SonicVisualiser
1.9
|
#include <MainWindow.h>
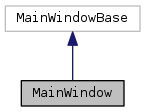
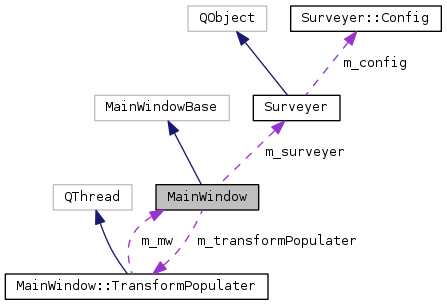
Classes | |
struct | LayerConfiguration |
class | TransformPopulater |
Public Slots | |
void | preferenceChanged (PropertyContainer::PropertyName) override |
virtual void | coloursChanged () |
virtual bool | commitData (bool mayAskUser) |
void | goFullScreen () |
void | endFullScreen () |
Signals | |
void | canChangeSolo (bool) |
void | canAlign (bool) |
Public Member Functions | |
MainWindow (AudioMode audioMode, MIDIMode midiMode, bool withOSCSupport) | |
virtual | ~MainWindow () |
Protected Types | |
typedef std::vector< std::pair< QAction *, LayerConfiguration > > | PaneActions |
typedef std::vector< std::pair< QAction *, LayerConfiguration > > | LayerActions |
typedef std::vector< std::pair< QAction *, Layer * > > | ExistingLayerActions |
typedef std::vector< std::pair< ViewManager::ToolMode, QAction * > > | ToolActions |
typedef std::vector< std::pair< QAction *, int > > | NumberingActions |
typedef std::vector< std::pair< QAction *, TransformId > > | TransformActions |
typedef std::map< TransformId, QAction * > | TransformActionReverseMap |
Protected Slots | |
virtual void | importAudio () |
virtual void | importMoreAudio () |
virtual void | replaceMainAudio () |
virtual void | openSomething () |
virtual void | openLocation () |
virtual void | openRecentFile () |
virtual void | applyTemplate () |
virtual void | exportAudio () |
virtual void | exportAudioData () |
virtual void | convertAudio () |
virtual void | importLayer () |
virtual void | exportLayer () |
virtual void | exportImage () |
virtual void | exportSVG () |
virtual void | browseRecordedAudio () |
virtual void | saveSession () |
virtual void | saveSessionAs () |
virtual void | newSession () |
void | closeSession () override |
virtual void | preferences () |
void | sampleRateMismatch (sv_samplerate_t, sv_samplerate_t, bool) override |
void | audioOverloadPluginDisabled () override |
virtual void | toolNavigateSelected () |
virtual void | toolSelectSelected () |
virtual void | toolEditSelected () |
virtual void | toolDrawSelected () |
virtual void | toolEraseSelected () |
virtual void | toolMeasureSelected () |
void | documentModified () override |
void | documentRestored () override |
virtual void | documentReplaced () |
void | updateMenuStates () override |
void | updateDescriptionLabel () override |
virtual void | setInstantsNumbering () |
virtual void | setInstantsCounterCycle () |
virtual void | setInstantsCounters () |
virtual void | resetInstantsCounters () |
virtual void | subdivideInstants () |
virtual void | winnowInstants () |
void | modelGenerationFailed (QString, QString) override |
void | modelGenerationWarning (QString, QString) override |
void | modelRegenerationFailed (QString, QString, QString) override |
void | modelRegenerationWarning (QString, QString, QString) override |
void | alignmentFailed (ModelId, QString) override |
void | paneRightButtonMenuRequested (Pane *, QPoint point) override |
void | panePropertiesRightButtonMenuRequested (Pane *, QPoint point) override |
void | layerPropertiesRightButtonMenuRequested (Pane *, Layer *, QPoint point) override |
virtual void | propertyStacksResized (int) |
virtual void | addPane () |
virtual void | addLayer () |
virtual void | addLayer (QString transformId) |
virtual void | renameCurrentLayer () |
virtual void | findTransform () |
void | paneAdded (Pane *) override |
void | paneHidden (Pane *) override |
void | paneAboutToBeDeleted (Pane *) override |
void | paneDropAccepted (Pane *, QStringList) override |
void | paneDropAccepted (Pane *, QString) override |
void | paneCancelButtonPressed (Layer *) |
virtual void | setupRecentFilesMenu () |
virtual void | setupRecentTransformsMenu () |
virtual void | setupTemplatesMenu () |
virtual void | playSpeedChanged (int) |
void | playSoloToggled () override |
virtual void | alignToggled () |
void | currentPaneChanged (Pane *) override |
