x
|
#include <string.h>
#include "arrayalloc.h"
#include "align8.h"
#include "fft.h"
#include "quickspec.h"
#include "tstream.h"
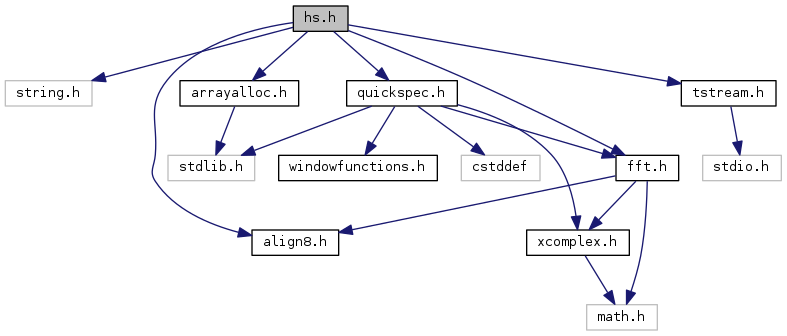
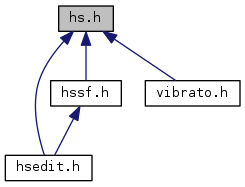
Go to the source code of this file.
Data Structures | |
struct | atom |
struct | candid |
struct | dsparams1 |
struct | NMResults |
struct | NMSettings |
class | stiffcandid |
class | THS |
class | TPolygon |
class | TTempAtom |
Macros | |
#define | ATOM_LOCALANCHOR 1 |
#define | HS_CONSTF 1 |
#define | MAX_CAND 64 |
#define | STIFF_B_MAX 0.01 |
Enumerations | |
enum | atomtype { atAnchor, atPeak, atInfered, atMuted, atBuried } |
Functions | |
double | ds0 (double, void *) |
void | areaandcentroid (double &A, double &cx, double &cy, int N, double *x, double *y) |
void | cutcvpoly (int &N, double *x, double *y, double A, double B, double C, bool protect=false) |
double | maximalminimum (double &x, double &y, int N, double *sx, double *sy) |
void | CutR (TPolygon *R, int apind, double af, double ef, bool protect=false) |
void | ExBStiff (double &Bmin, double &Bmax, int N, double *F, double *G) |
void | ExFmStiff (double &Fmin, double &Fmax, int m, int N, double *F, double *G) |
void | ExtendR (TPolygon *R, double delf1, double delf2, double minf) |
void | InitializeR (TPolygon *R, double af, double ef, double maxB) |
void | InitializeR (TPolygon *R, int apind, double af, double ef, double maxB) |
void | AtomsToPartials (int k, atom *part, int &M, int &Fr, atom **&partials, int offst) |
int | NMResultToAtoms (int M, atom *HP, int t, int wid, NMResults results) |
int | NMResultToPartials (int M, int fr, atom **Partials, int t, int wid, NMResults results) |
double | PeakShapeC (double f, int Fr, int N, cdouble **x, int B, int M, double *c, double iH2) |
double | PeakShapeC (double f, int Fr, int N, cfloat **x, int B, int M, double *c, double iH2) |
int | QuickPeaks (double *f, double *a, int N, cdouble *x, int M, double *c, double iH2, double mina, int binst=-1, int binen=-1, int B=5, double *rsr=0) |
int | QuickPeaks (double *f, double *a, int Fr, int N, cdouble **x, int fr0, int r0, int M, double *c, double iH2, double mina, int binst=-1, int binen=-1, int B=5, double *rsr=0) |
double | NoteMatchStiff3 (TPolygon *R, double &f0, double &B, int pc, double *fps, double *vps, int Fr, cdouble **x, int N, int offst, NMSettings *settings, NMResults *results, int lastp, double *lastvfp, double(*computes)(double a, void *params)=ds0, int forceinputlocalfr=-1) |
double | NoteMatchStiff3 (TPolygon *R, double &f0, double &B, int pc, double *fps, double *vps, double *rsr, int Fr, cdouble **x, int N, int offst, NMSettings *settings, NMResults *results, int lastp, double *lastvfp, double(*computes)(double a, void *params)=ds0, int forceinputlocalfr=-1) |
double | NoteMatchStiff3 (TPolygon *R, double &f0, double &B, int Fr, cdouble **x, int N, int offst, NMSettings *settings, NMResults *results, int lastp, double *lastvfp, double(*deltas)(double a, void *params)=ds0, bool forceinputlocalfr=false, int startfr=-1, int validfrrange=0) |
double | NoteMatchStiff3 (TPolygon *R, int peak0, int pin0, cdouble *x, int pc, double *fps, double *vps, int N, NMSettings *settings, double *vfp, int **pitchind, int newpc) |
