svgui
1.9
|
AudioDial is a nicer-looking QDial that by default reacts to mouse movement on horizontal and vertical axes instead of in a radial motion. More...
#include <AudioDial.h>
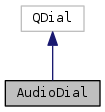
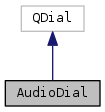
Public Slots | |
void | setKnobColor (const QColor &color) |
Set the colour of the knob. More... | |
void | setMeterColor (const QColor &color) |
Set the colour of the meter (the highlighted area around the knob that shows the current value). More... | |
void | setMouseDial (bool mouseDial) |
Specify that the dial should respond to radial mouse movements in the same way as QDial. More... | |
void | setDefaultValue (int defaultValue) |
void | setValue (int value) |
void | setDefaultMappedValue (double mappedValue) |
void | setMappedValue (double mappedValue) |
void | setToDefault () |
void | edit () |
Signals | |
void | mouseEntered () |
void | mouseLeft () |
Public Member Functions | |
AudioDial (QWidget *parent=0) | |
~AudioDial () | |
const QColor & | getKnobColor () const |
const QColor & | getMeterColor () const |
bool | getMouseDial () const |
void | setRangeMapper (RangeMapper *mapper) |
const RangeMapper * | rangeMapper () const |
double | mappedValue () const |
int | defaultValue () const |
void | setShowToolTip (bool show) |
void | setProvideContextMenu (bool provide) |
Protected Slots | |
void | updateMappedValue (int value) |
void | contextMenuRequested (const QPoint &) |
Protected Member Functions | |
void | drawTick (QPainter &paint, double angle, int size, bool internal) |
void | paintEvent (QPaintEvent *) override |
void | mousePressEvent (QMouseEvent *pMouseEvent) override |
void | mouseMoveEvent (QMouseEvent *pMouseEvent) override |
void | mouseReleaseEvent (QMouseEvent *pMouseEvent) override |
void | mouseDoubleClickEvent (QMouseEvent *pMouseEvent) override |
void | enterEvent (QEvent *) override |
void | leaveEvent (QEvent *) override |
Properties | |
QColor | knobColor |
QColor | meterColor |
bool | mouseDial |
Private Attributes | |
QColor | m_knobColor |
QColor | m_meterColor |
int | m_defaultValue |
double | m_defaultMappedValue |
double | m_mappedValue |
bool | m_noMappedUpdate |
bool | m_mouseDial |
bool | m_mousePressed |
QPoint | m_posMouse |
bool | m_showTooltip |
bool | m_provideContextMenu |
QString | m_title |
QMenu * | m_lastContextMenu |
RangeMapper * | m_rangeMapper |
Detailed Description
AudioDial is a nicer-looking QDial that by default reacts to mouse movement on horizontal and vertical axes instead of in a radial motion.
Move the mouse up or right to increment the value, down or left to decrement it. AudioDial also responds to the mouse wheel.
The programming interface for this widget is compatible with QDial, with the addition of properties for the knob colour and meter colour and a boolean property mouseDial that determines whether to respond to radial mouse motion in the same way as QDial (the default is no).
Definition at line 60 of file AudioDial.h.
Constructor & Destructor Documentation
AudioDial::AudioDial | ( | QWidget * | parent = 0 | ) |
Definition at line 76 of file AudioDial.cpp.
References contextMenuRequested(), m_mouseDial, and m_mousePressed.
AudioDial::~AudioDial | ( | void | ) |
Definition at line 98 of file AudioDial.cpp.
References m_lastContextMenu, and m_rangeMapper.
Member Function Documentation
|
inline |
Definition at line 71 of file AudioDial.h.
References m_knobColor.
|
inline |
Definition at line 72 of file AudioDial.h.
References m_meterColor.
|
inline |
Definition at line 73 of file AudioDial.h.
References m_mouseDial, and setRangeMapper().
void AudioDial::setRangeMapper | ( | RangeMapper * | mapper | ) |
Definition at line 126 of file AudioDial.cpp.
References m_rangeMapper, and updateMappedValue().