virtual void | speedUpPlayback () |
virtual void | slowDownPlayback () |
virtual void | restoreNormalPlayback () |
void | monitoringLevelsChanged (float, float) override |
void | layerRemoved (Layer *) override |
void | layerInAView (Layer *, bool) override |
void | mainModelChanged (ModelId) override |
virtual void | mainModelGainChanged (float) |
virtual void | mainModelPanChanged (float) |
void | modelAdded (ModelId) override |
virtual void | showLayerTree () |
virtual void | showActivityLog () |
virtual void | showUnitConverter () |
virtual void | mouseEnteredWidget () |
virtual void | mouseLeftWidget () |
void | handleOSCMessage (const OSCMessage &) override |
virtual void | midiEventsAvailable () |
virtual void | playStatusChanged (bool) |
void | populateTransformsMenu () |
virtual void | betaReleaseWarning () |
virtual void | pluginPopulationWarning (QString text) |
virtual void | saveSessionAsTemplate () |
virtual void | manageSavedTemplates () |
virtual void | website () |
virtual void | help () |
virtual void | about () |
virtual void | whatsNew () |
virtual void | keyReference () |
void | newerVersionAvailable (QString) override |
Protected Member Functions | |
QString | shortcutFor (LayerFactory::LayerType, bool isPaneMenu) |
void | updateLayerShortcutsFor (ModelId) |
QString | getReleaseText () const |
void | setupMenus () override |
void | setupFileMenu () |
void | setupEditMenu () |
void | setupViewMenu () |
void | setupPaneAndLayerMenus () |
void | prepareTransformsMenu () |
void | setupHelpMenu () |
void | setupExistingLayersMenus () |
void | setupToolbars () |
void | addPane (const LayerConfiguration &configuration, QString text) |
void | closeEvent (QCloseEvent *e) override |
bool | checkSaveModified () override |
void | exportAudio (bool asData) |
void | updateVisibleRangeDisplay (Pane *p) const override |
void | updatePositionStatusDisplays () const override |
bool | shouldCreateNewSessionForRDFAudio (bool *cancel) override |
void | connectLayerEditDialog (ModelDataTableDialog *) override |
Detailed Description
Definition at line 30 of file MainWindow.h.
Member Typedef Documentation
|
protected |
Definition at line 263 of file MainWindow.h.
|
protected |
Definition at line 266 of file MainWindow.h.
|
protected |
Definition at line 269 of file MainWindow.h.
|
protected |
Definition at line 273 of file MainWindow.h.
|
protected |
Definition at line 276 of file MainWindow.h.
|
protected |
Definition at line 279 of file MainWindow.h.
|
protected |
Definition at line 283 of file MainWindow.h.
Constructor & Destructor Documentation
MainWindow::MainWindow | ( | AudioMode | audioMode, |
MIDIMode | midiMode, | ||
bool | withOSCSupport | ||
) |
Definition at line 141 of file MainWindow.cpp.
References Surveyer::Config::acceptLabel, coloursChanged(), Surveyer::Config::countdownFrom, Surveyer::Config::countdownKey, documentReplaced(), getReleaseText(), NetworkPermissionTester::havePermission(), Surveyer::Config::hostname, Surveyer::Config::includeSystemInfo, m_activityLog, m_currentLabel, m_descriptionLabel, m_mainLevelPan, m_mainScroll, m_overview, m_panLayer, m_playControlsSpacer, m_playControlsWidth, m_playSpeed, m_surveyer, m_unitConverter, m_versionTester, midiEventsAvailable(), mouseEnteredWidget(), mouseLeftWidget(), newerVersionAvailable(), newSession(), playSpeedChanged(), propertyStacksResized(), Surveyer::Config::rejectLabel, setupHelpMenu(), setupMenus(), setupToolbars(), Surveyer::Config::surveyPath, Surveyer::Config::testPath, Surveyer::Config::text, and Surveyer::Config::title.
|
virtual |
Definition at line 391 of file MainWindow.cpp.
References m_activityLog, m_keyReference, m_layerTreeDialog, m_preferencesDialog, m_surveyer, m_transformPopulater, m_unitConverter, and m_versionTester.
Member Function Documentation
|
signal |
Referenced by alignToggled(), setupToolbars(), and updateMenuStates().
|
signal |
Referenced by setupToolbars(), and updateMenuStates().
|
overrideslot |
|
virtualslot |
Definition at line 4052 of file MainWindow.cpp.
