FFmpeg
|
AAC coefficients encoder. More...
#include "libavutil/libm.h"
#include <float.h>
#include "libavutil/mathematics.h"
#include "avcodec.h"
#include "put_bits.h"
#include "aac.h"
#include "aacenc.h"
#include "aactab.h"
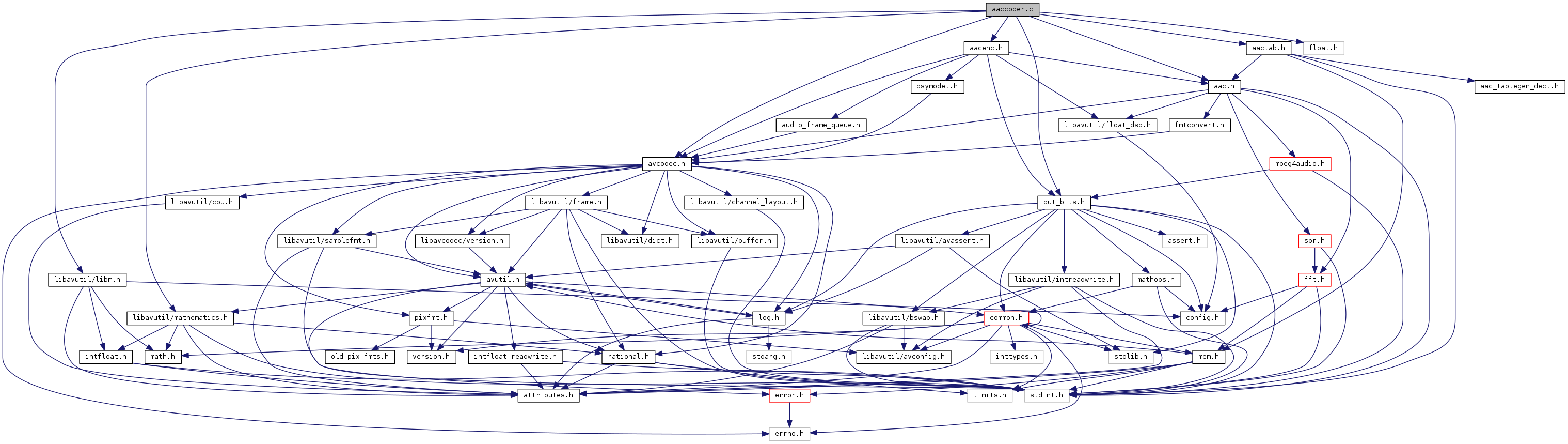
Go to the source code of this file.
Data Structures | |
struct | BandCodingPath |
structure used in optimal codebook search More... | |
struct | TrellisPath |
Macros | |
#define | QUANTIZE_AND_ENCODE_BAND_COST_FUNC(NAME, BT_ZERO, BT_UNSIGNED, BT_PAIR, BT_ESC) |
#define | quantize_and_encode_band_cost(s, pb, in, scaled, size, scale_idx, cb, lambda, uplim, bits) |
#define | TRELLIS_STAGES 121 |
#define | TRELLIS_STATES (SCALE_MAX_DIFF+1) |
Typedefs | |
typedef struct BandCodingPath | BandCodingPath |
structure used in optimal codebook search More... | |
typedef struct TrellisPath | TrellisPath |
Functions | |
static av_always_inline int | quant (float coef, const float Q) |
Quantize one coefficient. More... | |
static void | quantize_bands (int *out, const float *in, const float *scaled, int size, float Q34, int is_signed, int maxval) |
static void | abs_pow34_v (float *out, const float *in, const int size) |
static av_always_inline float | quantize_and_encode_band_cost_template (struct AACEncContext *s, PutBitContext *pb, const float *in, const float *scaled, int size, int scale_idx, int cb, const float lambda, const float uplim, int *bits, int BT_ZERO, int BT_UNSIGNED, int BT_PAIR, int BT_ESC) |
Calculate rate distortion cost for quantizing with given codebook. More... | |
static float | quantize_band_cost (struct AACEncContext *s, const float *in, const float *scaled, int size, int scale_idx, int cb, const float lambda, const float uplim, int *bits) |
static void | quantize_and_encode_band (struct AACEncContext *s, PutBitContext *pb, const float *in, int size, int scale_idx, int cb, const float lambda) |
static float | find_max_val (int group_len, int swb_size, const float *scaled) |
static int | find_min_book (float maxval, int sf) |
static void | encode_window_bands_info (AACEncContext *s, SingleChannelElement *sce, int win, int group_len, const float lambda) |
Encode band info for single window group bands. More... | |
static void | codebook_trellis_rate (AACEncContext *s, SingleChannelElement *sce, int win, int group_len, const float lambda) |
static av_always_inline uint8_t | coef2minsf (float coef) |
Return the minimum scalefactor where the quantized coef does not clip. More... | |
static av_always_inline uint8_t | coef2maxsf (float coef) |
Return the maximum scalefactor where the quantized coef is not zero. More... | |
static void | search_for_quantizers_anmr (AVCodecContext *avctx, AACEncContext *s, SingleChannelElement *sce, const float lambda) |
static void | search_for_quantizers_twoloop (AVCodecContext *avctx, AACEncContext *s, SingleChannelElement *sce, const float lambda) |
two-loop quantizers search taken from ISO 13818-7 Appendix C More... | |
static void | search_for_quantizers_faac (AVCodecContext *avctx, AACEncContext *s, SingleChannelElement *sce, const float lambda) |
static void | search_for_quantizers_fast (AVCodecContext *avctx, AACEncContext *s, SingleChannelElement *sce, const float lambda) |
static void | search_for_ms (AACEncContext *s, ChannelElement *cpe, const float lambda) |
Variables | |
static const uint8_t | run_value_bits_long [64] |
bits needed to code codebook run value for long windows More... | |
static const uint8_t | run_value_bits_short [16] |
bits needed to code codebook run value for short windows More... | |
static const uint8_t * | run_value_bits [2] |
static const uint8_t | aac_cb_range [12] = {0, 3, 3, 3, 3, 9, 9, 8, 8, 13, 13, 17} |
static const uint8_t | aac_cb_maxval [12] = {0, 1, 1, 2, 2, 4, 4, 7, 7, 12, 12, 16} |
static float(*const | quantize_and_encode_band_cost_arr [])(struct AACEncContext *s, PutBitContext *pb, const float *in, const float *scaled, int size, int scale_idx, int cb, const float lambda, const float uplim, int *bits) |
AACCoefficientsEncoder | ff_aac_coders [AAC_CODER_NB] |
Detailed Description
AAC coefficients encoder.
