FFmpeg
|
vmnc.c File Reference
VMware Screen Codec (VMnc) decoder As Alex Beregszaszi discovered, this is effectively RFB data dump. More...
#include <stdio.h>
#include <stdlib.h>
#include "libavutil/common.h"
#include "libavutil/intreadwrite.h"
#include "avcodec.h"
#include "internal.h"
Include dependency graph for vmnc.c:
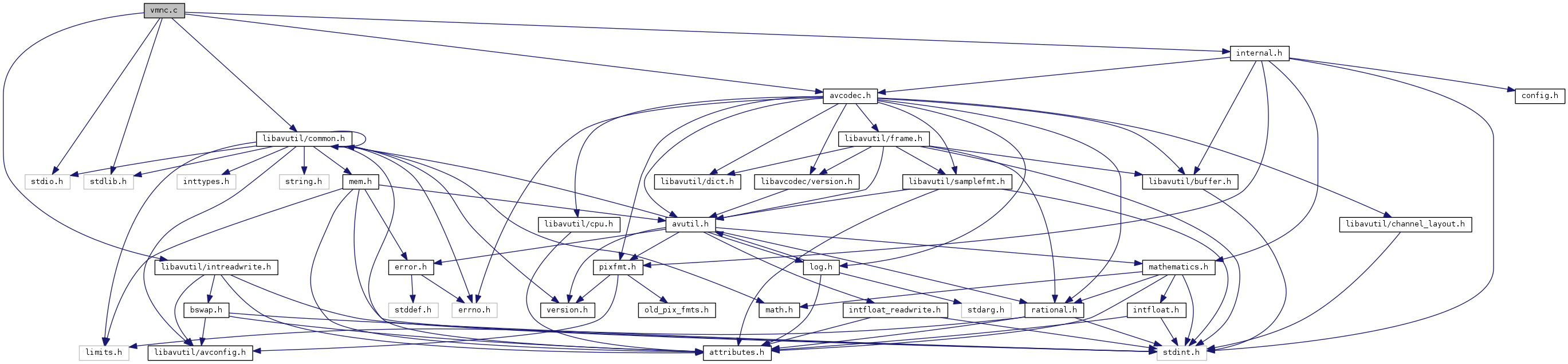
Go to the source code of this file.
Data Structures | |
struct | VmncContext |
Typedefs | |
typedef struct VmncContext | VmncContext |
Enumerations | |
enum | EncTypes { MAGIC_WMVd = 0x574D5664, MAGIC_WMVe, MAGIC_WMVf, MAGIC_WMVg, MAGIC_WMVh, MAGIC_WMVi, MAGIC_WMVj } |
enum | HexTile_Flags { HT_RAW = 1, HT_BKG = 2, HT_FG = 4, HT_SUB = 8, HT_CLR = 16 } |
Functions | |
static av_always_inline int | vmnc_get_pixel (const uint8_t *buf, int bpp, int be) |
static void | load_cursor (VmncContext *c, const uint8_t *src) |
static void | put_cursor (uint8_t *dst, int stride, VmncContext *c, int dx, int dy) |
static av_always_inline void | paint_rect (uint8_t *dst, int dx, int dy, int w, int h, int color, int bpp, int stride) |
static av_always_inline void | paint_raw (uint8_t *dst, int w, int h, const uint8_t *src, int bpp, int be, int stride) |
static int | decode_hextile (VmncContext *c, uint8_t *dst, const uint8_t *src, int ssize, int w, int h, int stride) |
static int | decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
static av_cold int | decode_init (AVCodecContext *avctx) |
static av_cold int | decode_end (AVCodecContext *avctx) |
Variables | |
AVCodec | ff_vmnc_decoder |
Detailed Description
VMware Screen Codec (VMnc) decoder As Alex Beregszaszi discovered, this is effectively RFB data dump.
Definition in file vmnc.c.
Typedef Documentation
typedef struct VmncContext VmncContext |
Enumeration Type Documentation
enum EncTypes |
enum HexTile_Flags |
Function Documentation
|
static |
|
static |
|
static |
Definition at line 229 of file vmnc.c.
Referenced by decode_frame().
|
static |
|
static |
Definition at line 88 of file vmnc.c.
Referenced by decode_frame().
|
static |
Definition at line 206 of file vmnc.c.
Referenced by decode_frame(), and decode_hextile().
|
static |
Definition at line 176 of file vmnc.c.
Referenced by decode_hextile().
|
static |
Definition at line 119 of file vmnc.c.
Referenced by decode_frame().
|
static |
Definition at line 76 of file vmnc.c.
Referenced by decode_hextile(), load_cursor(), and paint_raw().
Variable Documentation
AVCodec ff_vmnc_decoder |
Initial value:
= {
.name = "vmnc",
.type = AVMEDIA_TYPE_VIDEO,
.id = AV_CODEC_ID_VMNC,
.priv_data_size = sizeof(VmncContext),
.init = decode_init,
.close = decode_end,
.decode = decode_frame,
.capabilities = CODEC_CAP_DR1,
.long_name = NULL_IF_CONFIG_SMALL("VMware Screen Codec / VMware Video"),
}
struct VmncContext VmncContext
static int decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: vmnc.c:289
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: libavcodec/avcodec.h:192
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Generated on Wed Apr 16 2025 06:53:30 for FFmpeg by
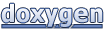