x
|
windowfunctions.h
Go to the documentation of this file.
40 double* NewdddWindow(WindowType wt, int Count, int* ips=0, double* dps=0, double* newdddwindow=0);
double * NewdWindow(WindowType wt, int Count, int *ips=0, double *dps=0, double *newdwindow=0)
Definition: windowfunctions.cpp:164
double * NewdddWindow(WindowType wt, int Count, int *ips=0, double *dps=0, double *newdddwindow=0)
Definition: windowfunctions.cpp:297
double * NewWindow8(WindowType wt, int Count, int *ips=0, double *dps=0, double *newwindow=0)
Definition: windowfunctions.cpp:147
double * NewddWindow(WindowType wt, int Count, int *ips=0, double *dps=0, double *newddwindow=0)
Definition: windowfunctions.cpp:230
void windowspec(WindowType wt, int N, int *M, double *c, double *iH2, double *d=0)
Definition: windowfunctions.cpp:364
double * NewWindow(WindowType wt, int Count, int *ips=0, double *dps=0, double *newwindow=0)
Definition: windowfunctions.cpp:128
void FillWindow(double *newwindow, WindowType wt, int Count, int *ips=0, double *dps=0)
Definition: windowfunctions.cpp:53
Generated on Tue Jul 8 2025 07:11:37 for x by
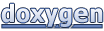