x
|
#include <memory.h>
#include <stdlib.h>
#include <values.h>
#include "fft.h"
#include "windowfunctions.h"

Go to the source code of this file.
Data Structures | |
struct | TickClock |
struct | fftparams |
struct | TGMM |
struct | TTFSpan |
struct | TSpecPeak |
class | TSpecTrack |
class | TTFSpans |
Macros | |
#define | testnn(x) {try{if (x<0) throw("testnn");}catch(...){x=0;}} |
#define | AllocateGMM(_MIXS, _DIM, _P, _M, _DEV) |
#define | ReleaseGMM(_P, _M, _DEV) delete[] _P; if (_M) {delete[] _M[0]; delete[] _M;} if (_DEV) {delete[] _DEV[0]; delete[] _DEV;} |
#define | ResetClock(AClock) {AClock.t=0;} |
#define | StartClock(AClock) {AClock.Ticks=GetTickCount();} |
#define | StopClock(AClock) {AClock.Ticks2=GetTickCount(); AClock.t+=0.001*(AClock.Ticks2-AClock.Ticks);} |
Functions | |
double | ACPower (double *data, int Count, void *) |
void | Add (double *dest, double *source, int Count) |
void | Add (double *out, double *addend, double *adder, int count) |
void | ApplyWindow (double *OutBuffer, double *Buffer, double *Weights, int Size) |
double | Avg (double *, int, void *) |
void | AvgFilter (double *dataout, double *data, int Count, int HWid) |
int | BitInv (int value, int order) |
void | CalculateSpectrogram (double *data, int Count, int start, int end, int Wid, int Offst, double *Window=0, double **Spec=0, double **Ph=0, double amp=1, bool half=true) |
void | Conv (double *out, int N1, double *in1, int N2, double *in2) |
void | DCT (double *output, double *input, int N) |
void | IDCT (double *output, double *input, int N) |
double | DCAmplitude (double *, int, void *) |
double | DCPower (double *, int, void *) |
double | ddIPHann (double, void *) |
void | DeDC (double *data, int Count, int HWid) |
void | DeDC_static (double *data, int Count) |
void | DoubleToInt (void *out, int BytesPerSample, double *in, int Count) |
void | DoubleToIntAdd (void *out, int BytesPerSample, double *in, int Count) |
double | DPower (double *data, int Count, void *) |
double | Energy (double *data, int Count) |
double | ExpOnsetFilter (double *dataout, double *data, int Count, double Tr, double Ta) |
double | ExpOnsetFilter_balanced (double *dataout, double *data, int Count, double Tr, double Ta, int bal=5) |
double | ExtractLinearComponent (double *dataout, double *data, int Count) |
void | FFTConv (double *dest, double *source1, int size1, double *source2, int size2, int zero=0, double *pre_buffer=NULL, double *post_buffer=NULL) |
void | FFTConv (unsigned char *dest, unsigned char *source1, int bps, int size1, double *source2, int size2, int zero=0, unsigned char *pre_buffer=NULL, unsigned char *post_buffer=NULL) |
void | FFTConv (double *dest, double *source1, int size1, double *source2, int size2, double *pre_buffer=NULL) |
void | FFTFilter (double *dataout, double *data, int Count, int Wid, int On, int Off) |
void | FFTFilterOLA (double *dataout, double *data, int Count, int Wid, int On, int Off, double *pre_buffer=NULL) |
void | FFTFilterOLA (unsigned char *dataout, unsigned char *data, int BytesPerSample, int Count, int Wid, int On, int Off, unsigned char *pre_buffer=NULL) |
void | FFTFilterOLA (double *dataout, double *data, int Count, double *amp, double *ph, int Wid, double *pre_buffer=NULL) |
double | FFTMask (double *dataout, double *data, int Count, int Wid, double DigiOn, double DigiOff, double maskcoef=1) |
int | FindInc (double value, double *data, int Count) |
double | Gaussian (int Dim, double *Vector, double *Mean, double *Dev, bool StartFrom1) |
void | HalfDCT (double *data, int Count, double *CC, int order) |
double | Hamming (double f, double T) |
double | Hann (double x, double N) |
double | HannSq (double x, double N) |
int | HxPeak2 (double *&hps, double *&vhps, double(*F)(double, void *), double(*dF)(double, void *), double(*ddF)(double, void *), void *params, double st, double en, double epf=1e-6) |
double | I0 (double x) |
int | InsertDec (double value, double *data, int Count) |
int | InsertDec (int value, int *data, int Count) |
int | InsertDec (double value, int index, double *data, int *indices, int Count) |
int | InsertInc (void *value, void **data, int Count, int(*Compare)(void *, void *)) |
int | InsertInc (double value, double *data, int Count, bool doinsert=true) |
