FFmpeg
|
vf_pullup.c
Go to the documentation of this file.
304 sscanf(args, "%d:%d:%d:%d:%d:%d", &c->junk_left, &c->junk_right, &c->junk_top, &c->junk_bottom, &c->strict_breaks, &c->metric_plane);
mp_image_t * ff_vf_get_image(vf_instance_t *vf, unsigned int outfmt, int mp_imgtype, int mp_imgflag, int w, int h)
Definition: vf_mp.c:380
Definition: avisynth_c.h:503
struct pullup_frame * ff_pullup_get_frame(struct pullup_context *c)
Definition: pullup.c:648
int ff_vf_next_config(struct vf_instance *vf, int width, int height, int d_width, int d_height, unsigned int flags, unsigned int outfmt)
Definition: vf_mp.c:584
void ff_pullup_release_frame(struct pullup_frame *fr)
Definition: pullup.c:732
void ff_pullup_pack_frame(struct pullup_context *c, struct pullup_frame *fr)
Definition: pullup.c:714
int(* put_image)(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf.h:68
Definition: pullup.h:53
Definition: pullup.h:34
int(* query_format)(struct vf_instance *vf, unsigned int fmt)
Definition: vf.h:64
static int put_image(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf_pullup.c:107
Definition: vf_dint.c:31
void ff_pullup_submit_field(struct pullup_context *c, struct pullup_buffer *b, int parity)
Definition: pullup.c:416
static void init_pullup(struct vf_instance *vf, mp_image_t *mpi)
Definition: vf_pullup.c:45
static int config(struct vf_instance *vf, int width, int height, int d_width, int d_height, unsigned int flags, unsigned int outfmt)
Definition: vf_pullup.c:269
static int query_format(struct vf_instance *vf, unsigned int fmt)
Definition: vf_pullup.c:257
int(* config)(struct vf_instance *vf, int width, int height, int d_width, int d_height, unsigned int flags, unsigned int outfmt)
Definition: vf.h:59
void ff_pullup_preinit_context(struct pullup_context *c)
Definition: pullup.c:757
struct pullup_context * ff_pullup_alloc_context(void)
Definition: pullup.c:748
void * fast_memcpy(void *to, const void *from, size_t len)
Definition: pullup.h:62
int ff_vf_next_put_image(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf_mp.c:539
void ff_pullup_release_buffer(struct pullup_buffer *b, int parity)
Definition: pullup.c:300
Definition: mp_image.h:125
int ff_vf_next_query_format(struct vf_instance *vf, unsigned int fmt)
Definition: vf_mp.c:371
Definition: vf.h:56
MUSIC TECHNOLOGY GROUP UNIVERSITAT POMPEU FABRA Free Non Commercial Binary License Agreement UNIVERSITAT POMPEU OR INDICATING ACCEPTANCE BY SELECTING THE ACCEPT BUTTON ON ANY DOWNLOAD OR INSTALL YOU ACCEPT THE TERMS OF THE LICENSE SUMMARY TABLE Software MELODIA Melody Extraction vamp plug in Licensor Music Technology Group Universitat Pompeu Plaça de la Spain Permitted purposes Non commercial internal research and validation and educational purposes only All commercial uses in a production either internal or are prohibited by this license and require an additional commercial exploitation license TERMS AND CONDITIONS SOFTWARE Software means the software programs identified herein in binary any other machine readable any updates or error corrections provided by and any user programming guides and other documentation provided to you by UPF under this Agreement LICENSE Subject to the terms and conditions of this UPF grants you a royalty free
Definition: MELODIA - License.txt:16
struct pullup_buffer * ff_pullup_get_buffer(struct pullup_context *c, int parity)
Definition: pullup.c:307
Generated on Thu Jun 19 2025 06:53:16 for FFmpeg by
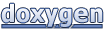