qm-dsp
1.8
|
#include <TempoTrack.h>
Public Member Functions | |
TempoTrack (TTParams Params) | |
virtual | ~TempoTrack () |
std::vector< int > | process (std::vector< double > DF, std::vector< double > *tempoReturn=0) |
Private Member Functions | |
void | initialise (TTParams Params) |
void | deInitialise () |
int | beatPredict (int FSP, double alignment, double period, int step) |
int | phaseMM (double *DF, double *weighting, int winLength, double period) |
void | createPhaseExtractor (double *Filter, int winLength, double period, int fsp, int lastBeat) |
int | findMeter (double *ACF, int len, double period) |
void | constDetect (double *periodP, int currentIdx, int *flag) |
void | stepDetect (double *periodP, double *periodG, int currentIdx, int *flag) |
void | createCombFilter (double *Filter, int winLength, int TSig, double beatLag) |
double | tempoMM (double *ACF, double *weight, int sig) |
Private Attributes | |
int | m_dataLength |
int | m_winLength |
int | m_lagLength |
double | m_rayparam |
double | m_sigma |
double | m_DFWVNnorm |
std::vector< int > | m_beats |
double | m_lockedTempo |
double * | m_tempoScratch |
double * | m_smoothRCF |
double * | m_rawDFFrame |
double * | m_smoothDFFrame |
double * | m_frameACF |
double * | m_ACoeffs |
double * | m_BCoeffs |
Framer | m_DFFramer |
DFProcess * | m_DFConditioning |
Correlation | m_correlator |
DFProcConfig | m_DFPParams |
DFProcess * | m_RCFConditioning |
DFProcConfig | m_RCFPParams |
Detailed Description
Definition at line 45 of file TempoTrack.h.
Constructor & Destructor Documentation
TempoTrack::TempoTrack | ( | TTParams | Params | ) |
Definition at line 35 of file TempoTrack.cpp.
References initialise(), m_dataLength, m_DFWVNnorm, m_frameACF, m_lagLength, m_rawDFFrame, m_rayparam, m_sigma, m_smoothDFFrame, m_smoothRCF, m_tempoScratch, and m_winLength.
|
virtual |
Definition at line 54 of file TempoTrack.cpp.
References deInitialise().
Member Function Documentation
vector< int > TempoTrack::process | ( | std::vector< double > | DF, |
std::vector< double > * | tempoReturn = 0 |
||
) |
Definition at line 618 of file TempoTrack.cpp.
References beatPredict(), constDetect(), createCombFilter(), createPhaseExtractor(), Correlation::doAutoUnBiased(), findMeter(), Framer::getFrame(), Framer::getMaxNoFrames(), m_beats, m_correlator, m_dataLength, m_DFConditioning, m_DFFramer, m_frameACF, m_lagLength, m_lockedTempo, m_rawDFFrame, m_smoothDFFrame, m_winLength, phaseMM(), DFProcess::process(), Framer::setSource(), stepDetect(), and tempoMM().
|
private |
Definition at line 59 of file TempoTrack.cpp.
References TTParams::alpha, DFProcConfig::AlphaNormParam, Framer::configure(), DFProcConfig::isMedianPositive, TTParams::lagLength, DFProcConfig::length, DFProcConfig::LPACoeffs, TTParams::LPACoeffs, DFProcConfig::LPBCoeffs, TTParams::LPBCoeffs, DFProcConfig::LPOrd, TTParams::LPOrd, m_DFConditioning, m_DFFramer, m_DFPParams, m_DFWVNnorm, m_frameACF, m_lagLength, m_rawDFFrame, m_rayparam, m_RCFConditioning, m_RCFPParams, m_sigma, m_smoothDFFrame, m_smoothRCF, m_tempoScratch, m_winLength, WinThresh::post, WinThresh::pre, TTParams::winLength, DFProcConfig::winPost, DFProcConfig::winPre, and TTParams::WinT.
Referenced by TempoTrack().
|
private |
Definition at line 100 of file TempoTrack.cpp.
References m_DFConditioning, m_frameACF, m_rawDFFrame, m_RCFConditioning, m_smoothDFFrame, m_smoothRCF, and m_tempoScratch.
Referenced by ~TempoTrack().
|
private |
Definition at line 594 of file TempoTrack.cpp.
References m_beats, and MathUtilities::round().
Referenced by process().
|
private |
Definition at line 556 of file TempoTrack.cpp.
References MathUtilities::round().
Referenced by process().
|
private |
(double)winLength);
Definition at line 487 of file TempoTrack.cpp.
References MathUtilities::getFrameMinMax(), MathUtilities::round(), and TWO_PI.
Referenced by process().
|
private |
Definition at line 425 of file TempoTrack.cpp.
References MathUtilities::round().
Referenced by process().
|
private |
Definition at line 414 of file TempoTrack.cpp.
Referenced by process().
|
private |
Definition at line 399 of file TempoTrack.cpp.
Referenced by process().
|
private |
Definition at line 111 of file TempoTrack.cpp.
References m_rayparam, m_sigma, and TWO_PI.
Referenced by process().
|
private |
Definition at line 132 of file TempoTrack.cpp.
References m_lagLength, m_lockedTempo, m_RCFConditioning, m_smoothRCF, m_tempoScratch, m_winLength, MathUtilities::mean(), and DFProcess::process().
Referenced by process().
Member Data Documentation
|
private |
Definition at line 68 of file TempoTrack.h.
Referenced by process(), and TempoTrack().
|
private |
Definition at line 69 of file TempoTrack.h.
Referenced by initialise(), process(), tempoMM(), and TempoTrack().
|
private |
Definition at line 70 of file TempoTrack.h.
Referenced by initialise(), process(), tempoMM(), and TempoTrack().
|
private |
Definition at line 72 of file TempoTrack.h.
Referenced by createCombFilter(), initialise(), and TempoTrack().
|
private |
Definition at line 73 of file TempoTrack.h.
Referenced by createCombFilter(), initialise(), and TempoTrack().
|
private |
Definition at line 74 of file TempoTrack.h.
Referenced by initialise(), and TempoTrack().
|
private |
Definition at line 76 of file TempoTrack.h.
Referenced by beatPredict(), and process().
|
private |
Definition at line 78 of file TempoTrack.h.
|
private |
Definition at line 80 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), tempoMM(), and TempoTrack().
|
private |
Definition at line 81 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), tempoMM(), and TempoTrack().
|
private |
Definition at line 84 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), process(), and TempoTrack().
|
private |
Definition at line 85 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), process(), and TempoTrack().
|
private |
Definition at line 86 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), process(), and TempoTrack().
|
private |
Definition at line 89 of file TempoTrack.h.
|
private |
Definition at line 90 of file TempoTrack.h.
|
private |
Definition at line 93 of file TempoTrack.h.
Referenced by initialise(), and process().
|
private |
Definition at line 94 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), and process().
|
private |
Definition at line 95 of file TempoTrack.h.
Referenced by process().
|
private |
Definition at line 97 of file TempoTrack.h.
Referenced by initialise().
|
private |
Definition at line 100 of file TempoTrack.h.
Referenced by deInitialise(), initialise(), and tempoMM().
|
private |
Definition at line 102 of file TempoTrack.h.
Referenced by initialise().
The documentation for this class was generated from the following files:
Generated by
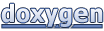