FFmpeg
|
JPEG 2000 encoder using libopenjpeg. More...
#include "libavutil/avassert.h"
#include "libavutil/common.h"
#include "libavutil/imgutils.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/opt.h"
#include "avcodec.h"
#include "internal.h"
#include <openjpeg.h>
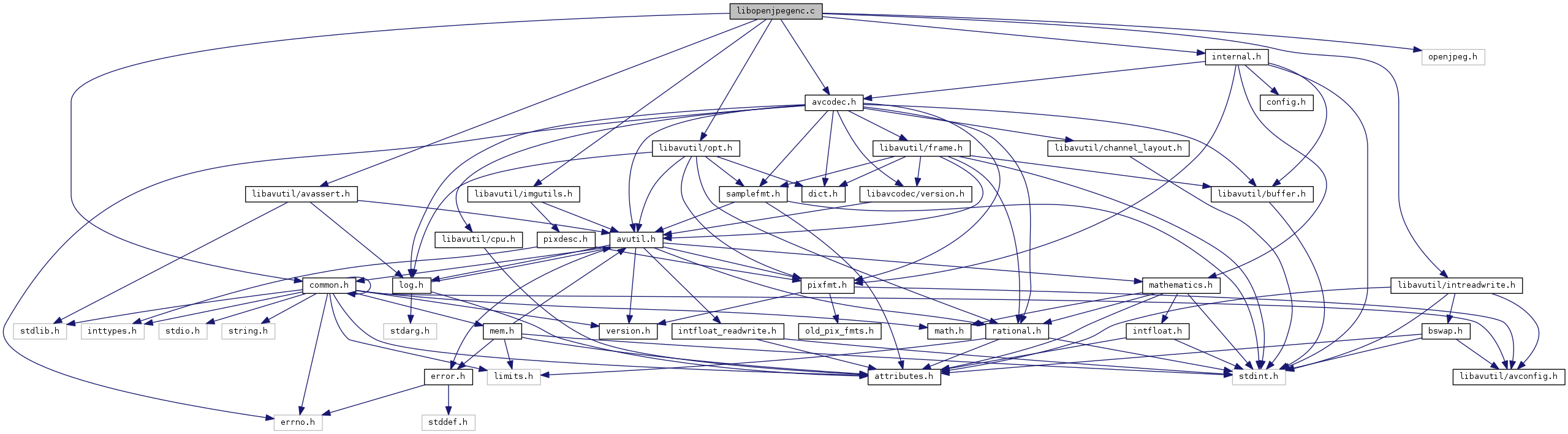
Go to the source code of this file.
Data Structures | |
struct | LibOpenJPEGContext |
Macros | |
#define | OPJ_STATIC |
#define | OFFSET(x) offsetof(LibOpenJPEGContext, x) |
#define | VE AV_OPT_FLAG_VIDEO_PARAM | AV_OPT_FLAG_ENCODING_PARAM |
Functions | |
static void | error_callback (const char *msg, void *data) |
static void | warning_callback (const char *msg, void *data) |
static void | info_callback (const char *msg, void *data) |
static opj_image_t * | mj2_create_image (AVCodecContext *avctx, opj_cparameters_t *parameters) |
static av_cold int | libopenjpeg_encode_init (AVCodecContext *avctx) |
static int | libopenjpeg_copy_packed8 (AVCodecContext *avctx, const AVFrame *frame, opj_image_t *image) |
static int | libopenjpeg_copy_packed16 (AVCodecContext *avctx, const AVFrame *frame, opj_image_t *image) |
static int | libopenjpeg_copy_unpacked8 (AVCodecContext *avctx, const AVFrame *frame, opj_image_t *image) |
static int | libopenjpeg_copy_unpacked16 (AVCodecContext *avctx, const AVFrame *frame, opj_image_t *image) |
static int | libopenjpeg_encode_frame (AVCodecContext *avctx, AVPacket *pkt, const AVFrame *frame, int *got_packet) |
static av_cold int | libopenjpeg_encode_close (AVCodecContext *avctx) |
Variables | |
static const AVOption | options [] |
class { | |
class_name = "libopenjpeg" | |
item_name = av_default_item_name | |
option = options | |
version = LIBAVUTIL_VERSION_INT | |
}; | |
AVCodec | ff_libopenjpeg_encoder |
Detailed Description
JPEG 2000 encoder using libopenjpeg.
Definition in file libopenjpegenc.c.
Macro Definition Documentation
#define OFFSET | ( | x | ) | offsetof(LibOpenJPEGContext, x) |
Definition at line 516 of file libopenjpegenc.c.
#define OPJ_STATIC |
Definition at line 27 of file libopenjpegenc.c.
#define VE AV_OPT_FLAG_VIDEO_PARAM | AV_OPT_FLAG_ENCODING_PARAM |
Definition at line 517 of file libopenjpegenc.c.
Function Documentation
Definition at line 60 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_init().
Definition at line 70 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_init().
|
static |
Definition at line 283 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_frame().
|
static |
Definition at line 253 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_frame().
|
static |
Definition at line 346 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_frame().
|
static |
Definition at line 314 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_frame().
|
static |
Definition at line 506 of file libopenjpegenc.c.
|
static |
Definition at line 380 of file libopenjpegenc.c.
|
static |
Definition at line 173 of file libopenjpegenc.c.
|
static |
Definition at line 75 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_init().
Definition at line 65 of file libopenjpegenc.c.
Referenced by libopenjpeg_encode_init().
Variable Documentation
const { ... } |
|
private |
Definition at line 546 of file libopenjpegenc.c.
AVCodec ff_libopenjpeg_encoder |
Definition at line 552 of file libopenjpegenc.c.
|
private |
Definition at line 547 of file libopenjpegenc.c.
|
private |
Definition at line 548 of file libopenjpegenc.c.
|
static |
Definition at line 518 of file libopenjpegenc.c.
|
private |
Definition at line 549 of file libopenjpegenc.c.
Generated on Tue Jan 21 2025 06:52:34 for FFmpeg by
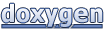