Referenced by getMouseDial(), PluginParameterBox::populate(), and PropertyBox::updatePropertyEditor().
|
inline |
Definition at line 76 of file AudioDial.h.
References m_rangeMapper, and mappedValue().
Referenced by PluginParameterBox::dialChanged(), and PluginParameterBox::spinBoxChanged().
double AudioDial::mappedValue | ( | ) | const |
Definition at line 455 of file AudioDial.cpp.
References m_mappedValue, and m_rangeMapper.
Referenced by PluginParameterBox::dialChanged(), rangeMapper(), and setMappedValue().
|
inline |
Definition at line 79 of file AudioDial.h.
References contextMenuRequested(), drawTick(), edit(), enterEvent(), leaveEvent(), m_defaultValue, mouseDial, mouseDoubleClickEvent(), mouseEntered(), mouseLeft(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), paintEvent(), setDefaultMappedValue(), setDefaultValue(), setKnobColor(), setMappedValue(), setMeterColor(), setMouseDial(), setProvideContextMenu(), setShowToolTip(), setToDefault(), setValue(), and updateMappedValue().
Referenced by setDefaultValue().
void AudioDial::setShowToolTip | ( | bool | show | ) |
Definition at line 440 of file AudioDial.cpp.
References m_noMappedUpdate, m_showTooltip, and updateMappedValue().
Referenced by defaultValue(), PluginParameterBox::populate(), and PropertyBox::updatePropertyEditor().
void AudioDial::setProvideContextMenu | ( | bool | provide | ) |
Definition at line 449 of file AudioDial.cpp.
References m_provideContextMenu.
Referenced by defaultValue().
|
signal |
Referenced by defaultValue(), and enterEvent().
|
signal |
Referenced by defaultValue(), and leaveEvent().
|
slot |
Set the colour of the knob.
The default is to inherit the colour from the widget's palette.
Definition at line 378 of file AudioDial.cpp.
References m_knobColor.
Referenced by defaultValue().
|
slot |
Set the colour of the meter (the highlighted area around the knob that shows the current value).
The default is to inherit the colour from the widget's palette.
Definition at line 385 of file AudioDial.cpp.
References m_meterColor.
Referenced by defaultValue().
|
slot |
Specify that the dial should respond to radial mouse movements in the same way as QDial.
Definition at line 392 of file AudioDial.cpp.
References m_mouseDial, and mouseDial.
Referenced by defaultValue().
|
slot |
Definition at line 398 of file AudioDial.cpp.
References defaultValue(), m_defaultMappedValue, m_defaultValue, and m_rangeMapper.
Referenced by defaultValue(), PluginParameterBox::populate(), and PropertyBox::updatePropertyEditor().
|
slot |
Definition at line 406 of file AudioDial.cpp.
References updateMappedValue().
Referenced by defaultValue(), edit(), PluginParameterBox::populate(), setMappedValue(), setToDefault(), PluginParameterBox::spinBoxChanged(), and PropertyBox::updatePropertyEditor().
|
slot |
Definition at line 412 of file AudioDial.cpp.
References m_defaultMappedValue, m_defaultValue, and m_rangeMapper.
Referenced by defaultValue().
|
slot |
Definition at line 420 of file AudioDial.cpp.
References m_mappedValue, m_noMappedUpdate, m_rangeMapper, mappedValue(), and setValue().
Referenced by defaultValue(), edit(), setToDefault(), and PluginParameterBox::spinBoxChanged().
|
slot |
Definition at line 509 of file AudioDial.cpp.
References m_defaultMappedValue, m_defaultValue, m_rangeMapper, setMappedValue(), and setValue().
Referenced by contextMenuRequested(), defaultValue(), and mousePressEvent().
|
slot |
Definition at line 550 of file AudioDial.cpp.
References m_mappedValue, m_rangeMapper, setMappedValue(), and setValue().
Referenced by contextMenuRequested(), defaultValue(), and mouseDoubleClickEvent().
|
protected |
Definition at line 350 of file AudioDial.cpp.
Referenced by defaultValue(), and paintEvent().
|
overrideprotected |
Definition at line 142 of file AudioDial.cpp.