int | FindNote (int _t, double _f, int &M, int &Fr, atom **&partials, int frst, int fren, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings) |
int | FindNoteConst (int _t, double _f, int &M, int &Fr, atom **&partials, int frst, int fren, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings, double brake) |
int | FindNoteF (atom *part, double &starts, TPolygon *R, int startp, double *startvfp, int frst, int fren, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings, double brake) |
int | FindNoteFB (int frst, TPolygon *Rst, double *vfpst, int fren, TPolygon *Ren, double *vfpen, int M, atom **partials, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings) |
void | NoteMatchStiff3FB (int &pitchcount, TPolygon **&R, double **&vfp, double *&sc, int *newpitches, int *prev, int pc, double *fps, double *vps, cdouble *x, int wid, int maxpitch, NMSettings *settings) |
double * | SynthesisHS (int pm, int pfr, atom **partials, int &dst, int &den, bool *terminatetag=0) |
double * | SynthesisHS (THS *HS, int &dst, int &den, bool *terminatetag=0) |
double * | SynthesisHS2 (int M, int Fr, atom **partials, int &dst, int &den, bool *terminatetag=0) |
double * | SynthesisHSp (int pm, int pfr, atom **partials, int &dst, int &den, double **startamp=0, int st_start=0, int st_offst=0, int st_count=0) |
double * | SynthesisHSp (THS *HS, int &dst, int &den) |
void | ReEstHS1 (THS *HS, __int16 *Data16) |
Detailed Description
- harmonic sinusoid model
Further reading: Wen X. and M. Sandler, "Sinusoid modeling in a harmonic context," in Proc. DAFx'07, Bordeaux, 2007.
Function Documentation
void areaandcentroid | ( | double & | A, |
double & | cx, | ||
double & | cy, | ||
int | N, | ||
double * | x, | ||
double * | y | ||
) |
function areaandcentroid: calculates the area and centroid of a convex polygon.
In: x[N], y[N]: x- and y-coordinates of vertices of a polygon Out: A: area (cx, cy): coordinate of the centroid
No return value.
function AtomsToPartials: sort a list of atoms as a number of partials. It is assumed that the atoms are simply those from a harmonic sinusoid in arbitrary order with uniform timing and partial index clearly marked, so that it is easy to sort them into a list of partials. However, the sorting process does not check for harmonicity, missing or duplicate atoms, uniformity of timing, etc.
In: part[k]: a list of atoms offst: interval between adjacent measurement points (hop size) Out: M, Fr, partials[M][Fr]: a list of partials containing the atoms.
No return value. partials[][] is allocated anew and must be freed by caller.
void cutcvpoly | ( | int & | N, |
double * | x, | ||
double * | y, | ||
double | A, | ||
double | B, | ||
double | C, | ||
bool | protect | ||
) |
function cutcvpoly: severs a polygon by a straight line
In: x[N], y[N]: x- and y-coordinates of vertices of a polygon, starting from the leftmost (x[0]= min x[n]) point clockwise; in case of two leftmost points, x[0] is the upper one and x[N-1] is the lower one. A, B, C: coefficients of a straight line Ax+By+C=0. protect: specifies what to do if the whole polygon satisfy Ax+By+C>0, true=do noting, false=eliminate. Out: N, x[N], y[N]: the polygon severed by the line, retaining that half for which Ax+By+C<=0.