References m_panLayer.
Referenced by MainWindow(), preferenceChanged(), and preferences().
|
virtualslot |
Definition at line 3878 of file MainWindow.cpp.
References checkSaveModified(), and m_preferencesDialog.
Referenced by SVApplication::commitData().
|
slot |
Definition at line 474 of file MainWindow.cpp.
References endFullScreen(), m_mainScroll, m_playAction, m_scrollLeftAction, m_scrollRightAction, m_showPropertyBoxesAction, m_zoomFitAction, m_zoomInAction, and m_zoomOutAction.
Referenced by setupViewMenu().
|
slot |
Definition at line 506 of file MainWindow.cpp.
References m_mainScroll.
Referenced by goFullScreen().
|
protectedvirtualslot |
Definition at line 2781 of file MainWindow.cpp.
|
protectedvirtualslot |
Definition at line 2795 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 2809 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3545 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3569 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3601 of file MainWindow.cpp.
Referenced by setupRecentFilesMenu().
|
protectedvirtualslot |
Definition at line 3634 of file MainWindow.cpp.
Referenced by setupTemplatesMenu().
|
protectedvirtualslot |
Definition at line 2823 of file MainWindow.cpp.
Referenced by exportAudioData(), and setupFileMenu().
|
protectedvirtualslot |
Definition at line 2829 of file MainWindow.cpp.
References exportAudio().
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3046 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3111 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3147 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3286 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3374 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3441 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 4000 of file MainWindow.cpp.
References documentRestored(), and saveSessionAs().
Referenced by checkSaveModified(), and setupFileMenu().
|
protectedvirtualslot |
Definition at line 4016 of file MainWindow.cpp.
References documentRestored().
Referenced by saveSession(), and setupFileMenu().
|
protectedvirtualslot |
Definition at line 3451 of file MainWindow.cpp.
References checkSaveModified(), closeSession(), documentRestored(), m_overview, and updateMenuStates().
Referenced by MainWindow(), setupFileMenu(), and shouldCreateNewSessionForRDFAudio().
|
overrideprotectedslot |
Definition at line 3492 of file MainWindow.cpp.
References checkSaveModified(), documentRestored(), m_activityLog, m_keyReference, m_layerTreeDialog, m_overview, m_preferencesDialog, and m_unitConverter.
Referenced by closeEvent(), and newSession().
|
protectedvirtualslot |
Definition at line 5267 of file MainWindow.cpp.
References coloursChanged(), m_preferencesDialog, and PreferencesDialog::TemplateTab.
Referenced by setupFileMenu(), and setupTemplatesMenu().
|
overrideprotectedslot |
Definition at line 4796 of file MainWindow.cpp.
References updateDescriptionLabel().
|
overrideprotectedslot |
Definition at line 4812 of file MainWindow.cpp.
|
protectedvirtualslot |
Definition at line 2745 of file MainWindow.cpp.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 2751 of file MainWindow.cpp.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 2757 of file MainWindow.cpp.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 2763 of file MainWindow.cpp.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 2769 of file MainWindow.cpp.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 2775 of file MainWindow.cpp.
Referenced by setupToolbars().
|
overrideprotectedslot |
!!
Definition at line 2731 of file MainWindow.cpp.
|
overrideprotectedslot |
!!
Definition at line 2738 of file MainWindow.cpp.
Referenced by closeSession(), newSession(), saveSession(), and saveSessionAs().
|
protectedvirtualslot |
Definition at line 3483 of file MainWindow.cpp.
References m_activityLog.
Referenced by MainWindow().
|
overrideprotectedslot |
Definition at line 2625 of file MainWindow.cpp.
References canAlign(), canChangeSolo(), m_deleteSelectedAction, m_ffwdAction, m_playSpeed, and m_rwdAction.
Referenced by addLayer(), addPane(), newSession(), paneCancelButtonPressed(), playSpeedChanged(), and populateTransformsMenu().
|
overrideprotectedslot |
!!???
Definition at line 2701 of file MainWindow.cpp.
References m_descriptionLabel.
Referenced by sampleRateMismatch().
|
protectedvirtualslot |
Definition at line 5005 of file MainWindow.cpp.
References m_numberingActions.
Referenced by setupEditMenu().
|
protectedvirtualslot |
Definition at line 5024 of file MainWindow.cpp.
