qm-dsp
1.8
|
Resampler resamples a stream from one integer sample rate to another (arbitrary) rate, using a kaiser-windowed sinc filter. More...
#include <Resampler.h>
Classes | |
struct | Phase |
Public Member Functions | |
Resampler (int sourceRate, int targetRate) | |
Construct a Resampler to resample from sourceRate to targetRate. More... | |
Resampler (int sourceRate, int targetRate, double snr, double bandwidth) | |
Construct a Resampler to resample from sourceRate to targetRate, using the given filter parameters. More... | |
virtual | ~Resampler () |
int | process (const double *src, double *dst, int n) |
Read n input samples from src and write resampled data to dst. More... | |
std::vector< double > | process (const double *src, int n) |
Read n input samples from src and return resampled data by value. More... | |
int | getLatency () const |
Return the number of samples of latency at the output due by the filter. More... | |
Static Public Member Functions | |
static std::vector< double > | resample (int sourceRate, int targetRate, const double *data, int n) |
Carry out a one-off resample of a single block of n samples. More... | |
Private Member Functions | |
void | initialise (double, double) |
double | reconstructOne () |
Private Attributes | |
int | m_sourceRate |
int | m_targetRate |
int | m_gcd |
int | m_filterLength |
int | m_latency |
double | m_peakToPole |
Phase * | m_phaseData |
int | m_phase |
std::vector< double > | m_buffer |
int | m_bufferOrigin |
Detailed Description
Resampler resamples a stream from one integer sample rate to another (arbitrary) rate, using a kaiser-windowed sinc filter.
The results and performance are pretty similar to libraries such as libsamplerate, though this implementation does not support time-varying ratios (the ratio is fixed on construction).
See also Decimator, which is faster and rougher but supports only power-of-two downsampling factors.
Definition at line 30 of file Resampler.h.
Constructor & Destructor Documentation
Resampler::Resampler | ( | int | sourceRate, |
int | targetRate | ||
) |
Construct a Resampler to resample from sourceRate to targetRate.
Definition at line 36 of file Resampler.cpp.
References initialise().
Resampler::Resampler | ( | int | sourceRate, |
int | targetRate, | ||
double | snr, | ||
double | bandwidth | ||
) |
Construct a Resampler to resample from sourceRate to targetRate, using the given filter parameters.
Definition at line 46 of file Resampler.cpp.
References initialise().
|
virtual |
Definition at line 54 of file Resampler.cpp.
References m_phaseData.
Member Function Documentation
int Resampler::process | ( | const double * | src, |
double * | dst, | ||
int | n | ||
) |
Read n input samples from src and write resampled data to dst.
The return value is the number of samples written, which will be no more than ceil((n * targetRate) / sourceRate). The caller must ensure the dst buffer has enough space for the samples returned.
Definition at line 303 of file Resampler.cpp.
References Resampler::Phase::filter, m_buffer, m_bufferOrigin, m_gcd, m_peakToPole, m_phase, m_phaseData, m_sourceRate, m_targetRate, and reconstructOne().
Referenced by process(), and resample().
vector< double > Resampler::process | ( | const double * | src, |
int | n | ||
) |
Read n input samples from src and return resampled data by value.
Definition at line 335 of file Resampler.cpp.
References m_sourceRate, m_targetRate, and process().
|
inline |
Return the number of samples of latency at the output due by the filter.
(That is, the output will be delayed by this number of samples relative to the input.)
Definition at line 68 of file Resampler.h.
References m_latency, and resample().
Referenced by resample().
|
static |
Carry out a one-off resample of a single block of n samples.
The output is latency-compensated.
Definition at line 346 of file Resampler.cpp.
References getLatency(), and process().
Referenced by getLatency().
|
private |
Definition at line 60 of file Resampler.cpp.
References SincWindow::cut(), KaiserWindow::cut(), Resampler::Phase::drop, Resampler::Phase::filter, MathUtilities::gcd(), KaiserWindow::Parameters::length, m_buffer, m_bufferOrigin, m_filterLength, m_gcd, m_latency, m_peakToPole, m_phase, m_phaseData, m_sourceRate, m_targetRate, Resampler::Phase::nextPhase, and KaiserWindow::parametersForBandwidth().
Referenced by Resampler().
|
private |
Definition at line 277 of file Resampler.cpp.
References Resampler::Phase::drop, Resampler::Phase::filter, m_buffer, m_bufferOrigin, m_phase, m_phaseData, Resampler::Phase::nextPhase, and QM_R__.
Referenced by process().
Member Data Documentation
|
private |
Definition at line 78 of file Resampler.h.
Referenced by initialise(), and process().
|
private |
Definition at line 79 of file Resampler.h.
Referenced by initialise(), and process().
|
private |
Definition at line 80 of file Resampler.h.
Referenced by initialise(), and process().
|
private |
Definition at line 81 of file Resampler.h.
Referenced by initialise().
|
private |
Definition at line 82 of file Resampler.h.
Referenced by getLatency(), and initialise().
|
private |
Definition at line 83 of file Resampler.h.
Referenced by initialise(), and process().
|
private |
Definition at line 91 of file Resampler.h.
Referenced by initialise(), process(), reconstructOne(), and ~Resampler().
|
private |
Definition at line 92 of file Resampler.h.
Referenced by initialise(), process(), and reconstructOne().
|
private |
Definition at line 93 of file Resampler.h.
Referenced by initialise(), process(), and reconstructOne().
|
private |
Definition at line 94 of file Resampler.h.
Referenced by initialise(), process(), and reconstructOne().
The documentation for this class was generated from the following files:
Generated by
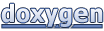