No return value.
double ds0 | ( | double | a, |
void * | s | ||
) |
function ds0: atom score 0 - energy
In: a: atom amplitude
Returns a^2, or s[0]+a^2 is s is not NULL, as atom score.
void ExBStiff | ( | double & | Bmin, |
double & | Bmax, | ||
int | N, | ||
double * | F, | ||
double * | G | ||
) |
function ExBStiff: finds the minimal and maximal values of stiffness coefficient B given a F-G polygon
In: F[N], G[N]: vertices of a F-G polygon Out: Bmin, Bmax: minimal and maximal values of the stiffness coefficient
No reutrn value.
void ExFmStiff | ( | double & | Fmin, |
double & | Fmax, | ||
int | m, | ||
int | N, | ||
double * | F, | ||
double * | G | ||
) |
function ExFmStiff: finds the minimal and maximal frequecies of partial m given a F-G polygon
In: F[N], G[N]: vertices of a F-G polygon m: partial index, fundamental=1 Out: Fmin, Fmax: minimal and maximal frequencies of partial m
No return value.
void ExtendR | ( | TPolygon * | R, |
double | delp1, | ||
double | delp2, | ||
double | minf | ||
) |
function ExtendR: extends a F-G polygon on the f axis (to allow a larger pitch range)
In: R: the F-G polygon delp1: amount of extension to the low end, in semitones delpe: amount of extension to the high end, in semitones minf: minimal fundamental frequency Out: R: the extended F-G polygon
No return value.
int FindNote | ( | int | _t, |
double | _f, | ||
int & | M, | ||
int & | Fr, | ||
atom **& | partials, | ||
int | frst, | ||
int | fren, | ||
int | wid, | ||
int | offst, | ||
TQuickSpectrogram * | Spec, | ||
NMSettings | settings | ||
) |
function FindNote: harmonic sinusoid tracking from a starting point in time-frequency plane forward and backward.
In: _t, _f: start time and frequency frst, fren: tracking range, in frames Spec: spectrogram wid, offst: atom scale and hop size, must be consistent with Spec settings: note match settings brake: tracking termination threshold Out: M, Fr, partials[M][Fr]: HS partials
Returns 0 if tracking is done by FindNoteF(), 1 if tracking is done by FindNoteConst()
int FindNoteConst | ( | int | _t, |
double | _f, | ||
int & | M, | ||
int & | Fr, | ||
atom **& | partials, | ||
int | frst, | ||
int | fren, | ||
int | wid, | ||
int | offst, | ||
TQuickSpectrogram * | Spec, | ||
NMSettings | settings, | ||
double | brake | ||
) |
function FindNoteConst: constant-pitch harmonic sinusoid tracking
In: _t, _f: start time and frequency frst, fren: tracking range, in frames Spec: spectrogram wid, offst: atom scale and hop size, must be consistent with Spec settings: note match settings brake: tracking termination threshold Out: M, Fr, partials[M][Fr]: HS partials
Returns 1.
int FindNoteF | ( | atom * | part, |
double & | starts, | ||
TPolygon * | R, | ||
int | startp, | ||
double * | startvfp, | ||
int | frst, | ||
int | fren, | ||
int | wid, | ||
int | offst, | ||
TQuickSpectrogram * | Spec, | ||
NMSettings | settings, | ||
double | brake | ||
) |
function FindNoteF: forward harmonic sinusoid tracking starting from a given harmonic atom until an endpoint is detected or a search boundary is reached.