Referenced by setupEditMenu().
|
protectedvirtualslot |
Definition at line 5041 of file MainWindow.cpp.
Referenced by setupEditMenu().
|
protectedvirtualslot |
Definition at line 5049 of file MainWindow.cpp.
Referenced by setupEditMenu().
|
protectedvirtualslot |
Definition at line 5055 of file MainWindow.cpp.
Referenced by setupEditMenu().
|
protectedvirtualslot |
Definition at line 5077 of file MainWindow.cpp.
Referenced by setupEditMenu().
|
overrideprotectedslot |
Definition at line 5099 of file MainWindow.cpp.
|
overrideprotectedslot |
Definition at line 5127 of file MainWindow.cpp.
|
overrideprotectedslot |
Definition at line 5136 of file MainWindow.cpp.
|
overrideprotectedslot |
Definition at line 5160 of file MainWindow.cpp.
|
overrideprotectedslot |
Definition at line 5171 of file MainWindow.cpp.
|
overrideprotectedslot |
Definition at line 5182 of file MainWindow.cpp.
References m_rightButtonMenu.
|
overrideprotectedslot |
Definition at line 5189 of file MainWindow.cpp.
References m_lastRightButtonPropertyMenu.
|
overrideprotectedslot |
Definition at line 5210 of file MainWindow.cpp.
References m_lastRightButtonPropertyMenu, and renameCurrentLayer().
|
protectedvirtualslot |
Definition at line 4083 of file MainWindow.cpp.
References m_playControlsSpacer, and m_playControlsWidth.
Referenced by MainWindow().
|
protectedvirtualslot |
Definition at line 4097 of file MainWindow.cpp.
References m_paneActions.
Referenced by handleOSCMessage(), and setupPaneAndLayerMenus().
|
protectedvirtualslot |
!!???
!! want to do something like this, but it's not supported in
Definition at line 4204 of file MainWindow.cpp.
References m_existingLayerActions, m_layerActions, m_sliceActions, m_toolActions, and m_transformActions.
Referenced by findTransform(), populateTransformsMenu(), setupExistingLayersMenus(), and setupPaneAndLayerMenus().
|
protectedvirtualslot |
Definition at line 4380 of file MainWindow.cpp.
References updateMenuStates().
|
protectedvirtualslot |
Definition at line 4499 of file MainWindow.cpp.
References setupExistingLayersMenus().
Referenced by layerPropertiesRightButtonMenuRequested(), and setupPaneAndLayerMenus().
|
protectedvirtualslot |
Definition at line 4531 of file MainWindow.cpp.
References addLayer().
Referenced by populateTransformsMenu().
|
overrideprotectedslot |
Definition at line 3724 of file MainWindow.cpp.
References m_overview, and paneCancelButtonPressed().
|
overrideprotectedslot |
Definition at line 3734 of file MainWindow.cpp.
References m_overview.
|
overrideprotectedslot |
Definition at line 3740 of file MainWindow.cpp.
References m_overview.
|
overrideprotectedslot |
Definition at line 3789 of file MainWindow.cpp.
Referenced by paneDropAccepted().
|
overrideprotectedslot |
!! open as text – but by importing as if a CSV, or just adding
Definition at line 3820 of file MainWindow.cpp.
References paneDropAccepted().
|
protectedslot |
Definition at line 3746 of file MainWindow.cpp.
References updateMenuStates().
Referenced by paneAdded().
|
protectedvirtualslot |
Definition at line 2069 of file MainWindow.cpp.
References m_keyReference, m_recentFilesMenu, and openRecentFile().
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 2142 of file MainWindow.cpp.
References m_keyReference, m_recentTransformsMenu, and m_transformActionsReverse.
Referenced by populateTransformsMenu().
|
protectedvirtualslot |
Definition at line 2091 of file MainWindow.cpp.
References applyTemplate(), m_manageTemplatesAction, m_templatesMenu, m_templateWatcher, and preferences().
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 4597 of file MainWindow.cpp.
References m_playSpeed, and updateMenuStates().
Referenced by MainWindow().
|
overrideprotectedslot |
Definition at line 4547 of file MainWindow.cpp.
References m_soloModified.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 4554 of file MainWindow.cpp.
References canChangeSolo(), m_prevSolo, m_soloAction, and m_soloModified.
Referenced by setupToolbars().
|
overrideprotectedslot |
Definition at line 4660 of file MainWindow.cpp.
