FFmpeg
|
vf_eq.c
Go to the documentation of this file.
static void process_C(unsigned char *dest, int dstride, unsigned char *src, int sstride, int w, int h, int brightness, int contrast)
Definition: vf_eq.c:100
static int put_image(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf_eq.c:128
int(* control)(struct vf_instance *vf, int request, void *data)
Definition: vf.h:62
mp_image_t * ff_vf_get_image(vf_instance_t *vf, unsigned int outfmt, int mp_imgtype, int mp_imgflag, int w, int h)
Definition: vf_mp.c:380
Definition: avisynth_c.h:503
static int query_format(struct vf_instance *vf, unsigned int fmt)
Definition: vf_eq.c:188
int(* put_image)(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf.h:68
static void(* process)(unsigned char *dest, int dstride, unsigned char *src, int sstride, int w, int h, int brightness, int contrast)
Definition: vf_eq.c:123
int(* query_format)(struct vf_instance *vf, unsigned int fmt)
Definition: vf.h:64
int ff_vf_next_control(struct vf_instance *vf, int request, void *data)
Definition: vf_mp.c:613
Definition: vf_dint.c:31
Definition: vf.h:92
int ff_vf_next_put_image(struct vf_instance *vf, mp_image_t *mpi, double pts)
Definition: vf_mp.c:539
Definition: mp_image.h:125
int ff_vf_next_query_format(struct vf_instance *vf, unsigned int fmt)
Definition: vf_mp.c:371
Definition: vf.h:56
MUSIC TECHNOLOGY GROUP UNIVERSITAT POMPEU FABRA Free Non Commercial Binary License Agreement UNIVERSITAT POMPEU OR INDICATING ACCEPTANCE BY SELECTING THE ACCEPT BUTTON ON ANY DOWNLOAD OR INSTALL YOU ACCEPT THE TERMS OF THE LICENSE SUMMARY TABLE Software MELODIA Melody Extraction vamp plug in Licensor Music Technology Group Universitat Pompeu Plaça de la Spain Permitted purposes Non commercial internal research and validation and educational purposes only All commercial uses in a production either internal or are prohibited by this license and require an additional commercial exploitation license TERMS AND CONDITIONS SOFTWARE Software means the software programs identified herein in binary any other machine readable any updates or error corrections provided by and any user programming guides and other documentation provided to you by UPF under this Agreement LICENSE Subject to the terms and conditions of this UPF grants you a royalty free
Definition: MELODIA - License.txt:16
Generated on Mon Nov 18 2024 06:52:02 for FFmpeg by
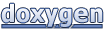