FFmpeg
|
Atrac 3 compatible decoder. More...
#include <math.h>
#include <stddef.h>
#include <stdio.h>
#include "libavutil/float_dsp.h"
#include "libavutil/libm.h"
#include "avcodec.h"
#include "bytestream.h"
#include "fft.h"
#include "fmtconvert.h"
#include "get_bits.h"
#include "internal.h"
#include "atrac.h"
#include "atrac3data.h"
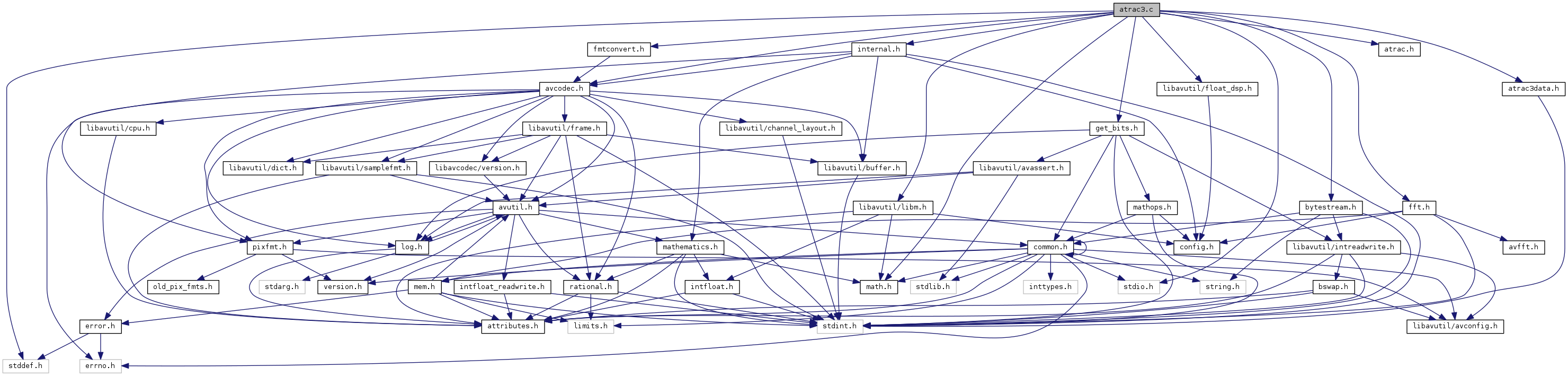
Go to the source code of this file.
Data Structures | |
struct | GainInfo |
struct | GainBlock |
struct | TonalComponent |
struct | ChannelUnit |
struct | ATRAC3Context |
Macros | |
#define | JOINT_STEREO 0x12 |
#define | STEREO 0x2 |
#define | SAMPLES_PER_FRAME 1024 |
#define | MDCT_SIZE 512 |
#define | INTERPOLATE(old, new, nsample) ((old) + (nsample) * 0.125 * ((new) - (old))) |
Typedefs | |
typedef struct GainInfo | GainInfo |
typedef struct GainBlock | GainBlock |
typedef struct TonalComponent | TonalComponent |
typedef struct ChannelUnit | ChannelUnit |
typedef struct ATRAC3Context | ATRAC3Context |
Functions | |
static | DECLARE_ALIGNED (32, float, mdct_window) |
static int | decode_bytes (const uint8_t *input, uint8_t *out, int bytes) |
static av_cold void | init_atrac3_window (void) |
static av_cold int | atrac3_decode_close (AVCodecContext *avctx) |
static void | read_quant_spectral_coeffs (GetBitContext *gb, int selector, int coding_flag, int *mantissas, int num_codes) |
Mantissa decoding. More... | |
static int | decode_spectrum (GetBitContext *gb, float *output) |
Restore the quantized band spectrum coefficients. More... | |
static int | decode_tonal_components (GetBitContext *gb, TonalComponent *components, int num_bands) |
Restore the quantized tonal components. More... | |
static int | decode_gain_control (GetBitContext *gb, GainBlock *block, int num_bands) |
Decode gain parameters for the coded bands. More... | |
static void | gain_compensate_and_overlap (float *input, float *prev, float *output, GainInfo *gain1, GainInfo *gain2) |
Apply gain parameters and perform the MDCT overlapping part. More... | |
static int | add_tonal_components (float *spectrum, int num_components, TonalComponent *components) |
Combine the tonal band spectrum and regular band spectrum. More... | |
static void | reverse_matrixing (float *su1, float *su2, int *prev_code, int *curr_code) |
static void | get_channel_weights (int index, int flag, float ch[2]) |
static void | channel_weighting (float *su1, float *su2, int *p3) |
static int | decode_channel_sound_unit (ATRAC3Context *q, GetBitContext *gb, ChannelUnit *snd, float *output, int channel_num, int coding_mode) |
Decode a Sound Unit. More... | |
static int | decode_frame (AVCodecContext *avctx, const uint8_t *databuf, float **out_samples) |
static int | atrac3_decode_frame (AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt) |
static void | atrac3_init_static_data (void) |
static av_cold int | atrac3_decode_init (AVCodecContext *avctx) |
Variables | |
AVCodec | ff_atrac3_decoder |
Detailed Description
Atrac 3 compatible decoder.