References m_panLayer, and updateLayerShortcutsFor().
|
protectedvirtualslot |
Definition at line 4636 of file MainWindow.cpp.
References m_playSpeed.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 4645 of file MainWindow.cpp.
References m_playSpeed.
Referenced by setupToolbars().
|
protectedvirtualslot |
Definition at line 4654 of file MainWindow.cpp.
References m_playSpeed.
Referenced by setupToolbars().
|
overrideprotectedslot |
Definition at line 4790 of file MainWindow.cpp.
References m_mainLevelPan.
|
overrideprotectedslot |
Definition at line 4945 of file MainWindow.cpp.
References setupExistingLayersMenus().
|
overrideprotectedslot |
Definition at line 4953 of file MainWindow.cpp.
References setupExistingLayersMenus().
|
overrideprotectedslot |
Definition at line 4969 of file MainWindow.cpp.
References m_mainLevelPan, m_panLayer, mainModelGainChanged(), and mainModelPanChanged().
|
protectedvirtualslot |
Definition at line 4984 of file MainWindow.cpp.
Referenced by mainModelChanged().
|
protectedvirtualslot |
Definition at line 4994 of file MainWindow.cpp.
Referenced by mainModelChanged().
|
overrideprotectedslot |
Definition at line 4960 of file MainWindow.cpp.
References setupPaneAndLayerMenus().
|
protectedvirtualslot |
Definition at line 5238 of file MainWindow.cpp.
References m_layerTreeDialog.
Referenced by setupViewMenu().
|
protectedvirtualslot |
Definition at line 5252 of file MainWindow.cpp.
References m_activityLog.
Referenced by setupViewMenu().
|
protectedvirtualslot |
Definition at line 5260 of file MainWindow.cpp.
References m_unitConverter.
Referenced by setupViewMenu().
|
protectedvirtualslot |
Definition at line 5303 of file MainWindow.cpp.
References m_mainLevelPan, and m_playSpeed.
Referenced by MainWindow().
|
protectedvirtualslot |
Definition at line 5324 of file MainWindow.cpp.
Referenced by MainWindow().
|
overrideprotectedslot |
!! we really need to spin here and not return until the
Definition at line 46 of file OSCHandler.cpp.
References addPane(), m_mainLevelPan, m_playSpeed, and NOW.
|
protectedvirtualslot |
Definition at line 4841 of file MainWindow.cpp.
Referenced by MainWindow().
|
protectedvirtualslot |
Definition at line 4929 of file MainWindow.cpp.
Referenced by setupToolbars().
|
protectedslot |
Definition at line 1749 of file MainWindow.cpp.
References addLayer(), findTransform(), m_keyReference, m_recentTransformsMenu, m_rightButtonTransformsMenu, m_transformActions, m_transformActionsReverse, m_transformsMenu, pluginPopulationWarning(), setupRecentTransformsMenu(), and updateMenuStates().
Referenced by MainWindow::TransformPopulater::run().
|
protectedvirtualslot |
Definition at line 4820 of file MainWindow.cpp.
|
protectedvirtualslot |
Definition at line 4828 of file MainWindow.cpp.
Referenced by populateTransformsMenu().
|
protectedvirtualslot |
Definition at line 3665 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 3717 of file MainWindow.cpp.
Referenced by setupFileMenu().
|
protectedvirtualslot |
Definition at line 5330 of file MainWindow.cpp.
|
protectedvirtualslot |
Definition at line 5336 of file MainWindow.cpp.
Referenced by setupHelpMenu().
|
protectedvirtualslot |
Definition at line 5436 of file MainWindow.cpp.
References getReleaseText().
Referenced by setupHelpMenu().
|
protectedvirtualslot |
Definition at line 5342 of file MainWindow.cpp.
References m_newerVersionIs.
Referenced by setupHelpMenu().
|
protectedvirtualslot |
Definition at line 5615 of file MainWindow.cpp.
References m_keyReference.
Referenced by setupHelpMenu().
|
overrideprotectedslot |
Definition at line 5621 of file MainWindow.cpp.
References m_newerVersionIs.
Referenced by MainWindow().
|
protected |
Definition at line 1181 of file MainWindow.cpp.
Referenced by setupPaneAndLayerMenus(), and updateLayerShortcutsFor().
|
protected |
Definition at line 1668 of file MainWindow.cpp.
References m_layerActions, m_paneActions, and shortcutFor().
Referenced by currentPaneChanged().
|
protected |
Definition at line 5409 of file MainWindow.cpp.
