FFmpeg
|
Duck TrueMotion v1 Video Decoder by Alex Beregszaszi and Mike Melanson (melan) son@ pcisy s.ne tMore...
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "avcodec.h"
#include "internal.h"
#include "libavutil/imgutils.h"
#include "libavutil/internal.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/mem.h"
#include "truemotion1data.h"
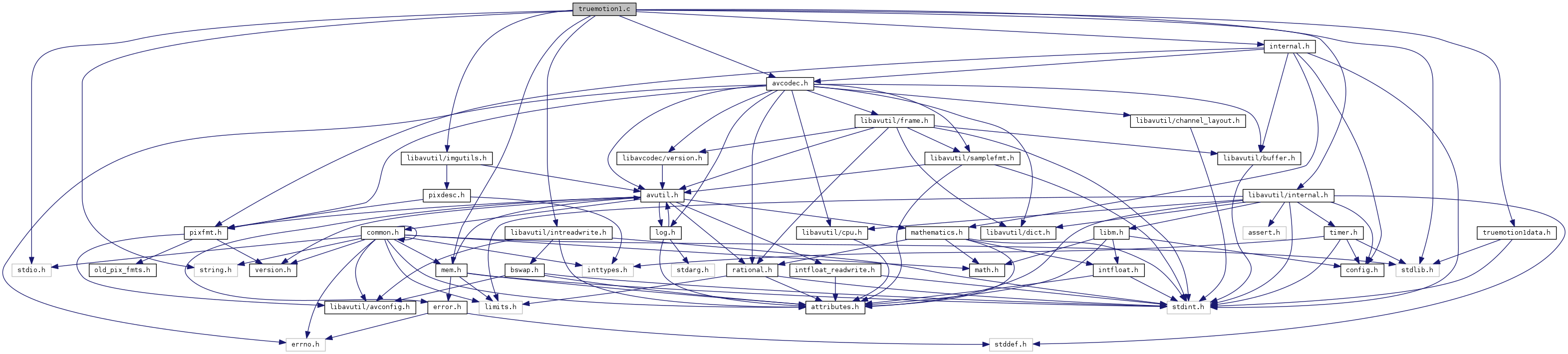
Go to the source code of this file.
Data Structures | |
struct | TrueMotion1Context |
struct | frame_header |
struct | comp_types |
Macros | |
#define | FLAG_SPRITE 32 |
#define | FLAG_KEYFRAME 16 |
#define | FLAG_INTERFRAME 8 |
#define | FLAG_INTERPOLATED 4 |
#define | ALGO_NOP 0 |
#define | ALGO_RGB16V 1 |
#define | ALGO_RGB16H 2 |
#define | ALGO_RGB24H 3 |
#define | BLOCK_2x2 0 |
#define | BLOCK_2x4 1 |
#define | BLOCK_4x2 2 |
#define | BLOCK_4x4 3 |
#define | GET_NEXT_INDEX() |
#define | APPLY_C_PREDICTOR() |
#define | APPLY_C_PREDICTOR_24() |
#define | APPLY_Y_PREDICTOR() |
#define | APPLY_Y_PREDICTOR_24() |
#define | OUTPUT_PIXEL_PAIR() |
Typedefs | |
typedef struct TrueMotion1Context | TrueMotion1Context |
typedef struct comp_types | comp_types |
Functions | |
static void | select_delta_tables (TrueMotion1Context *s, int delta_table_index) |
static int | make_ydt15_entry (int p1, int p2, int16_t *ydt) |
static int | make_cdt15_entry (int p1, int p2, int16_t *cdt) |
static int | make_ydt16_entry (int p1, int p2, int16_t *ydt) |
static int | make_cdt16_entry (int p1, int p2, int16_t *cdt) |
static int | make_ydt24_entry (int p1, int p2, int16_t *ydt) |
static int | make_cdt24_entry (int p1, int p2, int16_t *cdt) |
static void | gen_vector_table15 (TrueMotion1Context *s, const uint8_t *sel_vector_table) |
static void | gen_vector_table16 (TrueMotion1Context *s, const uint8_t *sel_vector_table) |
static void | gen_vector_table24 (TrueMotion1Context *s, const uint8_t *sel_vector_table) |
static int | truemotion1_decode_header (TrueMotion1Context *s) |
static av_cold int | truemotion1_decode_init (AVCodecContext *avctx) |
static void | truemotion1_decode_16bit (TrueMotion1Context *s) |
static void | truemotion1_decode_24bit (TrueMotion1Context *s) |
static int | truemotion1_decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
static av_cold int | truemotion1_decode_end (AVCodecContext *avctx) |
Variables | |
static const comp_types | compression_types [17] |
AVCodec | ff_truemotion1_decoder |
Detailed Description
Duck TrueMotion v1 Video Decoder by Alex Beregszaszi and Mike Melanson (melan) son@ pcisy s.ne t
The TrueMotion v1 decoder presently only decodes 16-bit TM1 data and outputs RGB555 (or RGB565) data. 24-bit TM1 data is not supported yet.
