter.h.
Referenced by convert().
|
protected |
Definition at line 85 of file MIDIFileWriter.h.
Referenced by convert(), and writeHeader().
|
protected |
Definition at line 86 of file MIDIFileWriter.h.
Referenced by convert(), and writeHeader().
|
protected |
Definition at line 87 of file MIDIFileWriter.h.
Referenced by convert(), writeComposition(), and writeHeader().
|
protected |
Definition at line 89 of file MIDIFileWriter.h.
Referenced by convert(), writeTrack(), and ~MIDIFileWriter().
|
protected |
Definition at line 91 of file MIDIFileWriter.h.
Referenced by writeComposition(), writeHeader(), and writeTrack().
|
protected |
Definition at line 92 of file MIDIFileWriter.h.
Referenced by getError(), isOK(), MIDIFileWriter(), and writeComposition().
The documentation for this class was generated from the following files:
Generated by
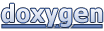