Referenced by about(), and MainWindow().
|
overrideprotected |
Definition at line 412 of file MainWindow.cpp.
References m_mainMenusCreated, m_rightButtonLayerMenu, m_rightButtonMenu, m_rightButtonTransformsMenu, prepareTransformsMenu(), setupEditMenu(), setupFileMenu(), setupPaneAndLayerMenus(), and setupViewMenu().
Referenced by MainWindow().
|
protected |
Definition at line 520 of file MainWindow.cpp.
References browseRecordedAudio(), convertAudio(), exportAudio(), exportAudioData(), exportImage(), exportLayer(), exportSVG(), importLayer(), importMoreAudio(), m_keyReference, m_mainMenusCreated, m_manageTemplatesAction, m_recentFilesMenu, m_templatesMenu, manageSavedTemplates(), newSession(), openLocation(), openSomething(), preferences(), replaceMainAudio(), saveSession(), saveSessionAs(), saveSessionAsTemplate(), setupRecentFilesMenu(), and setupTemplatesMenu().
Referenced by setupMenus().
|
protected |
Definition at line 706 of file MainWindow.cpp.
References m_deleteSelectedAction, m_keyReference, m_mainMenusCreated, m_numberingActions, m_rightButtonMenu, resetInstantsCounters(), setInstantsCounterCycle(), setInstantsCounters(), setInstantsNumbering(), subdivideInstants(), and winnowInstants().
Referenced by setupMenus().
|
protected |
Definition at line 956 of file MainWindow.cpp.
References goFullScreen(), m_keyReference, m_mainMenusCreated, m_scrollLeftAction, m_scrollRightAction, m_showPropertyBoxesAction, m_zoomFitAction, m_zoomInAction, m_zoomOutAction, showActivityLog(), showLayerTree(), and showUnitConverter().
Referenced by setupMenus().
|
protected |
Definition at line 1238 of file MainWindow.cpp.
References addLayer(), addPane(), m_existingLayersMenu, m_keyReference, m_layerActions, m_layerMenu, m_paneActions, m_paneMenu, m_rightButtonLayerMenu, m_rightButtonMenu, m_sliceMenu, renameCurrentLayer(), setupExistingLayersMenus(), and shortcutFor().
Referenced by modelAdded(), and setupMenus().
|
protected |
Definition at line 1706 of file MainWindow.cpp.
References m_transformPopulater, and m_transformsMenu.
Referenced by setupMenus().
|
protected |
Definition at line 2029 of file MainWindow.cpp.
References about(), help(), keyReference(), m_keyReference, and whatsNew().
Referenced by MainWindow().
|
protected |
Definition at line 2171 of file MainWindow.cpp.
References addLayer(), m_existingLayerActions, m_existingLayersMenu, m_sliceActions, and m_sliceMenu.
Referenced by layerInAView(), layerRemoved(), renameCurrentLayer(), and setupPaneAndLayerMenus().
|
protected |
Definition at line 2262 of file MainWindow.cpp.
References alignToggled(), canAlign(), canChangeSolo(), m_ffwdAction, m_ffwdEndAction, m_ffwdSimilarAction, m_keyReference, m_playAction, m_playbackMenu, m_playLoopAction, m_playSelectionAction, m_prevSolo, m_recordAction, m_rightButtonMenu, m_rightButtonPlaybackMenu, m_rwdAction, m_rwdSimilarAction, m_rwdStartAction, m_soloAction, m_toolActions, playSoloToggled(), playStatusChanged(), restoreNormalPlayback(), slowDownPlayback(), speedUpPlayback(), toolDrawSelected(), toolEditSelected(), toolEraseSelected(), toolMeasureSelected(), toolNavigateSelected(), and toolSelectSelected().
Referenced by MainWindow().
|
protected |
Definition at line 4131 of file MainWindow.cpp.
References MainWindow::LayerConfiguration::channel, MainWindow::LayerConfiguration::layer, MainWindow::LayerConfiguration::sourceModel, and updateMenuStates().
|
overrideprotected |
Definition at line 3839 of file MainWindow.cpp.
References checkSaveModified(), closeSession(), and m_preferencesDialog.
|
overrideprotected |
Definition at line 3930 of file MainWindow.cpp.
References saveSession().
Referenced by closeEvent(), closeSession(), commitData(), and newSession().
|
protected |
Definition at line 2835 of file MainWindow.cpp.
|
overrideprotected |
Definition at line 4708 of file MainWindow.cpp.
