qm-dsp
1.8
|
ClusterMeltSegmenter.h
Go to the documentation of this file.
int neighbourhoodLimit
Definition: ClusterMeltSegmenter.h:107
std::vector< std::vector< double > > histograms
Definition: ClusterMeltSegmenter.h:86
int neighbourhoodLimit
Definition: ClusterMeltSegmenter.h:57
Definition: Segmenter.h:41
Definition: ConstantQ.h:31
Definition: ClusterMeltSegmenter.h:60
feature_types featureType
Definition: ClusterMeltSegmenter.h:47
Definition: segment.h:41
ClusterMeltSegmenterParams()
Definition: ClusterMeltSegmenter.h:35
Decimator carries out a fast downsample by a power-of-two factor.
Definition: Decimator.h:24
Generated by
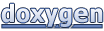