In: starts: harmonic atom score of the start HA startvfp[startp]: amplitudes of partials of start HA R: F-G polygon of the start HA frst, frst: frame index of the start and end frames of tracking. Spec: spectrogram wid, offst: atom scale and hop size, must be consistent with Spec settings: note match settings brake: tracking termination threshold. Out: part[return value]: list of atoms tracked. starts: maximal HA score of tracked frames. Its product with brake is used as the tracking threshold.
Returns the number of atoms found.
int FindNoteFB | ( | int | frst, |
TPolygon * | Rst, | ||
double * | vfpst, | ||
int | fren, | ||
TPolygon * | Ren, | ||
double * | vfpen, | ||
int | M, | ||
atom ** | partials, | ||
int | wid, | ||
int | offst, | ||
TQuickSpectrogram * | Spec, | ||
NMSettings | settings | ||
) |
function FindNoteFB: forward-backward harmonic sinusid tracking.
In: frst, fren: index of start and end frames Rst, Ren: F-G polygons of start and end harmonic atoms vfpst[M], vfpen[M]: amplitude vectors of start and end harmonic atoms Spec: spectrogram wid, offst: atom scale and hop size, must be consistent with Spec settings: note match settings Out: partials[0:fren-frst][M], hosting tracked HS between frst and fren (inc).
Returns 0 if successful, 1 if failure in pitch tracking stage (no feasible HA candidate at some frame). On start partials[0:fren-frst][M] shall be prepared to receive fren-frst+1 harmonic atoms
void InitializeR | ( | TPolygon * | R, |
double | af, | ||
double | ef, | ||
double | maxB | ||
) |
function InitailizeR: initializes a F-G polygon with a fundamental frequency range and stiffness coefficient bound
In: af, ef: centre and half width of the fundamental frequency range maxB: maximal value of stiffness coefficient (the minimal is set to 0) Out: R: the initialized F-G polygon.
No reutrn value.
void InitializeR | ( | TPolygon * | R, |
int | apind, | ||
double | af, | ||
double | ef, | ||
double | maxB | ||
) |
function InitialzeR: initializes a F-G polygon with a frequency range for a given partial and stiffness coefficient bound
In: apind: partial index af, ef: centre and half width of the frequency range of the apind-th partial maxB; maximal value of stiffness coefficient (the minimal is set to 0) Out: R: the initialized F-G polygon.
No return value.
double maximalminimum | ( | double & | x, |
double & | y, | ||
int | N, | ||
double * | sx, | ||
double * | sy | ||
) |
function maximalminimum: finds the point within a polygon that maximizes its minimal distance to the sides.
In: sx[N], sy[N]: x- and y-coordinates of vertices of a polygon Out: (x, y): point within the polygon with maximal minimal distance to the sides
Returns the maximial minimal distance. A circle centred at (x, y) with the return value as the radius is the maximum inscribed circle of the polygon.
function NMResultToAtoms: converts the note-match result hosted in a NMResults structure, i.e. parameters of a harmonic atom, to a list of atoms. The process retrieves atom parameters from low partials until a required number of atoms have been retrieved or a non-positive frequency is encountered (non-positive frequencies are returned by note-match to indicate high partials for which no informative estimates can be obtained).
In: results: the NMResults structure hosting the harmonic atom M: number of atoms to request from &results t: time of this harmonic atom wid: scale of this harmonic atom with which the note-match was performed Out: HP[return value]: list of atoms retrieved from $result.
Returns the number of atoms retrieved.
function NMResultToPartials: reads atoms of a harmonic atom in a NMResult structure into HS partials.