References updatePositionStatusDisplays().
|
overrideprotected |
Definition at line 4765 of file MainWindow.cpp.
References m_currentLabel.
Referenced by updateVisibleRangeDisplay().
|
overrideprotected |
!! This is very similar to part of MainWindowBase::openAudio – !! make them a bit more uniform
Definition at line 3964 of file MainWindow.cpp.
References newSession().
|
overrideprotected |
Definition at line 2611 of file MainWindow.cpp.
References m_ffwdAction, m_ffwdEndAction, m_playAction, m_rwdAction, and m_rwdStartAction.
Member Data Documentation
|
protected |
Definition at line 175 of file MainWindow.h.
Referenced by closeSession(), MainWindow(), newSession(), paneAboutToBeDeleted(), paneAdded(), and paneHidden().
|
protected |
Definition at line 176 of file MainWindow.h.
Referenced by handleOSCMessage(), mainModelChanged(), MainWindow(), monitoringLevelsChanged(), and mouseEnteredWidget().
|
protected |
Definition at line 177 of file MainWindow.h.
Referenced by handleOSCMessage(), MainWindow(), mouseEnteredWidget(), playSpeedChanged(), restoreNormalPlayback(), slowDownPlayback(), speedUpPlayback(), and updateMenuStates().
|
protected |
Definition at line 178 of file MainWindow.h.
Referenced by coloursChanged(), currentPaneChanged(), mainModelChanged(), and MainWindow().
|
protected |
Definition at line 180 of file MainWindow.h.
Referenced by endFullScreen(), goFullScreen(), and MainWindow().
|
protected |
Definition at line 182 of file MainWindow.h.
Referenced by setupEditMenu(), setupFileMenu(), setupMenus(), and setupViewMenu().
|
protected |
Definition at line 183 of file MainWindow.h.
Referenced by setupPaneAndLayerMenus().
|
protected |
Definition at line 184 of file MainWindow.h.
Referenced by setupPaneAndLayerMenus().
|
protected |
Definition at line 185 of file MainWindow.h.
Referenced by populateTransformsMenu(), and prepareTransformsMenu().
|
protected |
Definition at line 186 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 187 of file MainWindow.h.
Referenced by setupExistingLayersMenus(), and setupPaneAndLayerMenus().
|
protected |
Definition at line 188 of file MainWindow.h.
Referenced by setupExistingLayersMenus(), and setupPaneAndLayerMenus().
|
protected |
Definition at line 189 of file MainWindow.h.
Referenced by setupFileMenu(), and setupRecentFilesMenu().
|
protected |
Definition at line 190 of file MainWindow.h.
Referenced by populateTransformsMenu(), and setupRecentTransformsMenu().
|
protected |
Definition at line 191 of file MainWindow.h.
Referenced by setupFileMenu(), and setupTemplatesMenu().
|
protected |
Definition at line 192 of file MainWindow.h.
Referenced by paneRightButtonMenuRequested(), setupEditMenu(), setupMenus(), setupPaneAndLayerMenus(), and setupToolbars().
|
protected |
Definition at line 193 of file MainWindow.h.
Referenced by setupMenus(), and setupPaneAndLayerMenus().
|
protected |
Definition at line 194 of file MainWindow.h.
Referenced by populateTransformsMenu(), and setupMenus().
|
protected |
Definition at line 195 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 196 of file MainWindow.h.
Referenced by layerPropertiesRightButtonMenuRequested(), and panePropertiesRightButtonMenuRequested().
|
protected |
Definition at line 198 of file MainWindow.h.
Referenced by setupEditMenu(), and updateMenuStates().
|
protected |
Definition at line 199 of file MainWindow.h.
Referenced by alignToggled(), and setupToolbars().
|
protected |
Definition at line 200 of file MainWindow.h.
Referenced by connectLayerEditDialog(), and setupToolbars().
|
protected |
Definition at line 201 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 202 of file MainWindow.h.
Referenced by connectLayerEditDialog(), setupToolbars(), and updateMenuStates().
|
protected |
Definition at line 203 of file MainWindow.h.
Referenced by connectLayerEditDialog(), setupToolbars(), and updateMenuStates().
|
protected |
Definition at line 204 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 205 of file MainWindow.h.
Referenced by connectLayerEditDialog(), and setupToolbars().
|
protected |
Definition at line 206 of file MainWindow.h.
Referenced by connectLayerEditDialog(), goFullScreen(), and setupToolbars().
|
protected |
Definition at line 207 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 208 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 209 of file MainWindow.h.
