SonicVisualiser
1.9
|
PreferencesDialog.h
Go to the documentation of this file.
void tempDirButtonClicked()
Definition: PreferencesDialog.cpp:879
void colour3DColourChanged(int state)
Definition: PreferencesDialog.cpp:746
void timeToTextModeChanged(int mode)
Definition: PreferencesDialog.cpp:921
virtual ~PreferencesDialog()
Definition: PreferencesDialog.cpp:644
Definition: PreferencesDialog.h:33
int m_spectrogramXSmoothing
Definition: PreferencesDialog.h:117
void overviewColourChanged(int state)
Definition: PreferencesDialog.cpp:754
int m_spectrogramSmoothing
Definition: PreferencesDialog.h:116
void octaveSystemChanged(int system)
Definition: PreferencesDialog.cpp:935
QComboBox * m_audioRecordDeviceCombo
Definition: PreferencesDialog.h:104
QComboBox * m_audioPlaybackDeviceCombo
Definition: PreferencesDialog.h:103
void audioPlaybackDeviceChanged(int device)
Definition: PreferencesDialog.cpp:790
void networkPermissionChanged(int state)
Definition: PreferencesDialog.cpp:833
void showSplashChanged(int state)
Definition: PreferencesDialog.cpp:849
int m_audioPlaybackDevice
Definition: PreferencesDialog.h:126
void rebuildDeviceCombos()
Definition: PreferencesDialog.cpp:650
void propertyLayoutChanged(int layout)
Definition: PreferencesDialog.cpp:765
ColourComboBox * m_overviewColourCombo
Definition: PreferencesDialog.h:102
void audioImplementationChanged(int impl)
Definition: PreferencesDialog.cpp:779
void defaultTemplateChanged(int)
Definition: PreferencesDialog.cpp:857
int m_audioImplementation
Definition: PreferencesDialog.h:125
void windowTypeChanged(WindowType type)
Definition: PreferencesDialog.cpp:709
QLineEdit * m_tempDirRootEdit
Definition: PreferencesDialog.h:100
void spectrogramXSmoothingChanged(int state)
Definition: PreferencesDialog.cpp:723
void coloursChanged()
void viewFontSizeChanged(int sz)
Definition: PreferencesDialog.cpp:942
void spectrogramMColourChanged(int state)
Definition: PreferencesDialog.cpp:738
void spectrogramSmoothingChanged(int state)
Definition: PreferencesDialog.cpp:716
void backgroundModeChanged(int mode)
Definition: PreferencesDialog.cpp:891
void audioRecordDeviceChanged(int device)
Definition: PreferencesDialog.cpp:800
void gaplessModeChanged(int state)
Definition: PreferencesDialog.cpp:818
void resampleOnLoadChanged(int state)
Definition: PreferencesDialog.cpp:810
WindowTypeSelector * m_windowTypeSelector
Definition: PreferencesDialog.h:94
PreferencesDialog(QWidget *parent=0)
Definition: PreferencesDialog.cpp:55
void showHMSChanged(int state)
Definition: PreferencesDialog.cpp:928
void tuningFrequencyChanged(double freq)
Definition: PreferencesDialog.cpp:772
bool m_overviewColourIsSet
Definition: PreferencesDialog.h:121
void vampProcessSeparationChanged(int state)
Definition: PreferencesDialog.cpp:825
void applicationClosing(bool quickly)
Definition: PreferencesDialog.cpp:1072
PluginPathConfigurator * m_pluginPathConfigurator
Definition: PreferencesDialog.h:107
void audioDeviceChanged()
void tempDirRootChanged(QString root)
Definition: PreferencesDialog.cpp:872
void spectrogramGColourChanged(int state)
Definition: PreferencesDialog.cpp:730
bool m_runPluginsInProcess
Definition: PreferencesDialog.h:130
Generated by
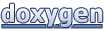