FFmpeg
|
vf.h
Go to the documentation of this file.
118 #define MP_NOPTS_VALUE (-1LL<<63) //both int64_t and double should be able to represent this exactly
123 mp_image_t* ff_vf_get_image(vf_instance_t* vf, unsigned int outfmt, int mp_imgtype, int mp_imgflag, int w, int h);
125 vf_instance_t* vf_open_plugin(const vf_info_t* const* filter_list, vf_instance_t* next, const char *name, char **args);
130 unsigned int ff_vf_match_csp(vf_instance_t** vfp,const unsigned int* list,unsigned int preferred);
143 void ff_vf_next_draw_slice (struct vf_instance *vf, unsigned char** src, int* stride, int w,int h, int x, int y);
vf_instance_t * vf_open_plugin(const vf_info_t *const *filter_list, vf_instance_t *next, const char *name, char **args)
mp_image_t * ff_vf_get_image(vf_instance_t *vf, unsigned int outfmt, int mp_imgtype, int mp_imgflag, int w, int h)
Definition: vf_mp.c:380
Definition: avisynth_c.h:503
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
int ff_vf_next_config(struct vf_instance *vf, int width, int height, int d_width, int d_height, unsigned int flags, unsigned int outfmt)
Definition: vf_mp.c:584
int ff_vf_output_queued_frame(vf_instance_t *vf)
struct vf_info_s vf_info_t
void ff_vf_uninit_filter_chain(vf_instance_t *vf)
int ff_vf_next_control(struct vf_instance *vf, int request, void *data)
Definition: vf_mp.c:613
void ff_vf_extra_flip(struct vf_instance *vf)
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was it was made of draw_slice(that could be called several times on distinct parts of the frame) and end_frame
Definition: vf_dint.c:31
struct vf_seteq_s vf_equalizer_t
int ff_vf_config_wrapper(struct vf_instance *vf, int width, int height, int d_width, int d_height, unsigned int flags, unsigned int outfmt)
void(* start_slice)(struct vf_instance *vf, mp_image_t *mpi)
Definition: vf.h:70
struct vf_image_context_s vf_image_context_t
vf_instance_t * vf_open_encoder(vf_instance_t *next, const char *name, char *args)
struct vf_format_context_t vf_format_context_t
void ff_vf_clone_mpi_attributes(mp_image_t *dst, mp_image_t *src)
Definition: vf_mp.c:293
Definition: vf.h:51
static int put_image(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf_dint.c:82
Definition: vf.h:92
static int config(struct vf_instance *vf, int width, int height, int d_width, int d_height, unsigned int flags, unsigned int outfmt)
Definition: vf_dint.c:45
vf_instance_t * ff_append_filters(vf_instance_t *last)
#define type
void ff_vf_uninit_filter(vf_instance_t *vf)
vf_instance_t * vf_open_filter(vf_instance_t *next, const char *name, char **args)
void ff_vf_next_draw_slice(struct vf_instance *vf, unsigned char **src, int *stride, int w, int h, int x, int y)
Definition: vf_mp.c:304
int ff_vf_next_put_image(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf_mp.c:539
vf_instance_t * ff_vf_add_before_vo(vf_instance_t **vf, char *name, char **args)
void ff_vf_mpi_clear(mp_image_t *mpi, int x0, int y0, int w, int h)
Definition: vf_mp.c:327
struct vf_instance vf_instance_t
int(* continue_buffered_image)(struct vf_instance *vf)
Definition: vf.h:76
unsigned int ff_vf_match_csp(vf_instance_t **vfp, const unsigned int *list, unsigned int preferred)
Definition: vf_mp.c:376
Definition: mp_image.h:125
static int query_format(struct vf_instance *vf, unsigned int fmt)
Definition: vf_down3dright.c:126
Definition: vf.h:43
int ff_vf_next_query_format(struct vf_instance *vf, unsigned int fmt)
Definition: vf_mp.c:371
Definition: vf.h:56
void ff_vf_queue_frame(vf_instance_t *vf, int(*)(vf_instance_t *))
Generated on Thu Jun 19 2025 06:53:15 for FFmpeg by
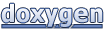