x
|
quickspec.h
Go to the documentation of this file.
78 __int24& __fastcall operator=(const __int32 a){loword=*(__int16*)&a; hibyte=((__int16*)&a)[1]; return *this;}
79 __int24& __fastcall operator=(const double f){__int32 a=f; loword=*(__int16*)&a; hibyte=((__int16*)&a)[1]; return *this;}
80 __fastcall operator __int32() const{__int32 result; *(__int16*)&result=loword; ((__int16*)&result)[1]=hibyte; return result;}
void * Parent
a pointer used as argument for calling GetFFTBuffers()
Definition: quickspec.h:142
int * Frame
indices to individual frames in internal storage
Definition: quickspec.h:147
WindowType WinType
window type for computing spectrogram
Definition: quickspec.h:150
int Id
an identifier given at create time, used as argument for calling GetFFTBuffers()
Definition: quickspec.h:141
Definition: quickspec.h:110
int DataLength
length of waveform audio, in samples
Definition: quickspec.h:154
int BytesPerSample
bytes per sample of waveform audio
Definition: quickspec.h:155
int * Valid
validity tags to individual frames in internal storage
Definition: quickspec.h:148
Definition: quickspec.h:55
double WinParam
additional parameter specifying certain window types (Gaussian, Kaiser, etc.)
Definition: quickspec.h:151
Definition: quickspec.h:71
GetBuf GetFFTBuffers
if specified, this supplies FFT buffers
Definition: quickspec.h:143
Definition: xcomplex.h:26
int Capacity
size of $Frame[] and &Valid[], usually set to the total number of frames of the data ...
Definition: quickspec.h:146
Generated on Fri Dec 27 2024 07:09:12 for x by
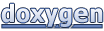