svgui
1.9
|
PluginParameterDialog.h
Go to the documentation of this file.
void setChannelArrangement(int sourceChannels, int targetChannels, int defaultChannel)
Definition: PluginParameterDialog.cpp:297
QString getInputModel() const
Definition: PluginParameterDialog.cpp:517
PluginParameterDialog(std::shared_ptr< Vamp::PluginBase > plugin, QWidget *parent=0)
Definition: PluginParameterDialog.cpp:46
bool m_currentSelectionOnly
Definition: PluginParameterDialog.h:127
void pluginConfigurationChanged(QString)
void inputModelComboChanged(int)
Definition: PluginParameterDialog.cpp:611
void setAdvancedVisible(bool)
Definition: PluginParameterDialog.cpp:582
void advancedToggled()
Definition: PluginParameterDialog.cpp:576
void getProcessingParameters(int &blockSize) const
!! merge with PluginTransform::ExecutionContext
Definition: PluginParameterDialog.cpp:529
void inputModelChanged(QString)
std::shared_ptr< Vamp::PluginBase > getPlugin()
Definition: PluginParameterDialog.h:67
void channelComboChanged(int)
Definition: PluginParameterDialog.cpp:605
void windowTypeChanged(WindowType type)
Definition: PluginParameterDialog.cpp:562
QGroupBox * m_inputModelBox
Definition: PluginParameterDialog.h:122
void setCandidateInputModels(const QStringList &names, QString defaultName)
Definition: PluginParameterDialog.cpp:459
void setShowSelectionOnlyOption(bool show)
Definition: PluginParameterDialog.cpp:493
void setOutputLabel(QString output, QString description)
Definition: PluginParameterDialog.cpp:261
PluginParameterBox * m_parameterBox
Definition: PluginParameterDialog.h:106
bool m_advancedVisible
Definition: PluginParameterDialog.h:131
QComboBox * m_inputModels
Definition: PluginParameterDialog.h:123
void setShowProcessingOptions(bool showWindowSize, bool showFrequencyDomainOptions)
Definition: PluginParameterDialog.cpp:361
QString m_currentInputModel
Definition: PluginParameterDialog.h:126
~PluginParameterDialog()
Definition: PluginParameterDialog.cpp:247
QLabel * m_outputDescription
Definition: PluginParameterDialog.h:110
void blockSizeComboChanged(const QString &)
Definition: PluginParameterDialog.cpp:547
A dialog for editing the parameters of a given plugin, using a PluginParameterBox.
Definition: PluginParameterDialog.h:43
bool m_haveChannelBoxData
Definition: PluginParameterDialog.h:117
void setMoreInfoUrl(QString url)
Definition: PluginParameterDialog.cpp:286
void selectionOnlyChanged(int)
Definition: PluginParameterDialog.cpp:619
QPushButton * m_advancedButton
Definition: PluginParameterDialog.h:129
Definition: PluginParameterBox.h:31
void incrementComboChanged(const QString &)
Definition: PluginParameterDialog.cpp:554
bool m_haveWindowBoxData
Definition: PluginParameterDialog.h:120
bool getSelectionOnly() const
Definition: PluginParameterDialog.cpp:523
std::shared_ptr< Vamp::PluginBase > m_plugin
Definition: PluginParameterDialog.h:99
QStringList m_inputModelList
Definition: PluginParameterDialog.h:125
QCheckBox * m_selectionOnly
Definition: PluginParameterDialog.h:124
Generated by
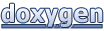