x
|
align8.h
Go to the documentation of this file.
32 pointer that is a multiple of 8. Aligning the stack does not automatically ensure that local double
33 -precision floating-point variables (or other variables one wants to align to 8-byte boundaries) are
Generated on Tue Jul 16 2024 07:04:48 for x by
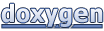