FFmpeg
|
H.264 / AVC / MPEG4 part10 motion vector predicion. More...
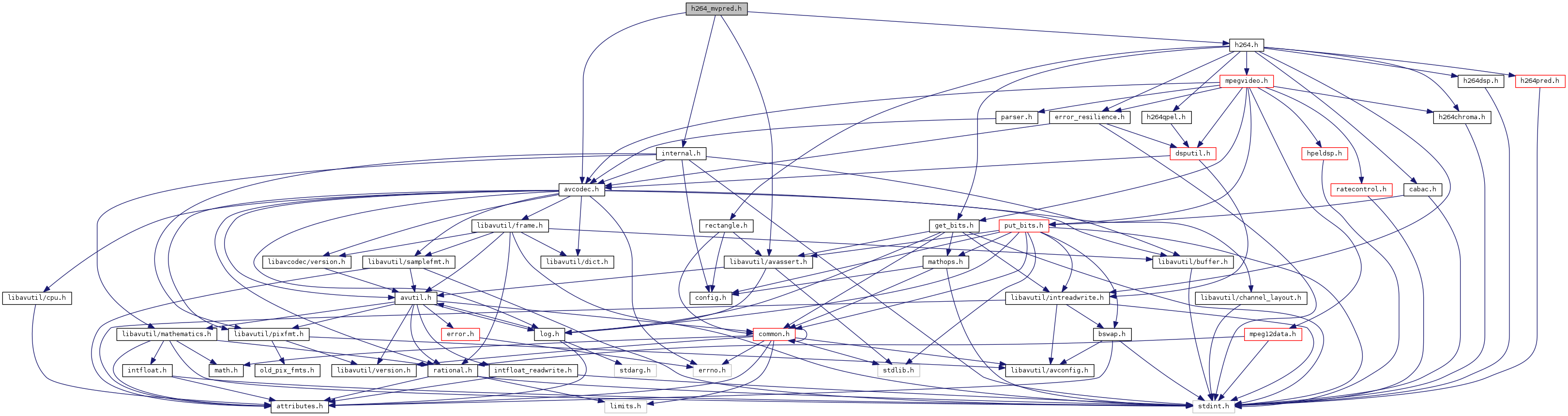
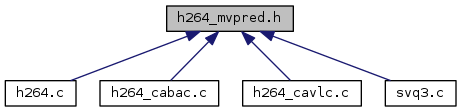
Go to the source code of this file.
Macros | |
#define | SET_DIAG_MV(MV_OP, REF_OP, XY, Y4) |
#define | FIX_MV_MBAFF(type, refn, mvn, idx) |
#define | MAP_MVS |
#define | MAP_F2F(idx, mb_type) |
#define | MAP_F2F(idx, mb_type) |
Functions | |
static av_always_inline int | fetch_diagonal_mv (H264Context *h, const int16_t **C, int i, int list, int part_width) |
static av_always_inline void | pred_motion (H264Context *const h, int n, int part_width, int list, int ref, int *const mx, int *const my) |
Get the predicted MV. More... | |
static av_always_inline void | pred_16x8_motion (H264Context *const h, int n, int list, int ref, int *const mx, int *const my) |
Get the directionally predicted 16x8 MV. More... | |
static av_always_inline void | pred_8x16_motion (H264Context *const h, int n, int list, int ref, int *const mx, int *const my) |
Get the directionally predicted 8x16 MV. More... | |
static av_always_inline void | pred_pskip_motion (H264Context *const h) |
static void | fill_decode_neighbors (H264Context *h, int mb_type) |
static void | fill_decode_caches (H264Context *h, int mb_type) |
static void av_unused | decode_mb_skip (H264Context *h) |
decodes a P_SKIP or B_SKIP macroblock More... | |
Detailed Description
H.264 / AVC / MPEG4 part10 motion vector predicion.
Definition in file h264_mvpred.h.
Macro Definition Documentation
#define FIX_MV_MBAFF | ( | type, | |
refn, | |||
mvn, | |||
idx | |||
) |
Definition at line 232 of file h264_mvpred.h.
Referenced by pred_pskip_motion().
#define MAP_F2F | ( | idx, | |
mb_type | |||
) |
#define MAP_F2F | ( | idx, | |
mb_type | |||
) |
#define MAP_MVS |
Referenced by fill_decode_caches().
#define SET_DIAG_MV | ( | MV_OP, | |
REF_OP, | |||
XY, | |||
Y4 | |||
) |
Referenced by fetch_diagonal_mv().
Function Documentation
|
static |
decodes a P_SKIP or B_SKIP macroblock
Definition at line 796 of file h264_mvpred.h.
Referenced by ff_h264_decode_mb_cabac(), and ff_h264_decode_mb_cavlc().
|
static |
Definition at line 37 of file h264_mvpred.h.
Referenced by pred_8x16_motion(), and pred_motion().
|
static |
Definition at line 438 of file h264_mvpred.h.
Referenced by decode_mb_skip(), ff_h264_decode_mb_cabac(), and ff_h264_decode_mb_cavlc().
|
static |
Definition at line 349 of file h264_mvpred.h.
Referenced by decode_mb_skip(), ff_h264_decode_mb_cabac(), and ff_h264_decode_mb_cavlc().
|
static |
Get the directionally predicted 16x8 MV.
- Parameters
-
n the block index mx the x component of the predicted motion vector my the y component of the predicted motion vector
Definition at line 156 of file h264_mvpred.h.
Referenced by ff_h264_decode_mb_cabac(), and ff_h264_decode_mb_cavlc().
|
static |
Get the directionally predicted 8x16 MV.
- Parameters
-
n the block index mx the x component of the predicted motion vector my the y component of the predicted motion vector
Definition at line 196 of file h264_mvpred.h.
Referenced by ff_h264_decode_mb_cabac(), and ff_h264_decode_mb_cavlc().
|
static |
Get the predicted MV.
- Parameters
-
n the block index part_width the width of the partition (4, 8,16) -> (1, 2, 4) mx the x component of the predicted motion vector my the y component of the predicted motion vector
Definition at line 93 of file h264_mvpred.h.
Referenced by ff_h264_decode_mb_cabac(), ff_h264_decode_mb_cavlc(), pred_16x8_motion(), pred_8x16_motion(), and svq3_mc_dir().
|
static |
Definition at line 251 of file h264_mvpred.h.
Referenced by decode_mb_skip().
Generated on Sun Mar 9 2025 06:53:38 for FFmpeg by
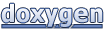