svgui
1.9
|
A dialog for editing the parameters of a given plugin, using a PluginParameterBox. More...
#include <PluginParameterDialog.h>
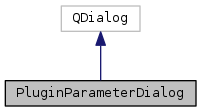
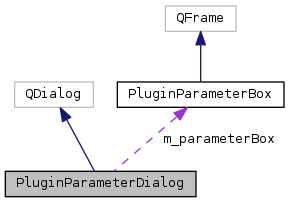
Signals | |
void | pluginConfigurationChanged (QString) |
void | inputModelChanged (QString) |
Public Member Functions | |
PluginParameterDialog (std::shared_ptr< Vamp::PluginBase > plugin, QWidget *parent=0) | |
~PluginParameterDialog () | |
void | setChannelArrangement (int sourceChannels, int targetChannels, int defaultChannel) |
void | setOutputLabel (QString output, QString description) |
void | setMoreInfoUrl (QString url) |
void | setShowProcessingOptions (bool showWindowSize, bool showFrequencyDomainOptions) |
void | setCandidateInputModels (const QStringList &names, QString defaultName) |
void | setShowSelectionOnlyOption (bool show) |
std::shared_ptr< Vamp::PluginBase > | getPlugin () |
int | getChannel () const |
QString | getInputModel () const |
bool | getSelectionOnly () const |
void | getProcessingParameters (int &blockSize) const |
!! merge with PluginTransform::ExecutionContext More... | |
void | getProcessingParameters (int &stepSize, int &blockSize, WindowType &windowType) const |
int | exec () override |
Protected Slots | |
void | channelComboChanged (int) |
void | blockSizeComboChanged (const QString &) |
void | incrementComboChanged (const QString &) |
void | windowTypeChanged (WindowType type) |
void | advancedToggled () |
void | moreInfo () |
void | setAdvancedVisible (bool) |
void | inputModelComboChanged (int) |
void | selectionOnlyChanged (int) |
void | dialogAccepted () |
Protected Attributes | |
std::shared_ptr< Vamp::PluginBase > | m_plugin |
int | m_channel |
int | m_stepSize |
int | m_blockSize |
WindowType | m_windowType |
PluginParameterBox * | m_parameterBox |
QLabel * | m_outputLabel |
QLabel * | m_outputValue |
QLabel * | m_outputDescription |
QLabel * | m_outputSpacer |
QPushButton * | m_moreInfo |
QString | m_moreInfoUrl |
QGroupBox * | m_channelBox |
bool | m_haveChannelBoxData |
QGroupBox * | m_windowBox |
bool | m_haveWindowBoxData |
QGroupBox * | m_inputModelBox |
QComboBox * | m_inputModels |
QCheckBox * | m_selectionOnly |
QStringList | m_inputModelList |
QString | m_currentInputModel |
bool | m_currentSelectionOnly |
QPushButton * | m_advancedButton |
QWidget * | m_advanced |
bool | m_advancedVisible |
Detailed Description
A dialog for editing the parameters of a given plugin, using a PluginParameterBox.
This dialog does not contain any mechanism for selecting the plugin in the first place. Note that the dialog directly modifies the parameters of the plugin, so they will remain modified even if the dialog is then cancelled.
Definition at line 43 of file PluginParameterDialog.h.
Constructor & Destructor Documentation
PluginParameterDialog::PluginParameterDialog | ( | std::shared_ptr< Vamp::PluginBase > | plugin, |
QWidget * | parent = 0 |
||
) |
Definition at line 46 of file PluginParameterDialog.cpp.
References advancedToggled(), dialogAccepted(), m_advanced, m_advancedButton, m_advancedVisible, m_channelBox, m_haveChannelBoxData, m_haveWindowBoxData, m_inputModelBox, m_inputModels, m_moreInfo, m_outputDescription, m_outputLabel, m_outputSpacer, m_outputValue, m_parameterBox, m_plugin, m_selectionOnly, m_windowBox, moreInfo(), pluginConfigurationChanged(), WidgetScale::scaleQSize(), and setAdvancedVisible().
PluginParameterDialog::~PluginParameterDialog | ( | ) |
Definition at line 247 of file PluginParameterDialog.cpp.
Member Function Documentation
void PluginParameterDialog::setChannelArrangement | ( | int | sourceChannels, |
int | targetChannels, | ||
int | defaultChannel | ||
) |
Definition at line 297 of file PluginParameterDialog.cpp.