int | InsertInc (double value, int index, double *data, int *indices, int Count) |
int | InsertInc (double value, double index, double *data, double *indices, int Count) |
int | InsertInc (double value, int *data, int Count, bool doinsert=true) |
int | InsertIncApp (double value, double *data, int Count) |
double | InstantFreq (int k, int hwid, cdouble *x, int mode=1) |
void | InstantFreq (double *freqspec, int hwid, cdouble *x, int mode=1) |
void | IntToDouble (double *out, void *in, int BytesPerSample, int Count) |
double | IPHannC (double f, cdouble *x, int N, int K1, int K2) |
double | IPHannC (double f, void *params) |
double | IPHannCP (double f, void *params) |
void | lse (double *x, double *y, int Count, double &a, double &b) |
void | memdoubleadd (double *dest, double *source, int count) |
void | MFCC (int FrameWidth, int NumBands, int Ceps_Order, double *Data, double *Bands, double *C, double *Amps, cdouble *W, cdouble *X) |
double * | MFCCPrepareBands (int NumberOfBands, int SamplesPerSec, int NumberOfBins) |
void | Multi (double *data, int count, double mul) |
void | Multi (double *out, double *in, int count, double mul) |
void | MultiAdd (double *out, double *in, double *adder, int count, double mul) |
int | NearestPeak (double *data, int count, int anindex) |
double | NegativeExp (double *x, double *y, int Count, double &lmd, int sample=1, double step=0.05, double eps=1e-6, int maxiter=50) |
double | NL (double *data, int Count, int Wid) |
double | Normalize (double *data, int Count, double Maxi=1) |
double | Normalize2 (double *data, int Count, double Norm=1) |
double | nextdouble (double x, double dir) |
double | PhaseSpan (double *data, int Count, void *) |
void | PolyFit (int P, double *a, int N, double *x, double *y) |
void | Pow (double *data, int Count, double ex) |
int | Rectify (double *data, int Count, double th=0) |
double | Res (double in, double mod) |
double | Romberg (int n, double(*f)(double, void *), double a, double b, void *params=0) |
double | Romberg (double(*f)(double, void *), double a, double b, void *params=0, int maxiter=50, double ep=1e-6) |
double | sinca (double x) |
double | sincd_unn (double x, int N) |
double | SmoothPhase (double *Arg, int Count, int mpi=2) |
double | StiffB (double f0, double fm, int m) |
double | StiffFm (double f0, int m, double B) |
double | StiffF0 (double fm, int m, double B) |
double | StiffM (double f0, double fm, double B) |
void | TFFilter (double *data, double *dataout, int Count, int Wid, TTFSpans *Spans, bool Pass, WindowType wt, double windp, int Sps, int TOffst=0) |
void | TFFilter (void *data, void *dataout, int BytesPerSample, int Count, int Wid, TTFSpans *Spans, bool Pass, WindowType wt, double windp, int Sps, int TOffst) |
void | TFMask (double *data, double *dataout, int Count, int Wid, TTFSpans *Spans, double masklevel, WindowType wt, double windp, int Sps, int TOffst) |
void | TFMask (void *data, void *dataout, int BytesPerSec, int Count, int Wid, TTFSpans *Spans, double masklevel, WindowType wt, double windp, int Sps, int TOffst) |
Detailed Description
- this file collects miscellaneous structures and functions. Not all of these are referenced somewhere else.
Macro Definition Documentation
#define AllocateGMM | ( | _MIXS, | |
_DIM, | |||
_P, | |||
_M, | |||
_DEV | |||
) |
#define testnn | ( | x | ) | {try{if (x<0) throw("testnn");}catch(...){x=0;}} |
macro testnn: non-negative test. This macro throws out an exception if the value of x is negative.
Function Documentation
double ACPower | ( | double * | data, |
int | Count, | ||
void * | |||
) |
function: ACPower: AC power
In: data[Count]: a signal
Returns the power of its AC content.
void Add | ( | double * | dest, |
double * | source, | ||
int | Count | ||
) |
function Add: vector addition
In: dest[Count], source[Count]: two vectors Out: dest[Count]: their sum
No return value.
void Add | ( | double * | out, |
double * | addend, | ||
double * | adder, | ||
int | count | ||
) |
function Add: vector addition
In: addend[count], adder[count]: two vectors Out: out[count]: their sum
No return value.
void ApplyWindow | ( | double * | OutBuffer, |
double * | Buffer, | ||
double * | Weights, | ||
int | Size | ||
) |
function ApplyWindow: applies window function to signal buffer.
