qm-dsp
1.8
|
ClusterMeltSegmenter.cpp
Go to the documentation of this file.
41 ncomponents(params.ncomponents), // NB currently not passed - no. of PCA components is set in cluser_segmenter.c
155 std::cerr << "ERROR: ClusterMeltSegmenter::extractFeatures: nsamples < windowsize (" << nsamples << " < " << getWindowsize() << ")" << std::endl;
251 std::cerr << "ERROR: ClusterMeltSegmenter::extractFeatures: nsamples < windowsize (" << nsamples << " < " << getWindowsize() << ")" << std::endl;
339 << " features with " << features[0].size() << " coefficients (ncoeff = " << ncoeff << ", ncomponents = " << ncomponents << ")" << std::endl;
361 cluster_segment(q, arrFeatures, features.size(), features[0].size(), nHMMStates, histogramLength,
void process(const double *src, double *dst)
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples...
Definition: Decimator.cpp:195
int neighbourhoodLimit
Definition: ClusterMeltSegmenter.h:107
void extractFeaturesMFCC(const double *, int)
Definition: ClusterMeltSegmenter.cpp:241
void extractFeaturesConstQ(const double *, int)
Definition: ClusterMeltSegmenter.cpp:145
virtual int getWindowsize()
Definition: ClusterMeltSegmenter.cpp:124
int process(const double *inframe, double *outceps)
Process time-domain input data.
Definition: MFCC.cpp:205
Definition: Window.h:26
static int getHighestSupportedFactor()
Definition: Decimator.h:57
Definition: segment.h:42
virtual ~ClusterMeltSegmenter()
Definition: ClusterMeltSegmenter.cpp:115
void cluster_segment(int *q, double **features, int frames_read, int feature_length, int nHMM_states, int histogram_length, int nclusters, int neighbour_limit)
void constq_segment(int *q, double **features, int frames_read, int bins, int ncoeff, int feature_type, int nHMM_states, int histogram_length, int nclusters, int neighbour_limit)
ClusterMeltSegmenter(ClusterMeltSegmenterParams params)
Definition: ClusterMeltSegmenter.cpp:30
void process(const double *FFTRe, const double *FFTIm, double *CQRe, double *CQIm)
Definition: ConstantQ.cpp:195
Definition: ConstantQ.h:31
Definition: segment.h:40
void forward(const double *realIn, double *realOut, double *imagOut)
Carry out a forward real-to-complex transform of size nsamples, where nsamples is the value provided ...
Definition: FFT.cpp:184
Definition: MFCC.h:23
void setFeatures(const std::vector< std::vector< double > > &f)
Definition: ClusterMeltSegmenter.cpp:319
Definition: segment.h:41
Definition: segment.h:43
Definition: Segmenter.h:23
Decimator carries out a fast downsample by a power-of-two factor.
Definition: Decimator.h:24
Definition: ConstantQ.h:23
virtual void initialise(int samplerate)
Definition: ClusterMeltSegmenter.cpp:50
void makeSegmentation(int *q, int len)
Definition: ClusterMeltSegmenter.cpp:380
virtual void extractFeatures(const double *samples, int nsamples)
Definition: ClusterMeltSegmenter.cpp:135
Generated by
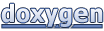