x
|
hs.h
Go to the documentation of this file.
138 stiffcandid(stiffcandid* prev, int ap, double af, double errf, double ds); //create by updating with new atom
177 void Resize(int aM, int aFr, bool copydata=false, bool forcealloc=false); //change size (M or Fr)
234 TTempAtom(TPolygon* AR, double delf1, double delf2, double minf); //create empty with extended R
235 TTempAtom(int apind, double af, double ef, double aa, double as, double maxB); //create with one partial
237 TTempAtom(TTempAtom* APrev, int apind, double af, double ef, double aa, double as, bool updateR=true); //duplicate and add one partial
245 void areaandcentroid(double& A, double& cx, double& cy, int N, double* x, double* y); //compute area and centroid
246 void cutcvpoly(int& N, double* x, double* y, double A, double B, double C, bool protect=false); //sever polygon by line
247 double maximalminimum(double& x, double& y, int N, double* sx, double* sy); //maximum inscribed circle
265 int QuickPeaks(double* f, double* a, int N, cdouble* x, int M, double* c, double iH2, double mina, int binst=-1, int binen=-1, int B=5, double* rsr=0);
266 int QuickPeaks(double* f, double* a, int Fr, int N, cdouble** x, int fr0, int r0, int M, double* c, double iH2, double mina, int binst=-1, int binen=-1, int B=5, double* rsr=0);
269 double NoteMatchStiff3(TPolygon* R, double &f0, double& B, int pc, double* fps, double* vps, int Fr, cdouble** x, int N, int offst, NMSettings* settings, NMResults* results, int lastp, double* lastvfp, double (*computes)(double a, void* params)=ds0, int forceinputlocalfr=-1); //basic grouping without rsr
270 double NoteMatchStiff3(TPolygon* R, double &f0, double& B, int pc, double* fps, double* vps, double* rsr, int Fr, cdouble** x, int N, int offst, NMSettings* settings, NMResults* results, int lastp, double* lastvfp, double (*computes)(double a, void* params)=ds0, int forceinputlocalfr=-1); //basic grouping with rsr
271 double NoteMatchStiff3(TPolygon* R, double &f0, double& B, int Fr, cdouble** x, int N, int offst, NMSettings* settings, NMResults* results, int lastp, double* lastvfp, double (*deltas)(double a, void* params)=ds0, bool forceinputlocalfr=false, int startfr=-1, int validfrrange=0); //basic grouping with rsr - wrapper
272 double NoteMatchStiff3(TPolygon* R, int peak0, int pin0, cdouble* x, int pc, double* fps, double* vps, int N, NMSettings* settings, double* vfp, int** pitchind, int newpc); //grouping with given pitch, single-frame
275 int FindNote(int _t, double _f, int& M, int& Fr, atom**& partials, int frst, int fren, int wid, int offst, TQuickSpectrogram* Spec, NMSettings settings); //harmonic sinusoid tracking (forward and backward tracking)
276 int FindNoteConst(int _t, double _f, int& M, int& Fr, atom**& partials, int frst, int fren, int wid, int offst, TQuickSpectrogram* Spec, NMSettings settings, double brake); //constant-pitch harmonic sinusoid tracking
277 int FindNoteF(atom* part, double& starts, TPolygon* R, int startp, double* startvfp, int frst, int fren, int wid, int offst, TQuickSpectrogram* Spec, NMSettings settings, double brake); //forward harmonic sinusoid tracking
278 int FindNoteFB(int frst, TPolygon* Rst, double* vfpst, int fren, TPolygon* Ren, double* vfpen, int M, atom** partials, int wid, int offst, TQuickSpectrogram* Spec, NMSettings settings); //forward-backward harmonic sinusoid tracking
279 void NoteMatchStiff3FB(int& pitchcount, TPolygon**& R, double**& vfp, double*& sc, int* newpitches, int* prev, int pc, double* fps, double* vps, cdouble* x, int wid, int maxpitch, NMSettings* settings); //single DP step of FindNoteFB()