In: Buffer[Size]: signal to be windowed Weight[Size]: the window Out: OutBuffer[Size]: windowed signal
No return value;
double Avg | ( | double * | data, |
int | Count, | ||
void * | |||
) |
function Avg: average
In: data[Count]: a data array
Returns the average of the array.
void AvgFilter | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
int | HWid | ||
) |
function AvgFilter: get slow-varying wave trace by averaging
In: data[Count]: input signal HWid: half the size of the averaging window Out: datout[Count]: the slow-varying part of data[].
No return value.
int BitInv | ( | int | Value, |
int | Order | ||
) |
function BitInv: inverse bit order of Value within an $Order-bit expression.
In: integer Value smaller than 2^Order
Returns an integer whose lowest Order bits are the lowest Order bits of Value in reverse order.
void CalculateSpectrogram | ( | double * | data, |
int | Count, | ||
int | start, | ||
int | end, | ||
int | Wid, | ||
int | Offst, | ||
double * | Window, | ||
double ** | Spec, | ||
double ** | Ph, | ||
double | amp, | ||
bool | half | ||
) |
function CalculateSpectrogram: computes the spectrogram of a signal
In: data[Count]: the time-domain signal start, end: start and end points marking the section for which the spectrogram is to be computed Wid, Offst: frame size and hop size Window: window function amp: a pre-amplifier half: specifies if the spectral values at Wid/2 are to be retried Out: Spec[][Wid/2] or Spec[][Wid/2+1]: amplitude spectrogram ph[][][Wid/2] or Ph[][Wid/2+1]: phase spectrogram
No return value. The caller is repsonse to arrange storage spance of output buffers.
void Conv | ( | double * | out, |
int | N1, | ||
double * | in1, | ||
int | N2, | ||
double * | in2 | ||
) |
function Conv: simple convolution
In: in1[N1], in2[N2]: two sequences Out: out[N1+N2-1]: their convolution
No return value.
double DCAmplitude | ( | double * | data, |
int | Count, | ||
void * | |||
) |
function DCAmplitude: DC amplitude
In: data[Count]: a signal
Returns its DC amplitude (=AC amplitude without DC removing)
double DCPower | ( | double * | data, |
int | Count, | ||
void * | |||
) |
function DCPower: DC power
In: data[Count]: a signal
Returns its DC power.
void DCT | ( | double * | output, |
double * | input, | ||
int | N | ||
) |
DCT: discrete cosine transform, direct computation. For fast DCT, see fft.cpp.
In: input[N]: a signal Out: output[N]: its DCT
No return value.
void DeDC | ( | double * | data, |
int | Count, | ||
int | HWid | ||
) |
function DeDC: removes DC component of a signal
In: data[Count]: the signal HWid: half of averaging window size Out: data[Count]: de-DC-ed signal
No return value.
void DeDC_static | ( | double * | data, |
int | Count | ||
) |
function DeDC_static: removes DC component statically
In: data[Count]: the signal Out: data[Count]: DC-removed signal
No return value.
void DoubleToInt | ( | void * | out, |
int | BytesPerSample, | ||
double * | in, | ||
int | Count | ||
) |
function DoubleToInt: converts double-precision floating point array to integer array
In: in[Count]: the double array BytesPerSample: bytes per sample of destination integers Out: out[Count]: the integer array
No return value.
void DoubleToIntAdd | ( | void * | out, |
int | BytesPerSample, | ||
double * | in, | ||
int | Count | ||
) |
function DoubleToIntAdd: adds double-precision floating point array to integer array
In: in[Count]: the double array out[Count]: the integer array BytesPerSample: bytes per sample of destination integers Out: out[Count]: the sum of the two arrays
No return value.
double DPower | ( | double * | data, |
int | Count, | ||
void * | |||
) |
DPower: in-frame power variation
In: data[Count]: a signal
returns the different between AC powers of its first and second halves.
double Energy | ( | double * | data, |
int | Count | ||
) |
function Energy: energy
In: data[Count]: a signal
Returns its total energy
double ExpOnsetFilter | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
double | Tr, | ||
double | Ta | ||
) |
function ExpOnsetFilter: onset filter with exponential impulse response h(t)=Aexp(-t/Tr)-Bexp(-t/Ta), A=1-exp(-1/Tr), B=1-exp(-1/Ta).
In: data[Count]: signal to filter Tr, Ta: time constants of h(t) Out: dataout[Count]: filtered signal, normalized by multiplying a factor.
