FFmpeg
|
life video source, based on John Conways' Life Game More...
#include "libavutil/file.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/lfg.h"
#include "libavutil/opt.h"
#include "libavutil/parseutils.h"
#include "libavutil/random_seed.h"
#include "libavutil/avstring.h"
#include "avfilter.h"
#include "internal.h"
#include "formats.h"
#include "video.h"
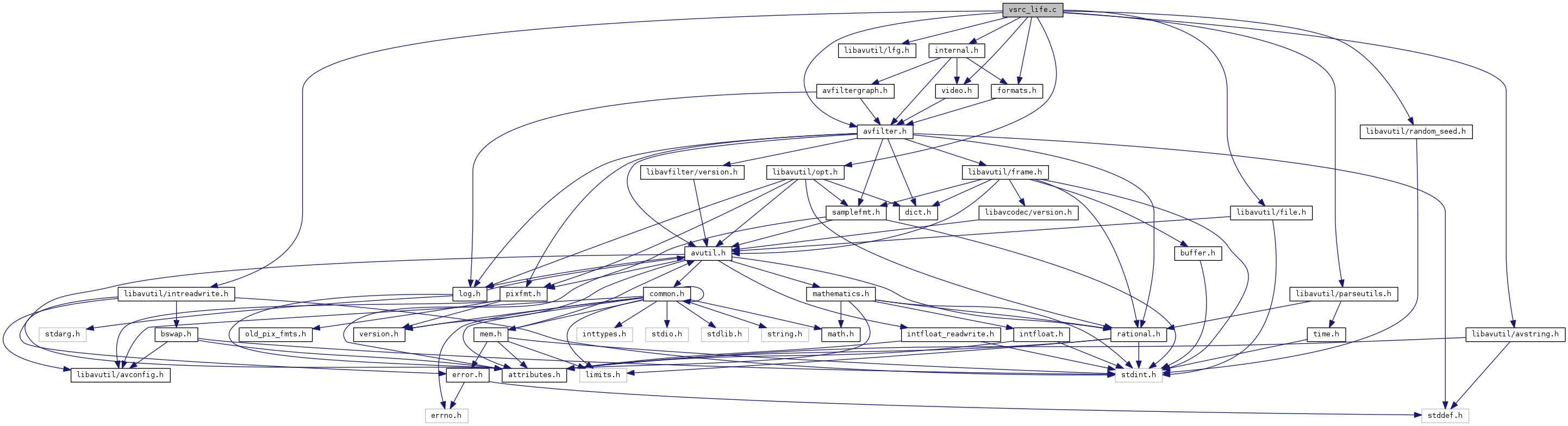
Go to the source code of this file.
Data Structures | |
struct | LifeContext |
Macros | |
#define | ALIVE_CELL 0xFF |
#define | OFFSET(x) offsetof(LifeContext, x) |
#define | FLAGS AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_FILTERING_PARAM |
#define | PARSE_COLOR(name) |
#define | FAST_DIV255(x) ((((x) + 128) * 257) >> 16) |
Functions | |
AVFILTER_DEFINE_CLASS (life) | |
static int | parse_rule (uint16_t *born_rule, uint16_t *stay_rule, const char *rule_str, void *log_ctx) |
static int | init_pattern_from_file (AVFilterContext *ctx) |
static int | init (AVFilterContext *ctx) |
static av_cold void | uninit (AVFilterContext *ctx) |
static int | config_props (AVFilterLink *outlink) |
static void | evolve (AVFilterContext *ctx) |
static void | fill_picture_monoblack (AVFilterContext *ctx, AVFrame *picref) |
static void | fill_picture_rgb (AVFilterContext *ctx, AVFrame *picref) |
static int | request_frame (AVFilterLink *outlink) |
static int | query_formats (AVFilterContext *ctx) |
Variables | |
static const AVOption | life_options [] |
static const AVFilterPad | life_outputs [] |
AVFilter | avfilter_vsrc_life |
Detailed Description
life video source, based on John Conways' Life Game
Definition in file vsrc_life.c.
Macro Definition Documentation
#define ALIVE_CELL 0xFF |
Definition at line 78 of file vsrc_life.c.
Referenced by evolve(), fill_picture_monoblack(), fill_picture_rgb(), init(), init_pattern_from_file(), and parse_rule().
Definition at line 384 of file vsrc_life.c.
Referenced by fill_picture_rgb().
#define FLAGS AV_OPT_FLAG_VIDEO_PARAM|AV_OPT_FLAG_FILTERING_PARAM |
Definition at line 80 of file vsrc_life.c.
#define OFFSET | ( | x | ) | offsetof(LifeContext, x) |
Definition at line 79 of file vsrc_life.c.
#define PARSE_COLOR | ( | name | ) |
Referenced by init().
Function Documentation
AVFILTER_DEFINE_CLASS | ( | life | ) |
|
static |
Definition at line 295 of file vsrc_life.c.
|
static |
Definition at line 306 of file vsrc_life.c.
Referenced by request_frame().
|
static |
Definition at line 361 of file vsrc_life.c.
Referenced by query_formats().
|
static |
Definition at line 386 of file vsrc_life.c.
Referenced by query_formats().
|
static |
Definition at line 223 of file vsrc_life.c.
|
static |
Definition at line 165 of file vsrc_life.c.
Referenced by init().
|
static |
Definition at line 104 of file vsrc_life.c.
Referenced by init().
|
static |
Definition at line 430 of file vsrc_life.c.
|
static |
Definition at line 413 of file vsrc_life.c.
|
static |
Definition at line 285 of file vsrc_life.c.
Variable Documentation
AVFilter avfilter_vsrc_life |
Definition at line 456 of file vsrc_life.c.
|
static |
Definition at line 82 of file vsrc_life.c.
|
static |
Definition at line 446 of file vsrc_life.c.
Generated on Wed May 8 2024 06:52:22 for FFmpeg by
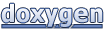