FFmpeg
|
mpegvideodec.c
Go to the documentation of this file.
72 FF_DEF_RAWVIDEO_DEMUXER(mpegvideo, "raw MPEG video", mpegvideo_probe, NULL, AV_CODEC_ID_MPEG1VIDEO)
#define FF_DEF_RAWVIDEO_DEMUXER(shortname, longname, probe, ext, id)
Definition: rawdec.h:52
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
Definition: libavcodec/avcodec.h:103
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
#define AVPROBE_SCORE_MAX
maximum score, half of that is used for file-extension-based detection
Definition: avformat.h:340
Main libavformat public API header.
Generated on Fri Aug 1 2025 06:53:44 for FFmpeg by
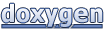