Returns the normalization factor. Identical data and dataout is allowed.
double ExpOnsetFilter_balanced | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
double | Tr, | ||
double | Ta, | ||
int | bal | ||
) |
function ExpOnsetFilter_balanced: exponential onset filter without starting step response. It extends the input signal at the front end by bal*Ta samples by repeating the its value at 0, then applies the onset filter on the extended signal instead.
In: data[Count]: signal to filter Tr, Ta: time constants to the impulse response of onset filter, see ExpOnsetFilter(). bal: balancing factor Out: dataout[Count]: filtered signal, normalized by multiplying a factor.
Returns the normalization factor. Identical data and dataout is allowed.
double ExtractLinearComponent | ( | double * | dataout, |
double * | data, | ||
int | Count | ||
) |
function ExtractLinearComponent: Legendre linear component
In: data[Count+1]: a signal Out: dataout[Count+1]: its Legendre linear component, optional.
Returns the coefficient to the linear component.
void FFTConv | ( | double * | dest, |
double * | source1, | ||
int | size1, | ||
double * | source2, | ||
int | size2, | ||
int | zero, | ||
double * | pre_buffer, | ||
double * | post_buffer | ||
) |
function FFTConv: fast convolution of two series by FFT overlap-add. In an overlap-add scheme it is assumed that one of the convolvends is short compared to the other one, which can be potentially infinitely long. The long convolvend is devided into short segments, each of which is convolved with the short convolvend, the results of which are then assembled into the final result. The minimal delay from input to output is the amount of overlap, which is the size of the short convolvend minus 1.
In: source1[size1]: convolvend source2[size2]: second convolvend zero: position of first point in convoluton result, relative to main output buffer. pre_buffer[-zero]: buffer hosting values to be overlap-added to the start of the result. Out: dest[size1]: the middle part of convolution result pre_buffer[-zero]: now updated by adding beginning part of the convolution result post_buffer[size2+zero]: end part of the convolution result
No return value. Identical dest and source1 allowed.
The convolution result has length size1+size2 (counting one trailing zero). If zero lies in the range between -size2 and 0, then the first -zero samples are added to pre_buffer[], next size1 samples are saved to dest[], and the last size2+zero sampled are saved to post_buffer[]; if not, the middle size1 samples are saved to dest[], while pre_buffer[] and post_buffer[] are not used.
void FFTConv | ( | unsigned char * | dest, |
unsigned char * | source1, | ||
int | bps, | ||
int | size1, | ||
double * | source2, | ||
int | size2, | ||
int | zero, | ||
unsigned char * | pre_buffer, | ||
unsigned char * | post_buffer | ||
) |
function FFTConv: fast convolution of two series by FFT overlap-add. Same as the three-output-buffer version above but using integer output buffers as well as integer source1.
In: source1[size1]: convolvend bps: bytes per sample of integer units in source1[]. source2[size2]: second convolvend zero: position of first point in convoluton result, relative to main output buffer. pre_buffer[-zero]: buffer hosting values to be overlap-added to the start of the result. Out: dest[size1]: the middle part of convolution result pre_buffer[-zero]: now updated by adding beginning part of the convolution result post_buffer[size2+zero]: end part of the convolution result
No return value. Identical dest and source1 allowed.
void FFTConv | ( | double * | dest, |
double * | source1, | ||
int | size1, | ||
double * | source2, | ||
int | HWid, | ||
double * | pre_buffer | ||
) |
function FFTConv: the simplified version using two output buffers instead of three. This is almost equivalent to setting zero=-size2 in the three-output-buffer version (so that post_buffer no longer exists), except that this version requires size2 (renamed HWid) be a power of 2, and pre_buffer point to the END of the storage (so that pre_buffer=dest automatically connects the two buffers in a continuous memory block).
In: source1[size1]: convolvend source2[HWid]: second convolved, HWid be a power of 2 pre_buffer[-HWid:-1]: buffer hosting values to be overlap-added to the start of the result. Out: dest[size1]: main output buffer, now hosting end part of the result (after HWid samples). pre_buffer[-HWid:-1]: now updated by added the start of the result
No return value.
void FFTFilter | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
int | Wid, | ||
int | On, | ||
int | Off | ||
) |
function FFTFilter: FFT with cosine-window overlap-add: This FFT filter is not an actural LTI system, but an block processing with overlap-add. In this function the blocks are overlapped by 50% and summed up with Hann windowing.
