SonicVisualiser
1.9
|
#include <PreferencesDialog.h>
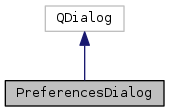
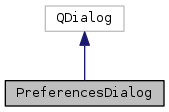
Public Types | |
enum | Tab { GeneralTab, AudioIOTab, AppearanceTab, AnalysisTab, TemplateTab, PluginTab } |
Public Slots | |
void | applicationClosing (bool quickly) |
Signals | |
void | audioDeviceChanged () |
void | coloursChanged () |
Public Member Functions | |
PreferencesDialog (QWidget *parent=0) | |
virtual | ~PreferencesDialog () |
void | switchToTab (Tab tab) |
Protected Member Functions | |
void | rebuildDeviceCombos () |
Detailed Description
Definition at line 33 of file PreferencesDialog.h.
Member Enumeration Documentation
Enumerator | |
---|---|
GeneralTab | |
AudioIOTab | |
AppearanceTab | |
AnalysisTab | |
TemplateTab | |
PluginTab |
Definition at line 41 of file PreferencesDialog.h.
Constructor & Destructor Documentation
PreferencesDialog::PreferencesDialog | ( | QWidget * | parent = 0 | ) |
Definition at line 55 of file PreferencesDialog.cpp.
References AnalysisTab, AppearanceTab, applyClicked(), audioImplementationChanged(), AudioIOTab, audioPlaybackDeviceChanged(), audioRecordDeviceChanged(), backgroundModeChanged(), cancelClicked(), colour3DColourChanged(), defaultTemplateChanged(), gaplessModeChanged(), GeneralTab, localeChanged(), m_applyButton, m_audioDeviceChanged, m_audioImplementation, m_audioPlaybackDeviceCombo, m_audioRecordDeviceCombo, m_backgroundMode, m_colour3DColour, m_currentLocale, m_currentTemplate, m_gapless, m_locales, m_networkPermission, m_octaveSystem, m_overviewColour, m_overviewColourCombo, m_overviewColourIsSet, m_pluginPathConfigurator, m_propertyLayout, m_resampleOnLoad, m_retina, m_runPluginsInProcess, m_showHMS, m_showSplash, m_spectrogramGColour, m_spectrogramMColour, m_spectrogramSmoothing, m_spectrogramXSmoothing, m_tabOrdering, m_tabs, m_tempDirRoot, m_tempDirRootEdit, m_templates, m_timeToTextMode, m_tuningFrequency, m_viewFontSize, m_windowType, m_windowTypeSelector, networkPermissionChanged(), octaveSystemChanged(), okClicked(), overviewColourChanged(), pluginPathsChanged(), PluginTab, propertyLayoutChanged(), rebuildDeviceCombos(), resampleOnLoadChanged(), retinaChanged(), showHMSChanged(), showSplashChanged(), spectrogramGColourChanged(), spectrogramMColourChanged(), spectrogramSmoothingChanged(), spectrogramXSmoothingChanged(), tempDirButtonClicked(), TemplateTab, timeToTextModeChanged(), tuningFrequencyChanged(), vampProcessSeparationChanged(), viewFontSizeChanged(), and windowTypeChanged().
|
virtual |
Definition at line 644 of file PreferencesDialog.cpp.
Member Function Documentation
void PreferencesDialog::switchToTab | ( | Tab | tab | ) |
Definition at line 701 of file PreferencesDialog.cpp.
References m_tabOrdering, and m_tabs.
|
signal |
Referenced by applyClicked().
|
signal |
Referenced by applyClicked().
|
slot |
Definition at line 1072 of file PreferencesDialog.cpp.
References applyClicked(), and m_applyButton.
|
protectedslot |
Definition at line 709 of file PreferencesDialog.cpp.
References m_applyButton, and m_windowType.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 716 of file PreferencesDialog.cpp.
References m_applyButton, and m_spectrogramSmoothing.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 723 of file PreferencesDialog.cpp.
References m_applyButton, and m_spectrogramXSmoothing.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 730 of file PreferencesDialog.cpp.
References m_applyButton, m_coloursChanged, and m_spectrogramGColour.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 738 of file PreferencesDialog.cpp.
References m_applyButton, m_coloursChanged, and m_spectrogramMColour.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 746 of file PreferencesDialog.cpp.
References m_applyButton, m_colour3DColour, and m_coloursChanged.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 754 of file PreferencesDialog.cpp.
References m_applyButton, m_coloursChanged, m_overviewColour, and m_overviewColourIsSet.
Referenced by backgroundModeChanged(), and PreferencesDialog().
|
protectedslot |
Definition at line 765 of file PreferencesDialog.cpp.
References m_applyButton, and m_propertyLayout.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 772 of file PreferencesDialog.cpp.
References m_applyButton, and m_tuningFrequency.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 779 of file PreferencesDialog.cpp.
References m_applyButton, m_audioDeviceChanged, m_audioImplementation, and rebuildDeviceCombos().
