FFmpeg
|
libx264.c File Reference
#include "libavutil/internal.h"
#include "libavutil/opt.h"
#include "libavutil/mem.h"
#include "libavutil/pixdesc.h"
#include "avcodec.h"
#include "internal.h"
#include <x264.h>
#include <float.h>
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
Include dependency graph for libx264.c:
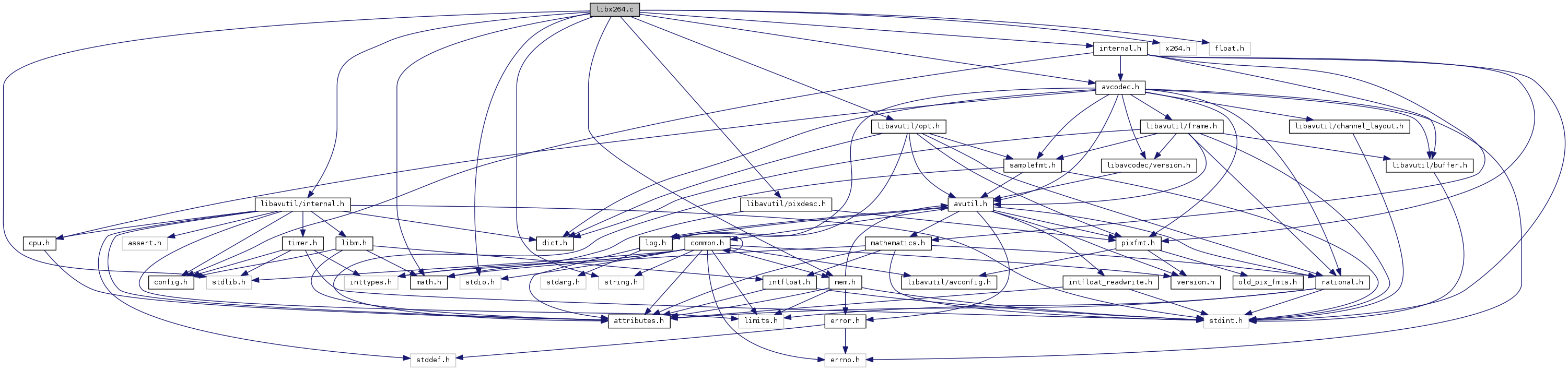
Go to the source code of this file.
Data Structures | |
struct | X264Context |
Macros | |
#define | OPT_STR(opt, param) |
#define | PARSE_X264_OPT(name, var) |
#define | OFFSET(x) offsetof(X264Context, x) |
#define | VE AV_OPT_FLAG_VIDEO_PARAM | AV_OPT_FLAG_ENCODING_PARAM |
Typedefs | |
typedef struct X264Context | X264Context |
Functions | |
static void | X264_log (void *p, int level, const char *fmt, va_list args) |
static int | encode_nals (AVCodecContext *ctx, AVPacket *pkt, x264_nal_t *nals, int nnal) |
static int | avfmt2_num_planes (int avfmt) |
static int | X264_frame (AVCodecContext *ctx, AVPacket *pkt, const AVFrame *frame, int *got_packet) |
static av_cold int | X264_close (AVCodecContext *avctx) |
static int | convert_pix_fmt (enum AVPixelFormat pix_fmt) |
static av_cold int | X264_init (AVCodecContext *avctx) |
static av_cold void | X264_init_static (AVCodec *codec) |
Variables | |
static enum AVPixelFormat | pix_fmts_8bit [] |
static enum AVPixelFormat | pix_fmts_9bit [] |
static enum AVPixelFormat | pix_fmts_10bit [] |
static enum AVPixelFormat | pix_fmts_8bit_rgb [] |
static const AVOption | options [] |
class { | |
class_name = "libx264" | |
item_name = av_default_item_name | |
option = options | |
version = LIBAVUTIL_VERSION_INT | |
}; | |
static const AVClass | rgbclass |
static const AVCodecDefault | x264_defaults [] |
AVCodec | ff_libx264_encoder |
AVCodec | ff_libx264rgb_encoder |
Macro Definition Documentation
#define OFFSET | ( | x | ) | offsetof(X264Context, x) |
#define OPT_STR | ( | opt, | |
param | |||
) |
Value:
do { \
av_log(avctx, AV_LOG_ERROR, \
"bad option '%s': '%s'\n", opt, param); \
av_log(avctx, AV_LOG_ERROR, \
"bad value for '%s': '%s'\n", opt, param); \
return -1; \
} \
} while (0)
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an it should directly call filter_frame on the corresponding output For a if there are queued frames already one of these frames should be pushed If the filter should request a frame on one of its repeatedly until at least one frame has been pushed Return it should return
Definition: filter_design.txt:216
Definition at line 237 of file libx264.c.
Referenced by X264_init().