This decoder handles Sony's ATRAC3 data.
Container formats used to store atrac 3 data: RealMedia (.rm), RIFF WAV (.wav, .at3), Sony OpenMG (.oma, .aa3).
To use this decoder, a calling application must supply the extradata bytes provided in the containers above.
Definition in file atrac3.c.
Macro Definition Documentation
#define INTERPOLATE | ( | old, | |
new, | |||
nsample | |||
) | ((old) + (nsample) * 0.125 * ((new) - (old))) |
Definition at line 529 of file atrac3.c.
Referenced by channel_weighting(), and reverse_matrixing().
#define JOINT_STEREO 0x12 |
Definition at line 51 of file atrac3.c.
Referenced by atrac3_decode_init(), decode_channel_sound_unit(), decode_frame(), and mp3lame_encode_init().
#define MDCT_SIZE 512 |
Definition at line 55 of file atrac3.c.
Referenced by DECLARE_ALIGNED().
#define SAMPLES_PER_FRAME 1024 |
Definition at line 54 of file atrac3.c.
Referenced by atrac3_decode_frame(), atrac3_decode_init(), decode_spectrum(), and decode_tonal_components().
#define STEREO 0x2 |
Definition at line 52 of file atrac3.c.
Referenced by atrac3_decode_init(), libshine_encode_init(), and mp3lame_encode_init().
Typedef Documentation
typedef struct ATRAC3Context ATRAC3Context |
typedef struct ChannelUnit ChannelUnit |
typedef struct TonalComponent TonalComponent |
Function Documentation
|
static |
Combine the tonal band spectrum and regular band spectrum.
- Parameters
-
spectrum output spectrum buffer num_components number of tonal components components tonal components for this band
- Returns
- position of the last tonal coefficient
Definition at line 511 of file atrac3.c.
Referenced by decode_channel_sound_unit().
|
static |
Definition at line 196 of file atrac3.c.
Referenced by atrac3_decode_init().
|
static |
|
static |
Definition at line 841 of file atrac3.c.
Referenced by atrac3_decode_init().
|
static |
Definition at line 607 of file atrac3.c.
Referenced by decode_frame().
|
static |
Reverse the odd bands before IMDCT, this is an effect of the QMF transform or it gives better compression to do it this way. FIXME: It should be possible to handle this in imdct_calc for that to happen a modification of the prerotation step of all SIMD code and C code is needed. Or fix the functions before so they generate a pre reversed spectrum.
Definition at line 158 of file atrac3.c.
Referenced by atrac3_decode_frame().
|
static |
Decode a Sound Unit.
- Parameters
-
snd the channel unit to be used output the decoded samples before IQMF in float representation channel_num channel number coding_mode the coding mode (JOINT_STEREO or regular stereo/mono)
Definition at line 638 of file atrac3.c.
Referenced by decode_frame().
|
static |
Definition at line 706 of file atrac3.c.
Referenced by atrac3_decode_frame().
|
static |
Decode gain parameters for the coded bands.
- Parameters
-
block the gainblock for the current band num_bands amount of coded bands
Definition at line 417 of file atrac3.c.
Referenced by decode_channel_sound_unit().
|
static |
Restore the quantized band spectrum coefficients.
- Returns
- subband count, fix for broken specification/files
Definition at line 275 of file atrac3.c.
Referenced by decode_channel_sound_unit().
|
static |
Restore the quantized tonal components.
- Parameters
-
components tonal components num_bands number of coded bands
Definition at line 333 of file atrac3.c.
Referenced by decode_channel_sound_unit().
|
static |
Apply gain parameters and perform the MDCT overlapping part.
- Parameters
-
input input buffer prev previous buffer to perform overlap against output output buffer gain1 current band gain info gain2 next band gain info
Definition at line 455 of file atrac3.c.
Referenced by decode_channel_sound_unit().
|
static |
Definition at line 594 of file atrac3.c.
Referenced by channel_weighting().
Definition at line 181 of file atrac3.c.
Referenced by atrac3_init_static_data().
|
static |
Mantissa decoding.
- Parameters
-
selector which table the output values are coded with coding_flag constant length coding or variable length coding mantissas mantissa output table num_codes number of values to get
Definition at line 216 of file atrac3.c.
Referenced by decode_spectrum(), and decode_tonal_components().
|
static |
Definition at line 532 of file atrac3.c.
Referenced by decode_frame().
Variable Documentation
AVCodec ff_atrac3_decoder |
Generated on Wed May 8 2024 06:52:15 for FFmpeg by
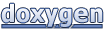