In: results: the NMResults structure hosting the harmonic atom M: number of atoms to request from &results fr: frame index of this harmonic atom t: time of this harmonic atom wid: scale of this harmonic atom with which the note-match was performed Out: Partials[M][]: HS partials whose fr-th frame is updated to the harmonic partial read from $results
Returns the number of partials retrieved.
double NoteMatchStiff3 | ( | TPolygon * | R, |
double & | f0, | ||
double & | B, | ||
int | pc, | ||
double * | fps, | ||
double * | vps, | ||
int | Fr, | ||
cdouble ** | x, | ||
int | N, | ||
int | offst, | ||
NMSettings * | settings, | ||
NMResults * | results, | ||
int | lastp, | ||
double * | lastvfp, | ||
double(*)(double a, void *params) | computes, | ||
int | forceinputlocalfr | ||
) |
function NoteMatchStiff3: finds harmonic atom from spectrum if Fr=1, or constant-pitch harmonic sinusoid from spectrogram if Fr>1.
In: x[Fr][N/2+1]: spectrogram fps[pc], vps[pc]: primitive (rough) peak frequencies and amplitudes N, offst: atom scale and hop size R: initial F-G polygon constraint, optional settings: note match settings computes: pointer to a function that computes HA score, must be ds0 or ds1 lastvfp[lastp]: amplitude of the previous harmonic atom forceinputlocalfr: specifies if partial settings->pin0 is taken for granted ("pinned") Out: results: note match results f0, B: fundamental frequency and stiffness coefficient R: F-G polygon of harmonic atom
Returns the total energy of HA or constant-pitch HS.
double NoteMatchStiff3 | ( | TPolygon * | R, |
double & | f0, | ||
double & | B, | ||
int | pc, | ||
double * | fps, | ||
double * | vps, | ||
double * | rsr, | ||
int | Fr, | ||
cdouble ** | x, | ||
int | N, | ||
int | offst, | ||
NMSettings * | settings, | ||
NMResults * | results, | ||
int | lastp, | ||
double * | lastvfp, | ||
double(*)(double a, void *params) | computes, | ||
int | forceinputlocalfr | ||
) |
function NoteMatchStiff3: finds harmonic atom from spectrum if Fr=1, or constant-pitch harmonic sinusoid from spectrogram if Fr>1. This version uses residue-sinusoid ratio ("rsr") that measures how "good" a spectral peak is in terms of its shape. peaks with large rsr[] is likely to be contaminated and their frequency estimates are regarded unreliable.
In: x[Fr][N/2+1]: spectrogram N, offst: atom scale and hop size fps[pc], vps[pc], rsr[pc]: primitive (rough) peak frequencies, amplitudes and shape factor R: initial F-G polygon constraint, optional settings: note match settings computes: pointer to a function that computes HA score, must be ds0 or ds1 lastvfp[lastp]: amplitude of the previous harmonic atom forceinputlocalfr: specifies if partial settings->pin0 is taken for granted ("pinned") Out: results: note match results f0, B: fundamental frequency and stiffness coefficient R: F-G polygon of harmonic atom
Returns the total energy of HA or constant-pitch HS.
double NoteMatchStiff3 | ( | TPolygon * | R, |
double & | f0, | ||
double & | B, | ||
int | Fr, | ||
cdouble ** | x, | ||
int | N, | ||
int | offst, | ||
NMSettings * | settings, | ||
NMResults * | results, | ||
int | lastp, | ||
double * | lastvfp, | ||
double(*)(double a, void *params) | computes, | ||
bool | forceinputlocalfr, | ||
int | startfr, | ||
int | validfrrange | ||
) |
function NoteMatchStiff3: wrapper function of the above that does peak picking and HA grouping (or constant-pitch HS finding) in a single call.
