FFmpeg
|
avcodec.c
Go to the documentation of this file.
56 av_log(0, AV_LOG_ERROR, "Layout indicates a different number of channels than actually present\n");
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
AVOptions.
libavcodec/libavfilter gluing utilities
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
void av_frame_set_pkt_pos(AVFrame *frame, int64_t val)
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
void av_frame_set_channels(AVFrame *frame, int val)
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
audio channel layout utility functions
int av_frame_get_channels(const AVFrame *frame)
void av_frame_set_sample_rate(AVFrame *frame, int val)
int format
format of the frame, -1 if unknown or unset Values correspond to enum AVPixelFormat for video frames...
Definition: frame.h:134
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
AVRational sample_aspect_ratio
Sample aspect ratio for the video frame, 0/1 if unknown/unspecified.
Definition: frame.h:154
int av_sample_fmt_is_planar(enum AVSampleFormat sample_fmt)
Check if the sample format is planar.
Definition: samplefmt.c:118
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
int avfilter_copy_frame_props(AVFilterBufferRef *dst, const AVFrame *src)
Definition: libavfilter/buffer.c:118
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel layout
Definition: filter_design.txt:12
int top_field_first
If the content is interlaced, is top field displayed first.
Definition: frame.h:275
void av_frame_set_channel_layout(AVFrame *frame, int64_t val)
int64_t av_frame_get_channel_layout(const AVFrame *frame)
Definition: avutil.h:143
void avfilter_unref_bufferp(AVFilterBufferRef **ref)
Definition: libavfilter/buffer.c:112
Generated on Wed May 7 2025 06:52:38 for FFmpeg by
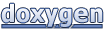