In: data[Count]: input data Wid: DFT size On, Off: cut-off frequencies of FFT filter. On<Off: band-pass; On>Off: band-stop. Out: dataout[Count]: filtered data
No return value. Identical data and dataout allowed
void FFTFilterOLA | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
int | Wid, | ||
int | On, | ||
int | Off, | ||
double * | pre_buffer | ||
) |
function FFTFilterOLA: FFTFilter with overlap-add support. This is a true LTI filter whose impulse response is constructed using IFFT. The filtering is implemented by fast convolution.
In: data[Count]: input data Wid: FFT size On, Off: cut-off frequencies, in bins, of the filter pre_buffer[Wid]: buffer hosting sampled to be added with the start of output Out: dataout[Count]: main output buffer, hosting the last $Count samples of output. pre_buffer[Wid]: now updated by adding the first Wid samples of output
No return value. The complete output contains Count+Wid effective samples (including final 0); firt $Wid are added to pre_buffer[], next Count samples saved to dataout[].
void FFTFilterOLA | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
double * | amp, | ||
double * | ph, | ||
int | Wid, | ||
double * | pre_buffer | ||
) |
function FFTFilterOLA: FFT filter with overlap-add support.
In: data[Count]: input data amp[0:HWid]: amplitude response ph[0:HWid]: phase response, where ph[0]=ph[HWid]=0; pre_buffer[Wid]: buffer hosting sampled to be added to the beginning of the output Out: dataout[Count]: main output buffer, hosting the middle $Count samples of output. pre_buffer[Wid]: now updated by adding the first Wid/2 samples of output
No return value.
double FFTMask | ( | double * | dataout, |
double * | data, | ||
int | Count, | ||
int | Wid, | ||
double | DigiOn, | ||
double | DigiOff, | ||
double | maskcoef | ||
) |
function FFTMask: masks a band of a signal with noise
In: data[Count]: input signal DigiOn, DigiOff: cut-off frequences of the band to mask maskcoef: masking noise amplifier. If set to 1 than the mask level is set to the highest signal level in the masking band. Out: dataout[Count]: output data
No return value.
int FindInc | ( | double | value, |
double * | data, | ||
int | Count | ||
) |
function FindInc: find the element in ordered list data that is closest to value.
In: data[Count]: a ordered list value: the value to locate in the list
Returns the index of the element in the sorted list which is closest to $value.
double Hamming | ( | double | f, |
double | T | ||
) |
function Hamming: calculates the amplitude spectrum of Hamming window at a given frequency
In: f: frequency T: size of Hamming window
Returns the amplitude spectrum at specified frequency.
double Hann | ( | double | x, |
double | N | ||
) |
function Hann: computes the Hann window amplitude spectrum (window-size-normalized).
In: x: frequency, in bins N: size of Hann window
Return the amplitude spectrum evaluated at x. Maximum 0.5 is reached at x=0. Time 2 to normalize maximum to 1.
double HannSq | ( | double | x, |
double | N | ||
) |
function HannSq: computes the square norm of Hann window spectrum (window-size-normalized)
In: x: frequency, in bins N: size of Hann window
Return the square norm.
int HxPeak2 | ( | double *& | hps, |
double *& | vhps, | ||
double(*)(double, void *) | F, | ||
double(*)(double, void *) | dF, | ||
double(*)(double, void *) | ddF, | ||
void * | params, | ||
double | st, | ||
double | en, | ||
double | epf | ||
) |
function HxPeak2: fine spectral peak detection. This does detection and high-precision LSE estimation in one go. However, since in practise most peaks are spurious, LSE estimation is not necessary on them. Accordingly, HxPeak2 is deprecated in favour of faster but coarser peak picking methods, such as QIFFT, which leaves fine estimation to a later stage of processing.
In: F, dF, ddF: pointers to functions that compute LSE peak energy for, plus its 1st and 2nd derivatives against, a given frequency. params: pointer to a data structure (l_hx) hosting input data fed to F, dF, and ddF (st, en): frequency range, in bins, to search for peaks in epf: convergence detection threshold Out: hps[return value]: peak frequencies vps[return value]; peak amplitudes
Returns the number of peaks detected.
double I0 | ( | double | x | ) |
function I0: Bessel function of order zero
Returns the Bessel function of x.
void IDCT | ( | double * | output, |
double * | input, | ||
int | N | ||
) |
function IDCT: inverse discrete cosine transform, direct computation. For fast IDCT, see fft.cpp.
In: input[N]: a signal Out: output[N]: its IDCT
No return value.
int InsertDec | ( | int | value, |
int * | data, | ||
int | Count | ||
) |
function InsertDec: inserts value into sorted decreasing list
In: data[Count]: a sorted decreasing list. value: the value to be added Out: data[Count]: the list with $value inserted if the latter is larger than its last entry, in which case the original last entry is discarded.