References AUDIO_DIAL_MAX, AUDIO_DIAL_MIN, AUDIO_DIAL_RANGE, drawTick(), knobColor, m_knobColor, m_meterColor, and meterColor.
Referenced by defaultValue().
|
overrideprotected |
Definition at line 522 of file AudioDial.cpp.
References m_mouseDial, m_mousePressed, m_posMouse, and setToDefault().
Referenced by defaultValue().
|
overrideprotected |
Definition at line 616 of file AudioDial.cpp.
References m_mouseDial, m_mousePressed, and m_posMouse.
Referenced by defaultValue().
|
overrideprotected |
Definition at line 636 of file AudioDial.cpp.
References m_mouseDial, and m_mousePressed.
Referenced by defaultValue().
|
overrideprotected |
!! needs a common base class with Thumbwheel
Definition at line 537 of file AudioDial.cpp.
References edit(), and m_mouseDial.
Referenced by defaultValue().
|
overrideprotected |
Definition at line 646 of file AudioDial.cpp.
References mouseEntered().
Referenced by defaultValue().
|
overrideprotected |
|
protectedslot |
Definition at line 465 of file AudioDial.cpp.
References m_mappedValue, m_noMappedUpdate, m_rangeMapper, m_showTooltip, and m_title.
Referenced by defaultValue(), setRangeMapper(), setShowToolTip(), and setValue().
|
protectedslot |
Definition at line 104 of file AudioDial.cpp.
References MenuTitle::addTitle(), edit(), m_lastContextMenu, m_provideContextMenu, m_title, and setToDefault().
Referenced by AudioDial(), and defaultValue().
Member Data Documentation
|
private |
Definition at line 137 of file AudioDial.h.
Referenced by getKnobColor(), paintEvent(), and setKnobColor().
|
private |
Definition at line 138 of file AudioDial.h.
Referenced by getMeterColor(), paintEvent(), and setMeterColor().
|
private |
Definition at line 140 of file AudioDial.h.
Referenced by defaultValue(), setDefaultMappedValue(), setDefaultValue(), and setToDefault().
|
private |
Definition at line 141 of file AudioDial.h.
Referenced by setDefaultMappedValue(), setDefaultValue(), and setToDefault().
|
private |
Definition at line 142 of file AudioDial.h.
Referenced by edit(), mappedValue(), setMappedValue(), and updateMappedValue().
|
private |
Definition at line 143 of file AudioDial.h.
Referenced by setMappedValue(), setShowToolTip(), and updateMappedValue().
|
private |
Definition at line 146 of file AudioDial.h.
Referenced by AudioDial(), getMouseDial(), mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), and setMouseDial().
|
private |
Definition at line 147 of file AudioDial.h.
Referenced by AudioDial(), mouseMoveEvent(), mousePressEvent(), and mouseReleaseEvent().
|
private |
Definition at line 148 of file AudioDial.h.
Referenced by mouseMoveEvent(), and mousePressEvent().
|
private |
Definition at line 150 of file AudioDial.h.
Referenced by setShowToolTip(), and updateMappedValue().
|
private |
Definition at line 151 of file AudioDial.h.
Referenced by contextMenuRequested(), and setProvideContextMenu().
|
private |
Definition at line 153 of file AudioDial.h.
Referenced by contextMenuRequested(), and updateMappedValue().
|
private |
Definition at line 155 of file AudioDial.h.
Referenced by contextMenuRequested(), and ~AudioDial().
|
private |
Definition at line 157 of file AudioDial.h.
Referenced by edit(), mappedValue(), rangeMapper(), setDefaultMappedValue(), setDefaultValue(), setMappedValue(), setRangeMapper(), setToDefault(), updateMappedValue(), and ~AudioDial().
Property Documentation
|
readwrite |
Definition at line 63 of file AudioDial.h.
Referenced by paintEvent().
|
readwrite |
Definition at line 64 of file AudioDial.h.
Referenced by paintEvent().
|
readwrite |
Definition at line 65 of file AudioDial.h.
Referenced by defaultValue(), and setMouseDial().
The documentation for this class was generated from the following files:
Generated by
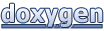