References channelComboChanged(), m_advancedButton, m_advancedVisible, m_channel, m_channelBox, m_haveChannelBoxData, and setAdvancedVisible().
void PluginParameterDialog::setOutputLabel | ( | QString | output, |
QString | description | ||
) |
Definition at line 261 of file PluginParameterDialog.cpp.
References m_outputDescription, m_outputLabel, m_outputSpacer, and m_outputValue.
void PluginParameterDialog::setMoreInfoUrl | ( | QString | url | ) |
Definition at line 286 of file PluginParameterDialog.cpp.
References m_moreInfo, and m_moreInfoUrl.
void PluginParameterDialog::setShowProcessingOptions | ( | bool | showWindowSize, |
bool | showFrequencyDomainOptions | ||
) |
Definition at line 361 of file PluginParameterDialog.cpp.
References blockSizeComboChanged(), incrementComboChanged(), m_advancedButton, m_advancedVisible, m_haveWindowBoxData, m_plugin, m_windowBox, setAdvancedVisible(), and windowTypeChanged().
void PluginParameterDialog::setCandidateInputModels | ( | const QStringList & | names, |
QString | defaultName | ||
) |
Definition at line 459 of file PluginParameterDialog.cpp.
References TextAbbrev::abbreviate(), inputModelComboChanged(), m_currentInputModel, m_inputModelBox, m_inputModelList, and m_inputModels.
void PluginParameterDialog::setShowSelectionOnlyOption | ( | bool | show | ) |
Definition at line 493 of file PluginParameterDialog.cpp.
References m_currentSelectionOnly, m_inputModelBox, m_inputModels, m_selectionOnly, and selectionOnlyChanged().
|
inline |
Definition at line 67 of file PluginParameterDialog.h.
References m_plugin.
|
inline |
Definition at line 69 of file PluginParameterDialog.h.
References advancedToggled(), blockSizeComboChanged(), channelComboChanged(), dialogAccepted(), exec(), getInputModel(), getProcessingParameters(), getSelectionOnly(), incrementComboChanged(), inputModelChanged(), inputModelComboChanged(), m_channel, moreInfo(), pluginConfigurationChanged(), selectionOnlyChanged(), setAdvancedVisible(), and windowTypeChanged().
QString PluginParameterDialog::getInputModel | ( | ) | const |
Definition at line 517 of file PluginParameterDialog.cpp.
References m_currentInputModel.
Referenced by dialogAccepted(), and getChannel().
bool PluginParameterDialog::getSelectionOnly | ( | ) | const |
Definition at line 523 of file PluginParameterDialog.cpp.
References m_currentSelectionOnly.
Referenced by dialogAccepted(), and getChannel().
void PluginParameterDialog::getProcessingParameters | ( | int & | blockSize | ) | const |
!! merge with PluginTransform::ExecutionContext
Definition at line 529 of file PluginParameterDialog.cpp.
References m_blockSize.
Referenced by getChannel().
void PluginParameterDialog::getProcessingParameters | ( | int & | stepSize, |
int & | blockSize, | ||
WindowType & | windowType | ||
) | const |
Definition at line 536 of file PluginParameterDialog.cpp.
References m_blockSize, m_stepSize, and m_windowType.
|
override |
Definition at line 252 of file PluginParameterDialog.cpp.
References m_advancedVisible, and setAdvancedVisible().
Referenced by getChannel().
|
signal |
Referenced by getChannel(), and PluginParameterDialog().
|
signal |
Referenced by getChannel(), and inputModelComboChanged().
|
protectedslot |
Definition at line 605 of file PluginParameterDialog.cpp.
References m_channel.
Referenced by getChannel(), and setChannelArrangement().
|
protectedslot |
Definition at line 547 of file PluginParameterDialog.cpp.
References m_blockSize.
Referenced by getChannel(), and setShowProcessingOptions().
|
protectedslot |
!! rename increment to step size throughout
Definition at line 554 of file PluginParameterDialog.cpp.
References m_stepSize.
Referenced by getChannel(), and setShowProcessingOptions().
|
protectedslot |
Definition at line 562 of file PluginParameterDialog.cpp.
References m_windowType.
Referenced by getChannel(), and setShowProcessingOptions().
|
protectedslot |
Definition at line 576 of file PluginParameterDialog.cpp.