Returns the index where $value is located in data[], or -1 if $value is smaller than or equal to data[Count-1].
int InsertDec | ( | double | value, |
int | index, | ||
double * | data, | ||
int * | indices, | ||
int | Count | ||
) |
function InsertDec: inserts value and attached integer into sorted decreasing list
In: data[Count]: a sorted decreasing list indices[Count]: a list of integers attached to entries of data[] value, index: the value to be added and its attached integer Out: data[Count], indices[Count]: the lists with $value and $index inserted if $value is larger than the last entry of data[], in which case the original last entries are discarded.
Returns the index where $value is located in data[], or -1 if $value is smaller than or equal to data[Count-1].
int InsertInc | ( | double | value, |
double * | data, | ||
int | Count, | ||
bool | doinsert | ||
) |
function InsertInc: inserts value into sorted increasing list
In: data[Count]: a sorted increasing list. value: the value to be added doinsert: specifies whether the actually insertion is to be performed Out: data[Count]: the list with $value inserted if the latter is smaller than its last entry, in which case the original last entry of data[] is discarded.
Returns the index where $value is located in data[], or -1 if value is larger than or equal to data[Count-1].
int InsertInc | ( | double | value, |
int | index, | ||
double * | data, | ||
int * | indices, | ||
int | Count | ||
) |
function InsertInc: inserts value and attached integer into sorted increasing list
In: data[Count]: a sorted increasing list indices[Count]: a list of integers attached to entries of data[] value, index: the value to be added and its attached integer Out: data[Count], indices[Count]: the lists with $value and $index inserted if $value is smaller than the last entry of data[], in which case the original last entries are discarded.
Returns the index where $value is located in data[], or -1 if $value is larger than or equal to data[Count-1].
int InsertIncApp | ( | double | value, |
double * | data, | ||
int | Count | ||
) |
function InsertIncApp: inserts value into flexible-length sorted increasing list
In: data[Count]: a sorted increasing list. value: the value to be added Out: data[Count+1]: the list with $value inserted.
Returns the index where $value is located in data[], or -1 if Count<0. data[] must have Count+1 storage units on calling.
double InstantFreq | ( | int | k, |
int | hwid, | ||
cdouble * | x, | ||
int | mode | ||
) |
function InstantFreq; calculates instantaneous frequency from spectrum, evaluated at bin k
In: x[hwid]: spectrum with scale 2hwid k: reference frequency, in bins mode: must be 1.
Returns an instantaneous frequency near bin k.
void InstantFreq | ( | double * | freqspec, |
int | hwid, | ||
cdouble * | x, | ||
int | mode | ||
) |
function InstantFreq; calculates "frequency spectrum", a sequence of frequencies evaluated at DFT bins
In: x[hwid]: spectrum with scale 2hwid mode: must be 1. Out: freqspec[hwid]: the frequency spectrum
No return value.
void IntToDouble | ( | double * | out, |
void * | in, | ||
int | BytesPerSample, | ||
int | Count | ||
) |
function IntToDouble: copy content of integer array to double array
In: in: pointer to integer array BytesPerSample: number of bytes each integer takes Count: size of integer array, in integers Out: vector out[Count].
No return value.
double IPHannC | ( | double | f, |
cdouble * | x, | ||
int | N, | ||
int | K1, | ||
int | K2 | ||
) |
function IntToDouble: copy content of integer array to double array
In: in: pointer to integer array BytesPerSample: number of bytes each integer takes Count: size of integer array, in integers Out: vector out[Count].
No return value.
This version is currently commented out in favour of the version implemented in QuickSpec.cpp which supports 24-bit integers. function IPHannC: inner product with Hann window spectrum
In: x[N]: spectrum f: reference frequency K1, K2: spectral truncation bounds
Returns the absolute value of the inner product of x[K1:K2] with the corresponding band of the spectrum of a sinusoid at frequency f.
void lse | ( | double * | x, |
double * | y, | ||
int | Count, | ||
double & | a, | ||
double & | b | ||
) |
function lse: linear regression y=ax+b
In: x[Count], y[Count]: input points Out: a, b: LSE estimation of coefficients in y=ax+b
No return value.
void memdoubleadd | ( | double * | dest, |
double * | source, | ||
int | count | ||
) |
memdoubleadd: vector addition
In: dest[count], source[count]: addends Out: dest[count]: sum
No return value.
void MFCC | ( | int | FrameWidth, |
int | NumBands, | ||
int | Ceps_Order, | ||
double * | Data, | ||
double * | Bands, | ||
double * | C, | ||
double * | Amps, | ||
cdouble * | W, | ||
cdouble * | X | ||
) |
function MFCC: calculates MFCC.