In: x[Fr][N/2+1]: spectrogram N, offst: atom scale and hop size R: initial F-G polygon constraint, optional startfr, validfrrange: centre and half width, in frames, of the interval used for peak picking if Fr>1 settings: note match settings computes: pointer to a function that computes HA score, must be ds0 or ds1 lastvfp[lastp]: amplitude of the previous harmonic atom forceinputlocalfr: specifies if partial settings->pin0 is taken for granted ("pinned") Out: results: note match results f0, B: fundamental frequency and stiffness coefficient R: F-G polygon of harmonic atom
Returns the total energy of HA or constant-pitch HS.
double NoteMatchStiff3 | ( | TPolygon * | R, |
int | peak0, | ||
int | pin0, | ||
cdouble * | x, | ||
int | pc, | ||
double * | fps, | ||
double * | vps, | ||
int | N, | ||
NMSettings * | settings, | ||
double * | vfp, | ||
int ** | pitchind, | ||
int | newpc | ||
) |
function NoteMatchStiff3: finds harmonic atom from spectrum given the pitch as a (partial index, frequency) pair. This is used internally by NoteMatch3FB().
In: x[N/2+1]: spectrum fps[pc], vps[pc]: primitive (rough) peak frequencies and amplitudes R: initial F-G polygon constraint, optional pin0: partial index in the pitch specifier pair peak0: index to frequency in fps[] in the pitch specifier pair settings: note match settings Out: vfp[]: partial amplitudes R: F-G polygon of harmonic atom
Returns the total energy of HA.
void NoteMatchStiff3FB | ( | int & | pitchcount, |
TPolygon **& | R, | ||
double **& | vfp, | ||
double *& | sc, | ||
int * | newpitches, | ||
int * | prev, | ||
int | pc, | ||
double * | fps, | ||
double * | vps, | ||
cdouble * | x, | ||
int | wid, | ||
int | maxpitch, | ||
NMSettings * | settings | ||
) |
function NoteMatchStiff3FB: does one dynamic programming step in forward-background pitch tracking of harmonic sinusoids. This is used internally by FindNoteFB().
In: R[pitchcount]: initial F-G polygons associated with pitch candidates at last frame vfp[pitchcount][maxp]: amplitude vectors associated with pitch candidates at last frame sc[pitchcount]: accumulated scores associated with pitch candidates at last frame fps[pc], vps[pc]: primitive (rough) peak frequencies and amplitudes at this frame maxpitch: maximal number of pitch candidates x[wid/2+1]: spectrum settings: note match settings Out: pitchcount: number of pitch candidiate at this frame newpitches[pitchcount]: pitch candidates at this frame, whose lower word is peak index, higher word is partial index prev[pitchcount]: indices to predecessors of the pitch candidates at this frame R[pitchcount]: F-G polygons associated with pitch candidates at this frame vfp[pitchcount][maxp]: amplitude vectors associated with pitch candidates at this frame sc[pitchcount]: accumulated scores associated with pitch candidates at this frame
No return value.
double PeakShapeC | ( | double | f, |
int | Fr, | ||
int | N, | ||
cdouble ** | x, | ||
int | B, | ||
int | M, | ||
double * | c, | ||
double | iH2 | ||
) |
function PeakShapeC: residue-sinusoid-ratio for a given (hypothesis) sinusoid frequency
In: x[Fr][N/2+1]: spectrogram M, c[], iH2: cosine-family window specifiers f: reference frequency, in bins B: spectral truncation width
Returns the residue-sinusoid-ratio.
int QuickPeaks | ( | double * | f, |
double * | a, | ||
int | N, | ||
cdouble * | x, | ||
int | M, | ||
double * | c, | ||
double | iH2, | ||
double | mina, | ||
int | binst, | ||
int | binen, | ||
int | B, | ||
double * | rsr | ||
) |
function QuickPeaks: finds rough peaks in the spectrum (peak picking)
In: x[N/2+1]: spectrum M, c[], iH2: cosine-family window function specifiers mina: minimal amplitude to spot a spectral peak [binst, binen): frequency range, in bins, to look for peaks B: spectral truncation width Out; f[return value], a[return value]: frequencies (in bins) and amplitudes of found peaks rsr[return value]: residue-sinusoid-ratio of found peaks, optional
Returns the number of peaks found. f[] and a[] must be allocated enough space before calling.
