svcore
1.9
|
PlayParameterRepository.h
Go to the documentation of this file.
std::shared_ptr< PlayParameters > getPlayParameters(int id)
Retrieve the play parameters for a playable.
Definition: PlayParameterRepository.cpp:84
static PlayParameterRepository * getInstance()
Definition: PlayParameterRepository.cpp:26
EditCommand(std::shared_ptr< PlayParameters > params)
Definition: PlayParameterRepository.cpp:122
void setPlayClipId(QString)
Definition: PlayParameterRepository.cpp:154
void execute() override
Definition: PlayParameterRepository.cpp:160
QString getName() const override
Definition: PlayParameterRepository.cpp:172
void setPlayMuted(bool)
Definition: PlayParameterRepository.cpp:130
void setPlayPan(float)
Definition: PlayParameterRepository.cpp:142
static PlayParameterRepository * m_instance
Definition: PlayParameterRepository.h:94
PlayParameters m_from
Definition: PlayParameterRepository.h:78
virtual ~PlayParameterRepository()
Definition: PlayParameterRepository.cpp:31
void setPlayAudible(bool)
Definition: PlayParameterRepository.cpp:136
PlayParameters m_to
Definition: PlayParameterRepository.h:79
void playParametersChanged()
Definition: PlayParameterRepository.cpp:93
std::map< int, std::shared_ptr< PlayParameters > > PlayableParameterMap
Definition: PlayParameterRepository.h:91
PlayableParameterMap m_playParameters
Definition: PlayParameterRepository.h:92
std::shared_ptr< PlayParameters > m_params
Definition: PlayParameterRepository.h:77
void copyParameters(int fromId, int toId)
Copy the play parameters from one playable to another.
Definition: PlayParameterRepository.cpp:70
Definition: PlayParameters.h:23
Definition: Playable.h:21
void addPlayable(int id, const Playable *)
Register a playable.
Definition: PlayParameterRepository.cpp:36
Definition: Command.h:26
void setPlayGain(float)
Definition: PlayParameterRepository.cpp:148
void unexecute() override
Definition: PlayParameterRepository.cpp:166
void playClipIdChanged(int playableId, QString)
Generated by
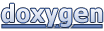