In: Data[FrameWidth]: data NumBands: number of frequency bands on mel scale Bands[3*NumBands]: mel frequency bands, comes as $NumBands triples, each containing the lower, middle and high frequencies, in bins, of one band, from which a weighting window is created to weight individual bins. Ceps_Order: number of MFC coefficients (i.e. DCT coefficients) W, X: FFT buffers Out: C[Ceps_Order]: MFCC Amps[NumBands]: log spectrum on MF bands
No return value. Use MFCCPrepareBands() to retrieve Bands[].
double* MFCCPrepareBands | ( | int | NumberOfBands, |
int | SamplesPerSec, | ||
int | NumberOfBins | ||
) |
function MFCCPrepareBands: returns a array of OVERLAPPING bands given in triples, whose 1st and 3rd entries are the start and end of a band, in bins, and the 2nd is a middle frequency.
In: SamplesPerSec: sampling rate NumberOfBins: FFT size NumberOfBands: number of mel-frequency bands
Returns pointer to the array of triples.
void Multi | ( | double * | data, |
int | count, | ||
double | mul | ||
) |
function Multi: vector-constant multiplication
In: data[count]: a vector mul: a constant Out: data[count]: their product
No return value.
void Multi | ( | double * | out, |
double * | in, | ||
int | count, | ||
double | mul | ||
) |
function Multi: vector-constant multiplication
In: in[count]: a vector mul: a constant Out: out[count]: their product
No return value.
void MultiAdd | ( | double * | out, |
double * | in, | ||
double * | adder, | ||
int | count, | ||
double | mul | ||
) |
function Multi: vector-constant multiply-addition
In: in[count], adder[count]: vectors mul: a constant Out: out[count]: in[]+adder[]*mul
No return value.
int NearestPeak | ( | double * | data, |
int | count, | ||
int | anindex | ||
) |
function NearestPeak: finds a peak value in an array that is nearest to a given index
In: data[count]: an array anindex: an index
Returns the index to a peak of data[] that is closest to anindex. In case of two cloest indices, returns the index to the higher peak of the two.
double NegativeExp | ( | double * | x, |
double * | y, | ||
int | Count, | ||
double & | lmd, | ||
int | sample, | ||
double | step, | ||
double | eps, | ||
int | maxiter | ||
) |
function NegativeExp: fits the curve y=1-x^lmd.
In: x[Count], y[Count]: sample points to fit, x[0]=0, x[Count-1]=1, y[0]=1, y[Count-1]=0 Out: lmd: coefficient of y=1-x^lmd.
Returns rms fitting error.
double NL | ( | double * | data, |
int | Count, | ||
int | Wid | ||
) |
function: NL: noise level, calculated on 5% of total frames with least energy
In: data[Count]: Wid: window width for power level estimation
Returns noise level, in rms.
double Normalize | ( | double * | data, |
int | Count, | ||
double | Maxi | ||
) |
function Normalize: normalizes data to [-Maxi, Maxi], without zero shift
In: data[Count]: data to be normalized Maxi: destination maximal absolute value Out: data[Count]: normalized data
Returns the original maximal absolute value.
double Normalize2 | ( | double * | data, |
int | Count, | ||
double | Norm | ||
) |
function Normalize2: normalizes data to a specified Euclidian norm
In: data[Count]: data to normalize Norm: destination Euclidian norm Out: data[Count]: normalized data.
Returns the original Euclidian norm.
double PhaseSpan | ( | double * | data, |
int | Count, | ||
void * | aparams | ||
) |
function PhaseSpan: computes the unwrapped phase variation across the Nyquist range
In: data[Count]: time-domain data aparams: a fftparams structure
Returns the difference between unwrapped phase angles at 0 and Nyquist frequency.
void PolyFit | ( | int | P, |
double * | a, | ||
int | N, | ||
double * | x, | ||
double * | y | ||
) |
function PolyFit: least square polynomial fitting y=sum(i){a[i]*x^i}
In: x[N], y[N]: sample points P: order of polynomial, P<N Out: a[P+1]: coefficients of polynomial
No return value.
void Pow | ( | double * | data, |
int | Count, | ||
double | ex | ||
) |
function Pow: vector power function
In: data[Count]: a vector ex: expontnet Out: data[Count]: point-wise $ex-th power of data[]
No return value.
int Rectify | ( | double * | data, |
int | Count, | ||
double | th | ||
) |
Rectify: semi-wave rectification
In: data[Count]: data to rectify th: rectification threshold: values below th are rectified to th Out: data[Count]: recitified data
Returns number of preserved (i.e. not rectified) samples.
double Res | ( | double | in, |
double | mod | ||
) |
function Res: minimum absolute residue.
