x
|
arrayalloc.h File Reference
#include <stdlib.h>
Include dependency graph for arrayalloc.h:
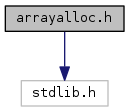
This graph shows which files directly or indirectly include this file:
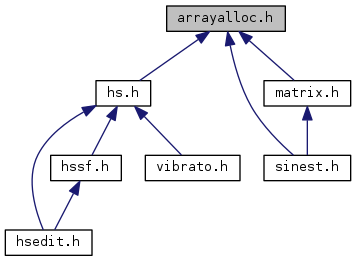
Go to the source code of this file.
Data Structures | |
class | MList |
Macros | |
#define | Alloc2(M, N, x) double **x=new double*[M]; x[0]=new double[(M)*(N)]; for (int _z=1; _z<M; _z++) x[_z]=&x[0][_z*(N)]; |
#define | Alloc2L(M, N, x, LIST) Alloc2(M, N, x); if (LIST) LIST->Add(x, 2); |
#define | Allocate2(INT, M, N, x) x=new INT*[M]; x[0]=new INT[(M)*(N)]; for (int _z=1; _z<M; _z++) x[_z]=&x[0][_z*(N)]; |
#define | Allocate2L(INT, M, N, x, LIST) Allocate2(INT, M, N, x); if (LIST) LIST->Add(x, 2); |
#define | DeAlloc2(x) {if (x) {delete[] (char*)(x[0]); delete[] x; x=0;}} |
#define | Alloc3(L, M, N, x) |
#define | Allocate3(INT, L, M, N, x) |
#define | Allocate3L(INT, M, N, O, x, LIST) Allocate3(INT, M, N, O, x); if (LIST) LIST->Add(x, 3); |
#define | DeAlloc3(x) {if (x) {delete[] (char*)(x[0][0]); delete[] x[0]; delete[] x; x=0;}} |
#define | Alloc4(L, M, N, O, x) |
#define | DeAlloc4(x) {if (x) {delete[] (char*)(x[0][0][0]); delete[] x[0][0]; delete[] x[0]; delete[] x; x=0;}} |
Detailed Description
- 2D, 3D and 4D array memory allocation routines.
An x-dimensional array (x=2, 3, 4, ...) is managed as a single memory block hosting all records, plus an index block which is a (x-1)D array itself. Therefore a 2D array is allocated as two 1D arrays, a 3D array is allocated as three 1D arrays, etc.
Examples: Alloc2(4, N, x) declares an array x[4][N] of double-precision floating points. Allocate3(int, K, L, M, x) allocates an array x[K][L][M] of integers and returns x as int***.
This file also includes a garbage collector class MList that works with arrays allcoated in this way.
Macro Definition Documentation
#define Alloc3 | ( | L, | |
M, | |||
N, | |||
x | |||
) |
Value:
double*** x=new double**[L]; x[0]=new double*[(L)*(M)]; x[0][0]=new double[(L)*(M)*(N)]; \
for (int _z=0; _z<L; _z++) {x[_z]=&x[0][_z*(M)]; x[_z][0]=&x[0][0][_z*(M)*(N)]; \
for (int __z=1; __z<M; __z++) x[_z][__z]=&x[_z][0][__z*(N)]; }
#define Alloc4 | ( | L, | |
M, | |||
N, | |||
O, | |||
x | |||
) |
Value:
double**** x=new double***[L]; x[0]=new double**[(L)*(M)]; \
x[0][0]=new double*[(L)*(M)*(N)]; x[0][0][0]=new double[(L)*(M)*(N)*(O)]; \
for (int _z=0; _z<L; _z++){ \
x[_z]=&x[0][_z*(M)]; x[_z][0]=&x[0][0][_z*(M)*(N)]; x[_z][0][0]=&x[0][0][0][_z*(M)*(N)*(O)]; \
for (int __z=0; __z<M; __z++){ \
x[_z][__z]=&x[_z][0][__z*(N)]; x[_z][__z][0]=&x[_z][0][0][__z*(N)*(O)]; \
for (int ___z=1; ___z<N; ___z++) x[_z][__z][___z]=&d[_z][__z][0][___z*(O)]; }}
#define Allocate3 | ( | INT, | |
L, | |||
M, | |||
N, | |||
x | |||
) |
Value:
x=new INT**[L]; x[0]=new INT*[(L)*(M)]; x[0][0]=new INT[(L)*(M)*(N)]; \
for (int _z=0; _z<L; _z++) {x[_z]=&x[0][_z*(M)]; x[_z][0]=&x[0][0][_z*(M)*(N)]; \
for (int __z=1; __z<M; __z++) x[_z][__z]=&x[_z][0][__z*(N)]; }
Generated on Tue Jul 1 2025 07:07:20 for x by
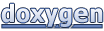