282 double* SynthesisHS(int pm, int pfr, atom** partials, int& dst, int& den, bool* terminatetag=0);
285 double* SynthesisHSp(int pm, int pfr, atom** partials, int& dst, int& den, double** startamp=0, int st_start=0, int st_offst=0, int st_count=0);
double * SynthesisHSp(int pm, int pfr, atom **partials, int &dst, int &den, double **startamp=0, int st_start=0, int st_offst=0, int st_count=0)
Definition: hs.cpp:4733
void AtomsToPartials(int k, atom *part, int &M, int &Fr, atom **&partials, int offst)
Definition: hs.cpp:636
Definition: hs.h:49
double maximalminimum(double &x, double &y, int N, double *sx, double *sy)
Definition: hs.cpp:2164
int NMResultToPartials(int M, int fr, atom **Partials, int t, int wid, NMResults results)
Definition: hs.cpp:3427
Definition: hs.h:73
Definition: quickspec.h:110
Definition: hs.h:91
double NoteMatchStiff3(TPolygon *R, double &f0, double &B, int pc, double *fps, double *vps, int Fr, cdouble **x, int N, int offst, NMSettings *settings, NMResults *results, int lastp, double *lastvfp, double(*computes)(double a, void *params)=ds0, int forceinputlocalfr=-1)
Definition: hs.cpp:3459
double * SynthesisHS2(int M, int Fr, atom **partials, int &dst, int &den, bool *terminatetag=0)
Definition: hs.cpp:4669
void ExBStiff(double &Bmin, double &Bmax, int N, double *F, double *G)
Definition: hs.cpp:927
Definition: tstream.h:26
Definition: hs.h:123
void cutcvpoly(int &N, double *x, double *y, double A, double B, double C, bool protect=false)
Definition: hs.cpp:704
int QuickPeaks(double *f, double *a, int N, cdouble *x, int M, double *c, double iH2, double mina, int binst=-1, int binen=-1, int B=5, double *rsr=0)
Definition: hs.cpp:4366
int FindNoteF(atom *part, double &starts, TPolygon *R, int startp, double *startvfp, int frst, int fren, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings, double brake)
Definition: hs.cpp:1692
int FindNoteFB(int frst, TPolygon *Rst, double *vfpst, int fren, TPolygon *Ren, double *vfpen, int M, atom **partials, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings)
Definition: hs.cpp:1736
int FindNoteConst(int _t, double _f, int &M, int &Fr, atom **&partials, int frst, int fren, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings, double brake)
Definition: hs.cpp:1551
Definition: hs.h:147
void ExFmStiff(double &Fmin, double &Fmax, int m, int N, double *F, double *G)
Definition: hs.cpp:948
Definition: xcomplex.h:26
int NMResultToAtoms(int M, atom *HP, int t, int wid, NMResults results)
Definition: hs.cpp:3402
double PeakShapeC(double f, int Fr, int N, cdouble **x, int B, int M, double *c, double iH2)
Definition: hs.cpp:4321
void NoteMatchStiff3FB(int &pitchcount, TPolygon **&R, double **&vfp, double *&sc, int *newpitches, int *prev, int pc, double *fps, double *vps, cdouble *x, int wid, int maxpitch, NMSettings *settings)
Definition: hs.cpp:4222
int FindNote(int _t, double _f, int &M, int &Fr, atom **&partials, int frst, int fren, int wid, int offst, TQuickSpectrogram *Spec, NMSettings settings)
Definition: hs.cpp:1497
Definition: hs.h:83
void areaandcentroid(double &A, double &cx, double &cy, int N, double *x, double *y)
Definition: hs.cpp:603
Definition: hs.h:62
Definition: hs.h:199
Definition: hs.h:220
double * SynthesisHS(int pm, int pfr, atom **partials, int &dst, int &den, bool *terminatetag=0)
Definition: hs.cpp:4606
Generated on Sat Apr 26 2025 07:05:12 for x by
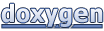