Referenced by setupToolbars().
|
protected |
Definition at line 210 of file MainWindow.h.
Referenced by setupFileMenu(), and setupTemplatesMenu().
|
protected |
Definition at line 211 of file MainWindow.h.
Referenced by goFullScreen(), and setupViewMenu().
|
protected |
Definition at line 212 of file MainWindow.h.
Referenced by goFullScreen(), and setupViewMenu().
|
protected |
Definition at line 213 of file MainWindow.h.
Referenced by goFullScreen(), and setupViewMenu().
|
protected |
Definition at line 214 of file MainWindow.h.
Referenced by goFullScreen(), and setupViewMenu().
|
protected |
Definition at line 215 of file MainWindow.h.
Referenced by goFullScreen(), and setupViewMenu().
|
protected |
Definition at line 216 of file MainWindow.h.
Referenced by goFullScreen(), and setupViewMenu().
|
protected |
Definition at line 218 of file MainWindow.h.
Referenced by alignToggled(), and playSoloToggled().
|
protected |
Definition at line 219 of file MainWindow.h.
Referenced by alignToggled(), and setupToolbars().
|
protected |
Definition at line 221 of file MainWindow.h.
Referenced by MainWindow(), and propertyStacksResized().
|
protected |
Definition at line 222 of file MainWindow.h.
Referenced by MainWindow(), and propertyStacksResized().
|
protected |
Definition at line 224 of file MainWindow.h.
Referenced by MainWindow(), and updateDescriptionLabel().
|
protected |
Definition at line 225 of file MainWindow.h.
Referenced by MainWindow(), and updatePositionStatusDisplays().
|
protected |
Definition at line 227 of file MainWindow.h.
Referenced by closeEvent(), closeSession(), commitData(), preferences(), and ~MainWindow().
|
protected |
Definition at line 228 of file MainWindow.h.
Referenced by closeSession(), showLayerTree(), and ~MainWindow().
|
protected |
Definition at line 230 of file MainWindow.h.
Referenced by closeSession(), documentReplaced(), MainWindow(), showActivityLog(), and ~MainWindow().
|
protected |
Definition at line 231 of file MainWindow.h.
Referenced by closeSession(), MainWindow(), showUnitConverter(), and ~MainWindow().
|
protected |
Definition at line 232 of file MainWindow.h.
Referenced by closeSession(), keyReference(), populateTransformsMenu(), setupEditMenu(), setupFileMenu(), setupHelpMenu(), setupPaneAndLayerMenus(), setupRecentFilesMenu(), setupRecentTransformsMenu(), setupToolbars(), setupViewMenu(), and ~MainWindow().
|
protected |
Definition at line 234 of file MainWindow.h.
Referenced by setupTemplatesMenu().
|
protected |
Definition at line 236 of file MainWindow.h.
Referenced by MainWindow(), and ~MainWindow().
|
protected |
Definition at line 237 of file MainWindow.h.
Referenced by MainWindow(), and ~MainWindow().
|
protected |
Definition at line 238 of file MainWindow.h.
Referenced by newerVersionAvailable(), and whatsNew().
|
protected |
Definition at line 264 of file MainWindow.h.
Referenced by addPane(), setupPaneAndLayerMenus(), and updateLayerShortcutsFor().
|
protected |
Definition at line 267 of file MainWindow.h.
Referenced by addLayer(), setupPaneAndLayerMenus(), and updateLayerShortcutsFor().
|
protected |
Definition at line 270 of file MainWindow.h.
Referenced by addLayer(), and setupExistingLayersMenus().
|
protected |
Definition at line 271 of file MainWindow.h.
Referenced by addLayer(), and setupExistingLayersMenus().
|
protected |
Definition at line 274 of file MainWindow.h.
Referenced by addLayer(), and setupToolbars().
|
protected |
Definition at line 277 of file MainWindow.h.
Referenced by setInstantsNumbering(), and setupEditMenu().
|
protected |
Definition at line 280 of file MainWindow.h.
Referenced by addLayer(), and populateTransformsMenu().
|
protected |
Definition at line 284 of file MainWindow.h.
Referenced by populateTransformsMenu(), and setupRecentTransformsMenu().
|
protected |
Definition at line 306 of file MainWindow.h.
Referenced by prepareTransformsMenu(), and ~MainWindow().
The documentation for this class was generated from the following files:
Generated by
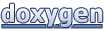