FFmpeg
|
ac3enc_fixed.c
Go to the documentation of this file.
static av_cold int ac3_fixed_encode_init(AVCodecContext *avctx)
Definition: ac3enc_fixed.c:147
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Definition: samplefmt.h:50
av_cold int AC3_NAME() mdct_init(AC3EncodeContext *s)
Initialize MDCT tables.
Definition: ac3enc_fixed.c:65
av_cold int ff_ac3_encode_close(AVCodecContext *avctx)
Finalize encoding and free any memory allocated by the encoder.
Definition: ac3enc.c:2015
av_cold void AC3_NAME() mdct_end(AC3EncodeContext *s)
Finalize MDCT and free allocated memory.
Definition: ac3enc_fixed.c:53
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an input
Definition: filter_design.txt:216
Definition: avutil.h:144
overlapping window(triangular window to avoid too much overlapping) ovidx
int(* ac3_max_msb_abs_int16)(const int16_t *src, int len)
Calculate the maximum MSB of the absolute value of each element in an array of int16_t.
Definition: ac3dsp.h:54
void(* apply_window_int16)(int16_t *output, const int16_t *input, const int16_t *window, unsigned int len)
Apply symmetric window in 16-bit fixed-point.
Definition: dsputil.h:294
void(* ac3_lshift_int16)(int16_t *src, unsigned int len, unsigned int shift)
Left-shift each value in an array of int16_t by a specified amount.
Definition: ac3dsp.h:65
void(* ac3_rshift_int32)(int32_t *src, unsigned int len, unsigned int shift)
Right-shift each value in an array of int32_t by a specified amount.
Definition: ac3dsp.h:76
void(* sum_square_butterfly_int32)(int64_t sum[4], const int32_t *coef0, const int32_t *coef1, int len)
Definition: ac3dsp.h:129
void(* vector_clip_int32)(int32_t *dst, const int32_t *src, int32_t min, int32_t max, unsigned int len)
Clip each element in an array of int32_t to a given minimum and maximum value.
Definition: dsputil.h:310
static CoefType calc_cpl_coord(CoefSumType energy_ch, CoefSumType energy_cpl)
Definition: ac3enc_fixed.c:134
Definition: libavcodec/avcodec.h:384
int ff_ac3_fixed_encode_frame(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
static void apply_window(void *dsp, int16_t *output, const int16_t *input, const int16_t *window, unsigned int len)
Definition: ac3enc_fixed.c:76
static void clip_coefficients(DSPContext *dsp, int32_t *coef, unsigned int len)
Definition: ac3enc_fixed.c:125
AC-3 encoder float/fixed template.
common internal api header.
const int16_t ff_ac3_window[AC3_WINDOW_SIZE/2]
these buffered frames must be flushed immediately if a new input produces new output(Example:frame rate-doubling filter:filter_frame must(1) flush the second copy of the previous frame, if it is still there,(2) push the first copy of the incoming frame,(3) keep the second copy for later.) If the input frame is not enough to produce output
AC-3 encoder & E-AC-3 encoder common header.
static void sum_square_butterfly(AC3EncodeContext *s, int64_t sum[4], const int32_t *coef0, const int32_t *coef1, int len)
Definition: ac3enc_fixed.c:115
E-AC-3 encoder.
Generated on Wed May 7 2025 06:52:37 for FFmpeg by
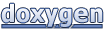