#define PARSE_X264_OPT | ( | name, | |
var | |||
) |
Value:
}
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an it should directly call filter_frame on the corresponding output For a if there are queued frames already one of these frames should be pushed If the filter should request a frame on one of its repeatedly until at least one frame has been pushed Return it should return
Definition: filter_design.txt:216
Definition at line 274 of file libx264.c.
Referenced by X264_init().
#define VE AV_OPT_FLAG_VIDEO_PARAM | AV_OPT_FLAG_ENCODING_PARAM |
Typedef Documentation
typedef struct X264Context X264Context |
Function Documentation
|
static |
Definition at line 133 of file libx264.c.
Referenced by X264_frame().
|
static |
Definition at line 251 of file libx264.c.
Referenced by X264_init().
|
static |
Definition at line 95 of file libx264.c.
Referenced by X264_frame().
|
static |
|
static |
|
static |
Definition at line 79 of file libx264.c.
Referenced by X264_init().
Variable Documentation
const { ... } |
AVCodec ff_libx264_encoder |
Initial value:
= {
.name = "libx264",
.type = AVMEDIA_TYPE_VIDEO,
.id = AV_CODEC_ID_H264,
.priv_data_size = sizeof(X264Context),
.encode2 = X264_frame,
.close = X264_close,
.capabilities = CODEC_CAP_DELAY | CODEC_CAP_AUTO_THREADS,
.long_name = NULL_IF_CONFIG_SMALL("libx264 H.264 / AVC / MPEG-4 AVC / MPEG-4 part 10"),
.priv_class = &class,
.defaults = x264_defaults,
.init_static_data = X264_init_static,
}
Definition: libavcodec/avcodec.h:130
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
#define CODEC_CAP_AUTO_THREADS
Codec supports avctx->thread_count == 0 (auto).
Definition: libavcodec/avcodec.h:822
struct X264Context X264Context
Definition: avutil.h:143
static int X264_frame(AVCodecContext *ctx, AVPacket *pkt, const AVFrame *frame, int *got_packet)
Definition: libx264.c:152
AVCodec ff_libx264rgb_encoder |
Initial value:
= {
.name = "libx264rgb",
.type = AVMEDIA_TYPE_VIDEO,
.id = AV_CODEC_ID_H264,
.priv_data_size = sizeof(X264Context),
.encode2 = X264_frame,
.close = X264_close,
.capabilities = CODEC_CAP_DELAY,
.long_name = NULL_IF_CONFIG_SMALL("libx264 H.264 / AVC / MPEG-4 AVC / MPEG-4 part 10 RGB"),
.priv_class = &rgbclass,
.defaults = x264_defaults,
.pix_fmts = pix_fmts_8bit_rgb,
}
Definition: libavcodec/avcodec.h:130
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
struct X264Context X264Context
Definition: avutil.h:143
static int X264_frame(AVCodecContext *ctx, AVPacket *pkt, const AVFrame *frame, int *got_packet)
Definition: libx264.c:152
|
private |
|
static |
Initial value:
Definition at line 596 of file libx264.c.
Referenced by X264_init_static().
|
static |
Initial value:
= {
}
Definition: pixfmt.h:67
planar YUV 4:4:4, 24bpp, (1 Cr & Cb sample per 1x1 Y samples)
Definition: pixfmt.h:73
planar YUV 4:2:2, 16bpp, (1 Cr & Cb sample per 2x1 Y samples)
Definition: pixfmt.h:72
planar YUV 4:2:0, 12bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV420P and setting color_...
Definition: pixfmt.h:80
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
Definition at line 584 of file libx264.c.
Referenced by X264_init_static().
|
static |
Initial value:
|
static |
Initial value:
Definition at line 591 of file libx264.c.
Referenced by X264_init_static().
|
static |
Initial value:
= {
.class_name = "libx264rgb",
.item_name = av_default_item_name,
.option = options,
.version = LIBAVUTIL_VERSION_INT,
}
|
private |
|
static |
Initial value:
= {
{ "b", "0" },
{ "bf", "-1" },
{ "flags2", "0" },
{ "g", "-1" },
{ "i_qfactor", "-1" },
{ "qmin", "-1" },
{ "qmax", "-1" },
{ "qdiff", "-1" },
{ "qblur", "-1" },
{ "qcomp", "-1" },
{ "refs", "-1" },
{ "sc_threshold", "-1" },
{ "trellis", "-1" },
{ "nr", "-1" },
{ "me_range", "-1" },
{ "me_method", "-1" },
{ "subq", "-1" },
{ "b_strategy", "-1" },
{ "keyint_min", "-1" },
{ "coder", "-1" },
{ "cmp", "-1" },
{ "threads", AV_STRINGIFY(X264_THREADS_AUTO) },
{ "thread_type", "0" },
{ "flags", "+cgop" },
{ "rc_init_occupancy","-1" },
{ NULL },
}
Generated on Mon Nov 18 2024 06:52:07 for FFmpeg by
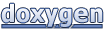