svgui
1.9
|
PluginParameterBox.h
Go to the documentation of this file.
void pluginConfigurationChanged(QString)
std::map< QString, ParamRec > m_params
Definition: PluginParameterBox.h:68
std::map< QString, QString > m_nameMap
Definition: PluginParameterBox.h:69
AudioDial is a nicer-looking QDial that by default reacts to mouse movement on horizontal and vertica...
Definition: AudioDial.h:60
std::shared_ptr< Vamp::PluginBase > getPlugin()
Definition: PluginParameterBox.h:40
void programComboChanged(const QString &)
Definition: PluginParameterBox.cpp:379
Vamp::PluginBase::ProgramList m_programs
Definition: PluginParameterBox.h:70
PluginParameterBox(std::shared_ptr< Vamp::PluginBase >, QWidget *parent=0)
Definition: PluginParameterBox.cpp:37
std::shared_ptr< Vamp::PluginBase > m_plugin
Definition: PluginParameterBox.h:56
Vamp::PluginBase::ParameterDescriptor param
Definition: PluginParameterBox.h:63
Definition: PluginParameterBox.h:31
void updateProgramCombo()
Definition: PluginParameterBox.cpp:429
Generated by
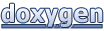