qm-dsp
1.8
|
TempoTrack.h
Go to the documentation of this file.
61 void createPhaseExtractor( double* Filter, int winLength, double period, int fsp, int lastBeat );
Definition: TempoTrack.h:33
Definition: Correlation.h:18
Definition: Filter.h:22
Definition: DFProcess.h:57
Definition: Framer.h:21
Definition: TempoTrack.h:27
Definition: DFProcess.h:31
Definition: TempoTrack.h:45
Generated by
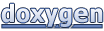