svcore
1.9
|
Clipboard.h
Go to the documentation of this file.
Definition: Clipboard.h:23
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
Generated by
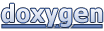