FFmpeg
|
#include "libavutil/avstring.h"
#include "libavutil/avassert.h"
#include "libavutil/bswap.h"
#include "libavutil/dict.h"
#include "libavutil/mathematics.h"
#include "libavutil/tree.h"
#include "avio_internal.h"
#include "nut.h"
#include "riff.h"
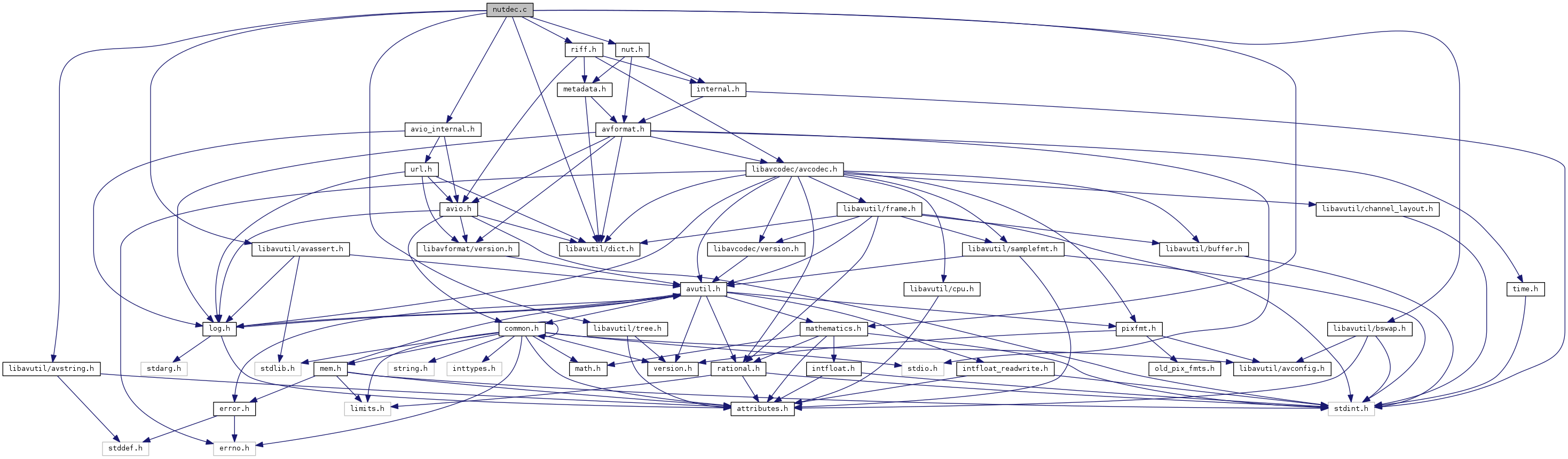
Go to the source code of this file.
Macros | |
#define | NUT_MAX_STREAMS 256 /* arbitrary sanity check value */ |
#define | GET_V(dst, check) |
Functions | |
static int64_t | nut_read_timestamp (AVFormatContext *s, int stream_index, int64_t *pos_arg, int64_t pos_limit) |
static int | get_str (AVIOContext *bc, char *string, unsigned int maxlen) |
static int64_t | get_s (AVIOContext *bc) |
static uint64_t | get_fourcc (AVIOContext *bc) |
static int | get_packetheader (NUTContext *nut, AVIOContext *bc, int calculate_checksum, uint64_t startcode) |
static uint64_t | find_any_startcode (AVIOContext *bc, int64_t pos) |
static int64_t | find_startcode (AVIOContext *bc, uint64_t code, int64_t pos) |
Find the given startcode. More... | |
static int | nut_probe (AVProbeData *p) |
static int | skip_reserved (AVIOContext *bc, int64_t pos) |
static int | decode_main_header (NUTContext *nut) |
static int | decode_stream_header (NUTContext *nut) |
static void | set_disposition_bits (AVFormatContext *avf, char *value, int stream_id) |
static int | decode_info_header (NUTContext *nut) |
static int | decode_syncpoint (NUTContext *nut, int64_t *ts, int64_t *back_ptr) |
static int64_t | find_duration (NUTContext *nut, int64_t filesize) |
static int | find_and_decode_index (NUTContext *nut) |
static int | nut_read_header (AVFormatContext *s) |
static int | decode_frame_header (NUTContext *nut, int64_t *pts, int *stream_id, uint8_t *header_idx, int frame_code) |
static int | decode_frame (NUTContext *nut, AVPacket *pkt, int frame_code) |
static int | nut_read_packet (AVFormatContext *s, AVPacket *pkt) |
static int | read_seek (AVFormatContext *s, int stream_index, int64_t pts, int flags) |
static int | nut_read_close (AVFormatContext *s) |
Variables | |
AVInputFormat | ff_nut_demuxer |
Macro Definition Documentation
Definition at line 194 of file nutdec.c.
Referenced by decode_frame_header(), decode_info_header(), decode_main_header(), decode_stream_header(), and find_and_decode_index().
Definition at line 33 of file nutdec.c.
Referenced by decode_main_header().
Function Documentation
|
static |
Definition at line 834 of file nutdec.c.
Referenced by nut_read_packet().
|
static |
Definition at line 760 of file nutdec.c.
Referenced by decode_frame().
|
static |
Definition at line 455 of file nutdec.c.
Referenced by nut_read_header(), and nut_read_packet().
|
static |
Definition at line 217 of file nutdec.c.
Referenced by nut_read_header().
|
static |
Definition at line 342 of file nutdec.c.
Referenced by nut_read_header().
|
static |
Definition at line 545 of file nutdec.c.
Referenced by nut_read_packet(), and nut_read_timestamp().
|
static |
Definition at line 596 of file nutdec.c.
Referenced by nut_read_header().
|
static |
Definition at line 138 of file nutdec.c.
Referenced by find_startcode(), nut_read_header(), and nut_read_packet().
|
static |
Definition at line 578 of file nutdec.c.
Referenced by find_and_decode_index().
|
static |
Find the given startcode.
- Parameters
-
code the startcode pos the start position of the search, or -1 if the current position
- Returns
- the position of the startcode or -1 if not found
Definition at line 169 of file nutdec.c.
Referenced by nut_read_header(), nut_read_timestamp(), and read_seek().
|
static |
Definition at line 68 of file nutdec.c.
Referenced by decode_stream_header().
|
static |
Definition at line 117 of file nutdec.c.
Referenced by decode_info_header(), decode_main_header(), decode_stream_header(), decode_syncpoint(), find_and_decode_index(), and nut_read_packet().
|
static |
Definition at line 58 of file nutdec.c.
Referenced by decode_frame_header(), decode_info_header(), decode_main_header(), and get_fourcc().
|
static |
Definition at line 38 of file nutdec.c.
Referenced by decode_info_header().
|
static |
|
static |
|
static |
|
static |
|
static |
Definition at line 934 of file nutdec.c.
Referenced by find_duration(), and read_seek().
|
static |
|
static |
Definition at line 440 of file nutdec.c.
Referenced by decode_info_header().
|
static |
Definition at line 204 of file nutdec.c.
Referenced by decode_info_header(), decode_main_header(), decode_stream_header(), decode_syncpoint(), and find_and_decode_index().
Variable Documentation
AVInputFormat ff_nut_demuxer |
Generated on Mon Nov 18 2024 06:52:08 for FFmpeg by
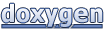