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 790 of file PreferencesDialog.cpp.
References m_applyButton, m_audioDeviceChanged, and m_audioPlaybackDevice.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 800 of file PreferencesDialog.cpp.
References m_applyButton, m_audioDeviceChanged, and m_audioRecordDevice.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 810 of file PreferencesDialog.cpp.
References m_applyButton, m_changesOnRestart, and m_resampleOnLoad.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 818 of file PreferencesDialog.cpp.
References m_applyButton, and m_gapless.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 825 of file PreferencesDialog.cpp.
References m_applyButton, m_changesOnRestart, and m_runPluginsInProcess.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 872 of file PreferencesDialog.cpp.
References m_applyButton, and m_tempDirRoot.
Referenced by tempDirButtonClicked().
|
protectedslot |
Definition at line 891 of file PreferencesDialog.cpp.
References m_applyButton, m_backgroundMode, m_changesOnRestart, m_overviewColourCombo, and overviewColourChanged().
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 921 of file PreferencesDialog.cpp.
References m_applyButton, and m_timeToTextMode.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 928 of file PreferencesDialog.cpp.
References m_applyButton, and m_showHMS.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 935 of file PreferencesDialog.cpp.
References m_applyButton, and m_octaveSystem.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 942 of file PreferencesDialog.cpp.
References m_applyButton, and m_viewFontSize.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 849 of file PreferencesDialog.cpp.
References m_applyButton, m_changesOnRestart, and m_showSplash.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 857 of file PreferencesDialog.cpp.
References m_applyButton, m_currentTemplate, and m_templates.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 864 of file PreferencesDialog.cpp.
References m_applyButton, m_changesOnRestart, m_currentLocale, and m_locales.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 833 of file PreferencesDialog.cpp.
References m_applyButton, m_changesOnRestart, and m_networkPermission.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 841 of file PreferencesDialog.cpp.
References m_applyButton, and m_retina.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 949 of file PreferencesDialog.cpp.
References m_applyButton, and m_changesOnRestart.
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 879 of file PreferencesDialog.cpp.
References m_changesOnRestart, m_tempDirRoot, m_tempDirRootEdit, and tempDirRootChanged().
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 956 of file PreferencesDialog.cpp.
References applyClicked().
Referenced by PreferencesDialog().
|
protectedslot |
Definition at line 963 of file PreferencesDialog.cpp.
References audioDeviceChanged(), coloursChanged(), m_applyButton, m_audioDeviceChanged, m_audioImplementation, m_audioPlaybackDevice, m_audioRecordDevice, m_backgroundMode, m_changesOnRestart, m_colour3DColour, m_coloursChanged, m_currentLocale, m_currentTemplate, m_gapless, m_networkPermission, m_octaveSystem, m_overviewColour, m_overviewColourIsSet, m_pluginPathConfigurator, m_propertyLayout, m_resampleOnLoad, m_retina, m_runPluginsInProcess, m_showHMS, m_showSplash, m_spectrogramGColour, m_spectrogramMColour, m_spectrogramSmoothing, m_spectrogramXSmoothing, m_tempDirRoot, m_timeToTextMode, m_tuningFrequency, m_viewFontSize, and m_windowType.
Referenced by applicationClosing(), okClicked(), and PreferencesDialog().
|
protectedslot |
Definition at line 1066 of file PreferencesDialog.cpp.
Referenced by PreferencesDialog().
|
protected |
Definition at line 650 of file PreferencesDialog.cpp.
References m_audioImplementation, m_audioPlaybackDevice, m_audioPlaybackDeviceCombo, m_audioRecordDevice, and m_audioRecordDeviceCombo.
Referenced by audioImplementationChanged(), and PreferencesDialog().
Member Data Documentation
|
protected |
Definition at line 94 of file PreferencesDialog.h.
Referenced by PreferencesDialog().
|
protected |
Definition at line 95 of file PreferencesDialog.h.
Referenced by applicationClosing(), applyClicked(), audioImplementationChanged(), audioPlaybackDeviceChanged(), audioRecordDeviceChanged(), backgroundModeChanged(), colour3DColourChanged(), defaultTemplateChanged(), gaplessModeChanged(), localeChanged(), networkPermissionChanged(), octaveSystemChanged(), overviewColourChanged(), pluginPathsChanged(), PreferencesDialog(), propertyLayoutChanged(), resampleOnLoadChanged(), retinaChanged(), showHMSChanged(), showSplashChanged(), spectrogramGColourChanged(), spectrogramMColourChanged(), spectrogramSmoothingChanged(), spectrogramXSmoothingChanged(), tempDirRootChanged(), timeToTextModeChanged(), tuningFrequencyChanged(), vampProcessSeparationChanged(), viewFontSizeChanged(), and windowTypeChanged().
|
protected |
Definition at line 97 of file PreferencesDialog.h.
Referenced by PreferencesDialog(), and switchToTab().
|
protected |
Definition at line 98 of file PreferencesDialog.h.