References m_advancedVisible, and setAdvancedVisible().
Referenced by getChannel(), and PluginParameterDialog().
|
protectedslot |
Definition at line 568 of file PluginParameterDialog.cpp.
References m_moreInfoUrl.
Referenced by getChannel(), and PluginParameterDialog().
|
protectedslot |
Definition at line 582 of file PluginParameterDialog.cpp.
References m_advanced, m_advancedButton, and m_advancedVisible.
Referenced by advancedToggled(), exec(), getChannel(), PluginParameterDialog(), setChannelArrangement(), and setShowProcessingOptions().
|
protectedslot |
Definition at line 611 of file PluginParameterDialog.cpp.
References inputModelChanged(), m_currentInputModel, and m_inputModelList.
Referenced by getChannel(), and setCandidateInputModels().
|
protectedslot |
Definition at line 619 of file PluginParameterDialog.cpp.
References m_currentSelectionOnly.
Referenced by getChannel(), and setShowSelectionOnlyOption().
|
protectedslot |
Definition at line 629 of file PluginParameterDialog.cpp.
References getInputModel(), getSelectionOnly(), m_inputModels, and m_selectionOnly.
Referenced by getChannel(), and PluginParameterDialog().
Member Data Documentation
|
protected |
Definition at line 99 of file PluginParameterDialog.h.
Referenced by getPlugin(), PluginParameterDialog(), and setShowProcessingOptions().
|
protected |
Definition at line 101 of file PluginParameterDialog.h.
Referenced by channelComboChanged(), getChannel(), and setChannelArrangement().
|
protected |
Definition at line 102 of file PluginParameterDialog.h.
Referenced by getProcessingParameters(), and incrementComboChanged().
|
protected |
Definition at line 103 of file PluginParameterDialog.h.
Referenced by blockSizeComboChanged(), and getProcessingParameters().
|
protected |
Definition at line 105 of file PluginParameterDialog.h.
Referenced by getProcessingParameters(), and windowTypeChanged().
|
protected |
Definition at line 106 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog().
|
protected |
Definition at line 108 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setOutputLabel().
|
protected |
Definition at line 109 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setOutputLabel().
|
protected |
Definition at line 110 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setOutputLabel().
|
protected |
Definition at line 111 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setOutputLabel().
|
protected |
Definition at line 113 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setMoreInfoUrl().
|
protected |
Definition at line 114 of file PluginParameterDialog.h.
Referenced by moreInfo(), and setMoreInfoUrl().
|
protected |
Definition at line 116 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setChannelArrangement().
|
protected |
Definition at line 117 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setChannelArrangement().
|
protected |
Definition at line 119 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setShowProcessingOptions().
|
protected |
Definition at line 120 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setShowProcessingOptions().
|
protected |
Definition at line 122 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), setCandidateInputModels(), and setShowSelectionOnlyOption().
|
protected |
Definition at line 123 of file PluginParameterDialog.h.
Referenced by dialogAccepted(), PluginParameterDialog(), setCandidateInputModels(), and setShowSelectionOnlyOption().
|
protected |
Definition at line 124 of file PluginParameterDialog.h.
Referenced by dialogAccepted(), PluginParameterDialog(), and setShowSelectionOnlyOption().
|
protected |
Definition at line 125 of file PluginParameterDialog.h.
Referenced by inputModelComboChanged(), and setCandidateInputModels().
|
protected |
Definition at line 126 of file PluginParameterDialog.h.
Referenced by getInputModel(), inputModelComboChanged(), and setCandidateInputModels().
|
protected |
Definition at line 127 of file PluginParameterDialog.h.
Referenced by getSelectionOnly(), selectionOnlyChanged(), and setShowSelectionOnlyOption().
|
protected |
Definition at line 129 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), setAdvancedVisible(), setChannelArrangement(), and setShowProcessingOptions().
|
protected |
Definition at line 130 of file PluginParameterDialog.h.
Referenced by PluginParameterDialog(), and setAdvancedVisible().
|
protected |
Definition at line 131 of file PluginParameterDialog.h.
Referenced by advancedToggled(), exec(), PluginParameterDialog(), setAdvancedVisible(), setChannelArrangement(), and setShowProcessingOptions().
The documentation for this class was generated from the following files:
Generated by
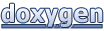