Definition in file truemotion1.c.
Macro Definition Documentation
#define ALGO_NOP 0 |
Definition at line 105 of file truemotion1.c.
Referenced by truemotion1_decode_frame().
#define ALGO_RGB16H 2 |
Definition at line 107 of file truemotion1.c.
#define ALGO_RGB16V 1 |
Definition at line 106 of file truemotion1.c.
#define ALGO_RGB24H 3 |
Definition at line 108 of file truemotion1.c.
Referenced by truemotion1_decode_frame(), and truemotion1_decode_header().
#define APPLY_C_PREDICTOR | ( | ) |
Definition at line 515 of file truemotion1.c.
Referenced by truemotion1_decode_16bit().
#define APPLY_C_PREDICTOR_24 | ( | ) |
Definition at line 536 of file truemotion1.c.
Referenced by truemotion1_decode_24bit().
#define APPLY_Y_PREDICTOR | ( | ) |
Definition at line 558 of file truemotion1.c.
Referenced by truemotion1_decode_16bit().
#define APPLY_Y_PREDICTOR_24 | ( | ) |
Definition at line 579 of file truemotion1.c.
Referenced by truemotion1_decode_24bit().
#define BLOCK_2x2 0 |
Definition at line 111 of file truemotion1.c.
Referenced by truemotion1_decode_16bit(), and truemotion1_decode_24bit().
#define BLOCK_2x4 1 |
Definition at line 112 of file truemotion1.c.
#define BLOCK_4x2 2 |
Definition at line 113 of file truemotion1.c.
Referenced by truemotion1_decode_16bit(), and truemotion1_decode_24bit().
#define BLOCK_4x4 3 |
Definition at line 114 of file truemotion1.c.
#define FLAG_INTERFRAME 8 |
Definition at line 84 of file truemotion1.c.
Referenced by truemotion1_decode_header().
#define FLAG_INTERPOLATED 4 |
Definition at line 85 of file truemotion1.c.
Referenced by truemotion1_decode_header().
#define FLAG_KEYFRAME 16 |
Definition at line 83 of file truemotion1.c.
Referenced by truemotion1_decode_16bit(), truemotion1_decode_24bit(), and truemotion1_decode_header().
#define FLAG_SPRITE 32 |
Definition at line 82 of file truemotion1.c.
Referenced by truemotion1_decode_header().
#define GET_NEXT_INDEX | ( | ) |
Definition at line 506 of file truemotion1.c.
Referenced by truemotion1_decode_16bit(), and truemotion1_decode_24bit().
#define OUTPUT_PIXEL_PAIR | ( | ) |
Definition at line 600 of file truemotion1.c.
Referenced by truemotion1_decode_16bit(), and truemotion1_decode_24bit().
Typedef Documentation
typedef struct comp_types comp_types |
typedef struct TrueMotion1Context TrueMotion1Context |
Function Documentation
|
static |
Definition at line 239 of file truemotion1.c.
Referenced by truemotion1_decode_header().
|
static |
Definition at line 260 of file truemotion1.c.
Referenced by truemotion1_decode_header().
|
static |
Definition at line 281 of file truemotion1.c.
Referenced by truemotion1_decode_header().
|
static |
Definition at line 186 of file truemotion1.c.
Referenced by gen_vector_table15().
|
static |
Definition at line 211 of file truemotion1.c.
Referenced by gen_vector_table16().
|
static |
Definition at line 230 of file truemotion1.c.
Referenced by gen_vector_table24().
|
static |
Definition at line 174 of file truemotion1.c.
Referenced by gen_vector_table15(), and select_delta_tables().
|
static |
Definition at line 199 of file truemotion1.c.
Referenced by gen_vector_table16(), and make_cdt15_entry().
|
static |
Definition at line 221 of file truemotion1.c.
Referenced by gen_vector_table24().
|
static |
Definition at line 148 of file truemotion1.c.
Referenced by truemotion1_decode_header().
|
static |
Definition at line 604 of file truemotion1.c.
Referenced by truemotion1_decode_frame().
|
static |
Definition at line 730 of file truemotion1.c.
Referenced by truemotion1_decode_frame().
|
static |
Definition at line 889 of file truemotion1.c.
|
static |
Definition at line 857 of file truemotion1.c.
|
static |
Definition at line 310 of file truemotion1.c.
Referenced by truemotion1_decode_frame().
|
static |
Definition at line 459 of file truemotion1.c.
Variable Documentation
|
static |
Definition at line 124 of file truemotion1.c.
AVCodec ff_truemotion1_decoder |
Definition at line 899 of file truemotion1.c.
Generated on Tue Mar 25 2025 06:54:37 for FFmpeg by
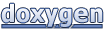