int QuickPeaks | ( | double * | f, |
double * | a, | ||
int | Fr, | ||
int | N, | ||
cdouble ** | x, | ||
int | fr0, | ||
int | r0, | ||
int | M, | ||
double * | c, | ||
double | iH2, | ||
double | mina, | ||
int | binst, | ||
int | binen, | ||
int | B, | ||
double * | rsr | ||
) |
function QuickPeaks: finds rough peaks in the spectrogram (peak picking) for constant-frequency sinusoids
In: x[Fr][N/2+1]: spectrogram fr0, r0: centre and half width of interval (in frames) to use for peak picking M, c[], iH2: cosine-family window function specifiers mina: minimal amplitude to spot a spectral peak [binst, binen): frequency range, in bins, to look for peaks B: spectral truncation width Out; f[return value], a[return value]: frequencies (in bins) and summary amplitudes of found peaks rsr[return value]: residue-sinusoid-ratio of found peaks, optional
Returns the number of peaks found. f[] and a[] must be allocated enough space before calling.
void ReEstHS1 | ( | THS * | HS, |
__int16 * | Data16 | ||
) |
function ReEstHS1: wrapper function.
In: HS: a harmonic sinusoid Data16: its waveform data Out: HS: updated harmonic sinusoid
No return value.
double* SynthesisHS | ( | int | M, |
int | Fr, | ||
atom ** | partials, | ||
int & | dst, | ||
int & | den, | ||
bool * | terminatetag | ||
) |
function SynthesisHS: synthesizes a harmonic sinusoid without aligning the phases
In: partials[M][Fr]: HS partials terminatetag: external termination flag. Function SynthesisHS() polls *terminatetag and exits with 0 when it is set. Out: [dst, den): time interval synthesized xrec[den-dst]: resynthesized harmonic sinusoid
Returns pointer to xrec on normal finish, or 0 on external termination by setting $terminatetag. In the first case xrec is created anew with malloc8() and must be freed by caller using free8().
double* SynthesisHS2 | ( | int | M, |
int | Fr, | ||
atom ** | partials, | ||
int & | dst, | ||
int & | den, | ||
bool * | terminatetag | ||
) |
function SynthesisHS2: synthesizes a perfectly harmonic sinusoid without aligning the phases. Frequencies of partials above the fundamental are not used in this synthesis process.
In: partials[M][Fr]: HS partials terminatetag: external termination flag. This function polls *terminatetag and exits with 0 when it is set. Out: [dst, den) time interval synthesized xrec[den-dst]: resynthesized harmonic sinusoid
Returns pointer to xrec on normal finish, or 0 on external termination by setting $terminatetag. In the first case xrec is created anew with malloc8() and must be freed by caller using free8().
double* SynthesisHSp | ( | int | pm, |
int | pfr, | ||
atom ** | partials, | ||
int & | dst, | ||
int & | den, | ||
double ** | startamp, | ||
int | st_start, | ||
int | st_offst, | ||
int | st_count | ||
) |
function SynthesisHSp: synthesizes a harmonic sinusoid with phase alignment
In: partials[pm][pfr]: HS partials startamp[pm][st_count]: onset amplifiers, optional st_start, st_offst: start of and interval between onset amplifying points, optional Out: [dst, den): time interval synthesized xrec[den-dst]: resynthesized harmonic sinusoid
Returns pointer to xrec. xrec is created anew with malloc8() and must be freed by caller with free8().
double* SynthesisHSp | ( | THS * | HS, |
int & | dst, | ||
int & | den | ||
) |
function SynthesisHSp: wrapper function.
In: HS: a harmonic sinusoid. Out: [dst, den): time interval synthesized xrec[den-dst]: resynthesized harmonic sinusoid
Returns pointer to xrec, which is created anew with malloc8() and must be freed by caller using free8().
Generated on Wed Jun 18 2025 07:06:21 for x by
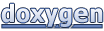