Referenced by PreferencesDialog(), and switchToTab().
|
protected |
Definition at line 100 of file PreferencesDialog.h.
Referenced by PreferencesDialog(), and tempDirButtonClicked().
|
protected |
Definition at line 102 of file PreferencesDialog.h.
Referenced by backgroundModeChanged(), and PreferencesDialog().
|
protected |
Definition at line 103 of file PreferencesDialog.h.
Referenced by PreferencesDialog(), and rebuildDeviceCombos().
|
protected |
Definition at line 104 of file PreferencesDialog.h.
Referenced by PreferencesDialog(), and rebuildDeviceCombos().
|
protected |
Definition at line 107 of file PreferencesDialog.h.
Referenced by applyClicked(), and PreferencesDialog().
|
protected |
Definition at line 109 of file PreferencesDialog.h.
Referenced by applyClicked(), defaultTemplateChanged(), and PreferencesDialog().
|
protected |
Definition at line 110 of file PreferencesDialog.h.
Referenced by defaultTemplateChanged(), and PreferencesDialog().
|
protected |
Definition at line 112 of file PreferencesDialog.h.
Referenced by applyClicked(), localeChanged(), and PreferencesDialog().
|
protected |
Definition at line 113 of file PreferencesDialog.h.
Referenced by localeChanged(), and PreferencesDialog().
|
protected |
Definition at line 115 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and windowTypeChanged().
|
protected |
Definition at line 116 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and spectrogramSmoothingChanged().
|
protected |
Definition at line 117 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and spectrogramXSmoothingChanged().
|
protected |
Definition at line 118 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and spectrogramGColourChanged().
|
protected |
Definition at line 119 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and spectrogramMColourChanged().
|
protected |
Definition at line 120 of file PreferencesDialog.h.
Referenced by applyClicked(), colour3DColourChanged(), and PreferencesDialog().
|
protected |
Definition at line 121 of file PreferencesDialog.h.
Referenced by applyClicked(), overviewColourChanged(), and PreferencesDialog().
|
protected |
Definition at line 122 of file PreferencesDialog.h.
Referenced by applyClicked(), overviewColourChanged(), and PreferencesDialog().
|
protected |
Definition at line 123 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and propertyLayoutChanged().
|
protected |
Definition at line 124 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and tuningFrequencyChanged().
|
protected |
Definition at line 125 of file PreferencesDialog.h.
Referenced by applyClicked(), audioImplementationChanged(), PreferencesDialog(), and rebuildDeviceCombos().
|
protected |
Definition at line 126 of file PreferencesDialog.h.
Referenced by applyClicked(), audioPlaybackDeviceChanged(), and rebuildDeviceCombos().
|
protected |
Definition at line 127 of file PreferencesDialog.h.
Referenced by applyClicked(), audioRecordDeviceChanged(), and rebuildDeviceCombos().
|
protected |
Definition at line 128 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and resampleOnLoadChanged().
|
protected |
Definition at line 129 of file PreferencesDialog.h.
Referenced by applyClicked(), gaplessModeChanged(), and PreferencesDialog().
|
protected |
Definition at line 130 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and vampProcessSeparationChanged().
|
protected |
Definition at line 131 of file PreferencesDialog.h.
Referenced by applyClicked(), networkPermissionChanged(), and PreferencesDialog().
|
protected |
Definition at line 132 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and retinaChanged().
|
protected |
Definition at line 133 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), tempDirButtonClicked(), and tempDirRootChanged().
|
protected |
Definition at line 134 of file PreferencesDialog.h.
Referenced by applyClicked(), backgroundModeChanged(), and PreferencesDialog().
|
protected |
Definition at line 135 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and timeToTextModeChanged().
|
protected |
Definition at line 136 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and showHMSChanged().
|
protected |
Definition at line 137 of file PreferencesDialog.h.
Referenced by applyClicked(), octaveSystemChanged(), and PreferencesDialog().
|
protected |
Definition at line 138 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and viewFontSizeChanged().
|
protected |
Definition at line 139 of file PreferencesDialog.h.
Referenced by applyClicked(), PreferencesDialog(), and showSplashChanged().
|
protected |
Definition at line 141 of file PreferencesDialog.h.
Referenced by applyClicked(), audioImplementationChanged(), audioPlaybackDeviceChanged(), audioRecordDeviceChanged(), and PreferencesDialog().
|
protected |
Definition at line 142 of file PreferencesDialog.h.
Referenced by applyClicked(), colour3DColourChanged(), overviewColourChanged(), spectrogramGColourChanged(), and spectrogramMColourChanged().
|
protected |
Definition at line 143 of file PreferencesDialog.h.
Referenced by applyClicked(), backgroundModeChanged(), localeChanged(), networkPermissionChanged(), pluginPathsChanged(), resampleOnLoadChanged(), showSplashChanged(), tempDirButtonClicked(), and vampProcessSeparationChanged().
The documentation for this class was generated from the following files:
Generated by
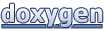