In: in: a number mod: modulus
Returns the minimal absolute residue of $in devided by $mod.
double Romberg | ( | int | n, |
double(*)(double, void *) | f, | ||
double | a, | ||
double | b, | ||
void * | params | ||
) |
function Romberg: Romberg algorithm for numerical integration
In: f: function to integrate params: extra argument for calling f a, b: integration boundaries n: depth of sampling
Returns the integral of f(*, params) over [a, b].
double Romberg | ( | double(*)(double, void *) | f, |
double | a, | ||
double | b, | ||
void * | params, | ||
int | n, | ||
double | ep | ||
) |
function Romberg: Romberg algorithm for numerical integration, may return before specified depth on convergence.
In: f: function to integrate params: extra argument for calling f a, b: integration boundaries n: depth of sampling ep: convergence test threshold
Returns the integral of f(*, params) over [a, b].
double sinca | ( | double | x | ) |
function sinca: analog sinc function.
In: x: frequency
Returns sinc(x)=sin(pi*x)/(pi*x), sinca(0)=1, sinca(1)=0
double sincd_unn | ( | double | x, |
int | N | ||
) |
function sincd_unn: unnormalized discrete sinc function
In: x: frequency N: scale (window size, DFT size)
Returns sinc(x)=sin(pi*x)/sin(pi*x/N), sincd(0)=N, sincd(1)=0.
double SmoothPhase | ( | double * | Arg, |
int | Count, | ||
int | mpi | ||
) |
SmoothPhase: phase unwrapping on module mpi*PI, 2PI by default
In: Arg[Count]: phase angles to unwrap mpi: unwrapping modulus, in pi's Out: Arg[Count]: unwrapped phase
Returns the amount of unwrap, in pi's, of the last phase angle
double StiffB | ( | double | f0, |
double | fm, | ||
int | m | ||
) |
StiffB: computes stiffness coefficient from fundamental and another partial frequency based on the stiff string partial frequency model f[m]=mf[1]*sqrt(1+B(m*m-1)).
In: f0: fundamental frequency m: 1-based partial index fm: frequency of partial m
Returns stiffness coefficient B.
double StiffF0 | ( | double | fm, |
int | m, | ||
double | B | ||
) |
StiffF0: computes fundamental frequency from another partial frequency and stiffness coefficient based on the stiff string partial frequency model f[m]=mf[1]*sqrt(1+B(m*m-1)).
In: m: 1-based partial index fm: frequency of partial m B: stiffness coefficient
Returns the fundamental frequency.
double StiffFm | ( | double | f0, |
int | m, | ||
double | B | ||
) |
StiffFm: computes a partial frequency from fundamental frequency and partial index based on the stiff string partial frequency model f[m]=mf[1]*sqrt(1+B(m*m-1)).
In: f0: fundamental frequency m: 1-based partial index B: stiffness coefficient
Returns frequency of the m-th partial.
double StiffM | ( | double | f0, |
double | fm, | ||
double | B | ||
) |
StiffM: computes 1-based partial index from partial frequency, fundamental frequency and stiffness coefficient based on the stiff string partial frequency model f[m]=mf[1]*sqrt(1+B(m*m-1)).
In: f0: fundamental freqency fm: a partial frequency B: stiffness coefficient
Returns the 1-based partial index which will generate the specified partial frequency from the model which, however, does not have to be an integer in this function.
void TFFilter | ( | double * | data, |
double * | dataout, | ||
int | Count, | ||
int | Wid, | ||
TTFSpans * | Spans, | ||
bool | Pass, | ||
WindowType | wt, | ||
double | windp, | ||
int | Sps, | ||
int | TOffst | ||
) |
TFFilter: time-frequency filtering with Hann-windowed overlap-add.
In: data[Count]: input data Spans: time-frequency spans wt, windp: type and extra parameter of FFT window Sps: sampling rate TOffst: optional offset applied to all time values in Spans, set to Spans timing of of data[0]. Pass: set to pass T-F content covered by Spans, clear to stop T-F content covered by Spans Out: dataout[Count]: filtered data
No return value. Identical data and dataout allowed.
Generated on Tue Jul 16 2024 07:04:48 for x by
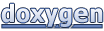