FFmpeg
|
libavcodec/srtenc.c
Go to the documentation of this file.
ASSSplitContext * ff_ass_split(const char *buf)
Split a full ASS file or a ASS header from a string buffer and store the split structure in a newly a...
Definition: ass_split.c:303
static av_cold int srt_encode_init(AVCodecContext *avctx)
Definition: libavcodec/srtenc.c:136
Definition: libavcodec/srtenc.c:31
static int srt_encode_frame(AVCodecContext *avctx, unsigned char *buf, int bufsize, const AVSubtitle *sub)
Definition: libavcodec/srtenc.c:241
text(-8, 1,'a)')
char * text
actual text which will be displayed as a subtitle, can include style override control codes (see ff_a...
Definition: ass_split.h:61
struct SRTContext SRTContext
int alignment
position of the text (left, center, top...), defined after the layout of the numpad (1-3 sub...
Definition: ass_split.h:48
static const float even_table[] __attribute__((aligned(8)))
Definition: ass_split.c:168
static void srt_font_size_cb(void *priv, int size)
Definition: libavcodec/srtenc.c:180
int ff_ass_split_override_codes(const ASSCodesCallbacks *callbacks, void *priv, const char *buf)
Split override codes out of a ASS "Dialogue" Text field.
Definition: ass_split.c:370
static int srt_encode_close(AVCodecContext *avctx)
Definition: libavcodec/srtenc.c:292
Definition: libavcodec/avcodec.h:472
ASSDialog * ff_ass_split_dialog(ASSSplitContext *ctx, const char *buf, int cache, int *number)
Split one or several ASS "Dialogue" lines from a string buffer and store them in a already initialize...
Definition: ass_split.c:338
Definition: libavcodec/avcodec.h:3102
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
external API header
size_t av_strlcpy(char *dst, const char *src, size_t size)
Copy the string src to dst, but no more than size - 1 bytes, and null-terminate dst.
Definition: avstring.c:82
Definition: libavcodec/avcodec.h:464
static void srt_color_cb(void *priv, unsigned int color, unsigned int color_id)
Definition: libavcodec/srtenc.c:163
static void srt_move_cb(void *priv, int x1, int y1, int x2, int y2, int t1, int t2)
Definition: libavcodec/srtenc.c:202
static int srt_stack_push(SRTContext *s, const char c)
Definition: libavcodec/srtenc.c:56
Set of callback functions corresponding to each override codes that can be encountered in a "Dialogue...
Definition: ass_split.h:119
static void srt_style_apply(SRTContext *s, const char *style)
Definition: libavcodec/srtenc.c:97
static void srt_cancel_overrides_cb(void *priv, const char *style)
Definition: libavcodec/srtenc.c:196
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
ASSStyle * ff_ass_style_get(ASSSplitContext *ctx, const char *style)
Find an ASSStyle structure by its name.
Definition: ass_split.c:465
static void srt_style_cb(void *priv, char style, int close)
Definition: libavcodec/srtenc.c:156
static void srt_font_name_cb(void *priv, const char *name)
Definition: libavcodec/srtenc.c:173
void(* text)(void *priv, const char *text, int len)
Definition: ass_split.h:124
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static void srt_new_line_cb(void *priv, int forced)
Definition: libavcodec/srtenc.c:151
Formatted text, the ass field must be set by the decoder and is authoritative.
Definition: libavcodec/avcodec.h:3071
static int srt_stack_find(SRTContext *s, const char c)
Definition: libavcodec/srtenc.c:71
static void srt_print(SRTContext *s, const char *str,...)
Definition: libavcodec/srtenc.c:48
static void srt_alignment_cb(void *priv, int alignment)
Definition: libavcodec/srtenc.c:187
void ff_ass_split_free(ASSSplitContext *ctx)
Free all the memory allocated for an ASSSplitContext.
Definition: ass_split.c:357
static void srt_text_cb(void *priv, const char *text, int len)
Definition: libavcodec/srtenc.c:144
Definition: avutil.h:146
static void srt_stack_push_pop(SRTContext *s, const char c, int close)
Definition: libavcodec/srtenc.c:85
uint8_t * subtitle_header
Header containing style information for text subtitles.
Definition: libavcodec/avcodec.h:2759
Generated on Thu May 29 2025 06:52:53 for FFmpeg by
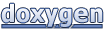