Definition in file aaccoder.c.
Macro Definition Documentation
#define quantize_and_encode_band_cost | ( | s, | |
pb, | |||
in, | |||
scaled, | |||
size, | |||
scale_idx, | |||
cb, | |||
lambda, | |||
uplim, | |||
bits | |||
) |
Definition at line 250 of file aaccoder.c.
Referenced by quantize_and_encode_band(), and quantize_band_cost().
#define QUANTIZE_AND_ENCODE_BAND_COST_FUNC | ( | NAME, | |
BT_ZERO, | |||
BT_UNSIGNED, | |||
BT_PAIR, | |||
BT_ESC | |||
) |
Definition at line 210 of file aaccoder.c.
#define TRELLIS_STAGES 121 |
Definition at line 551 of file aaccoder.c.
Referenced by search_for_quantizers_anmr().
#define TRELLIS_STATES (SCALE_MAX_DIFF+1) |
Definition at line 552 of file aaccoder.c.
Referenced by search_for_quantizers_anmr().
Typedef Documentation
typedef struct BandCodingPath BandCodingPath |
structure used in optimal codebook search
typedef struct TrellisPath TrellisPath |
Function Documentation
|
static |
Definition at line 86 of file aaccoder.c.
Referenced by codebook_trellis_rate(), encode_window_bands_info(), quantize_and_encode_band_cost_template(), search_for_ms(), search_for_quantizers_anmr(), search_for_quantizers_faac(), and search_for_quantizers_twoloop().
|
static |
Definition at line 413 of file aaccoder.c.
|
static |
Return the maximum scalefactor where the quantized coef is not zero.
Definition at line 542 of file aaccoder.c.
Referenced by search_for_quantizers_anmr().
|
static |
Return the minimum scalefactor where the quantized coef does not clip.
Definition at line 537 of file aaccoder.c.
Referenced by search_for_quantizers_anmr().
|
static |
Encode band info for single window group bands.
Definition at line 312 of file aaccoder.c.
|
static |
Definition at line 274 of file aaccoder.c.
Referenced by search_for_quantizers_anmr(), and search_for_quantizers_twoloop().
|
static |
Definition at line 285 of file aaccoder.c.
Referenced by search_for_quantizers_anmr(), and search_for_quantizers_twoloop().
|
static |
Quantize one coefficient.
- Returns
- absolute value of the quantized coefficient
- See also
- 3GPP TS26.403 5.6.2 "Scalefactor determination"
Definition at line 66 of file aaccoder.c.
Referenced by quantize_and_encode_band_cost_template(), and search_for_quantizers_faac().
|
static |
Definition at line 266 of file aaccoder.c.
|
static |
Calculate rate distortion cost for quantizing with given codebook.
- Returns
- quantization distortion
Definition at line 105 of file aaccoder.c.
|
static |
Definition at line 257 of file aaccoder.c.
Referenced by codebook_trellis_rate(), encode_window_bands_info(), search_for_ms(), search_for_quantizers_anmr(), search_for_quantizers_faac(), and search_for_quantizers_twoloop().
|
static |
Definition at line 72 of file aaccoder.c.
Referenced by quantize_and_encode_band_cost_template().
|
static |
Definition at line 1054 of file aaccoder.c.
|
static |
Definition at line 554 of file aaccoder.c.
|
static |
Definition at line 852 of file aaccoder.c.
|
static |
Definition at line 1020 of file aaccoder.c.
|
static |
two-loop quantizers search taken from ISO 13818-7 Appendix C
Definition at line 707 of file aaccoder.c.
Variable Documentation
|
static |
Definition at line 98 of file aaccoder.c.
Referenced by quantize_and_encode_band_cost_template().
|
static |
Definition at line 97 of file aaccoder.c.
Referenced by quantize_and_encode_band_cost_template().
AACCoefficientsEncoder ff_aac_coders[AAC_CODER_NB] |
Definition at line 1115 of file aaccoder.c.
Referenced by aac_encode_init().
|
static |
Definition at line 230 of file aaccoder.c.
|
static |
Definition at line 56 of file aaccoder.c.
Referenced by codebook_trellis_rate(), and encode_window_bands_info().
|
static |
bits needed to code codebook run value for long windows
Definition at line 44 of file aaccoder.c.
|
static |
bits needed to code codebook run value for short windows
Definition at line 52 of file aaccoder.c.
Generated on Sun May 11 2025 06:53:49 for FFmpeg by
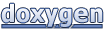